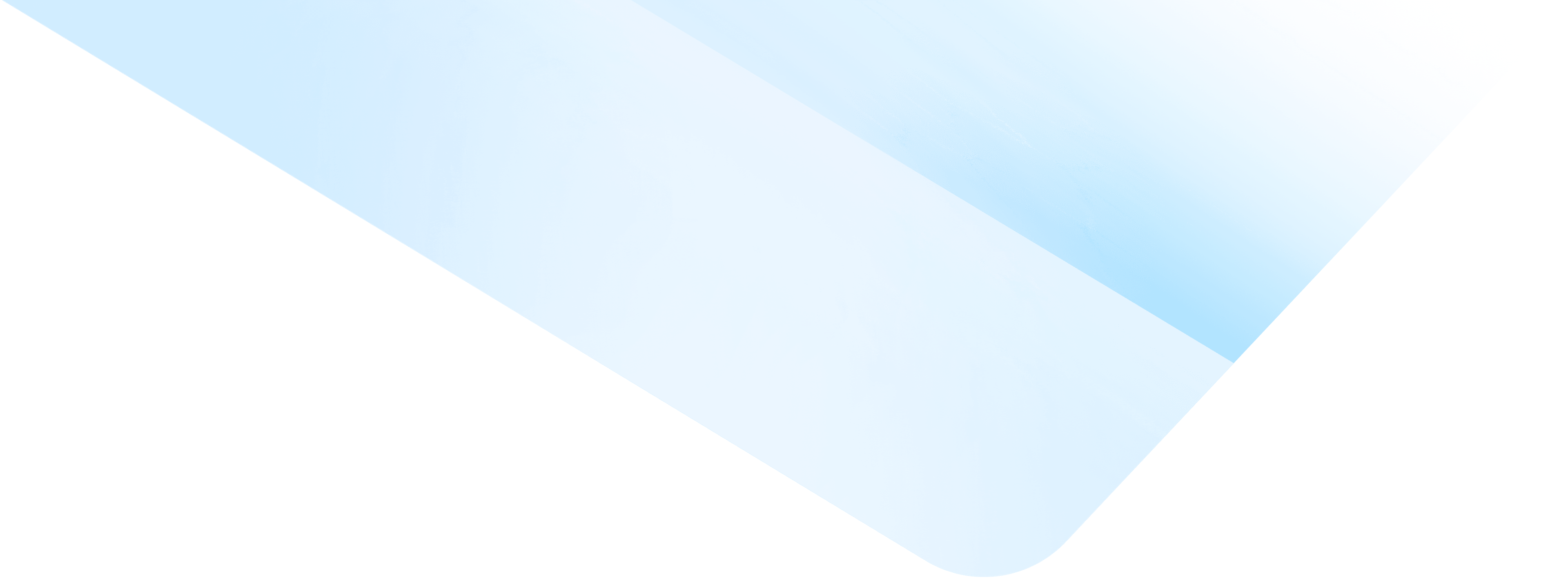
Developer Tools
A few lines of code to create a seamless NFT experience
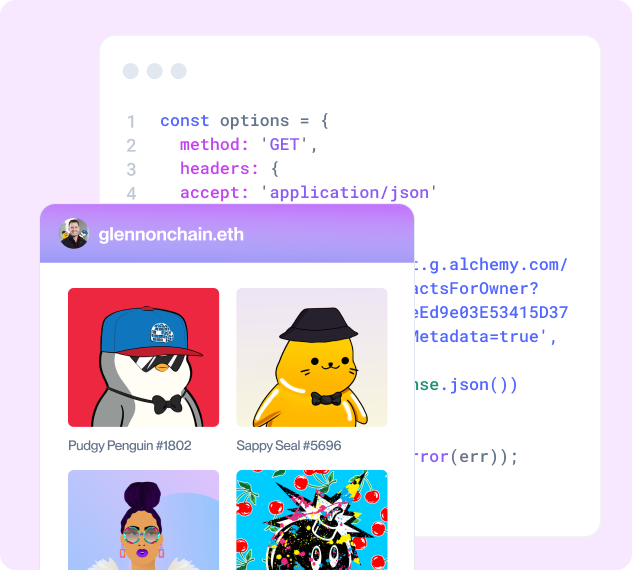
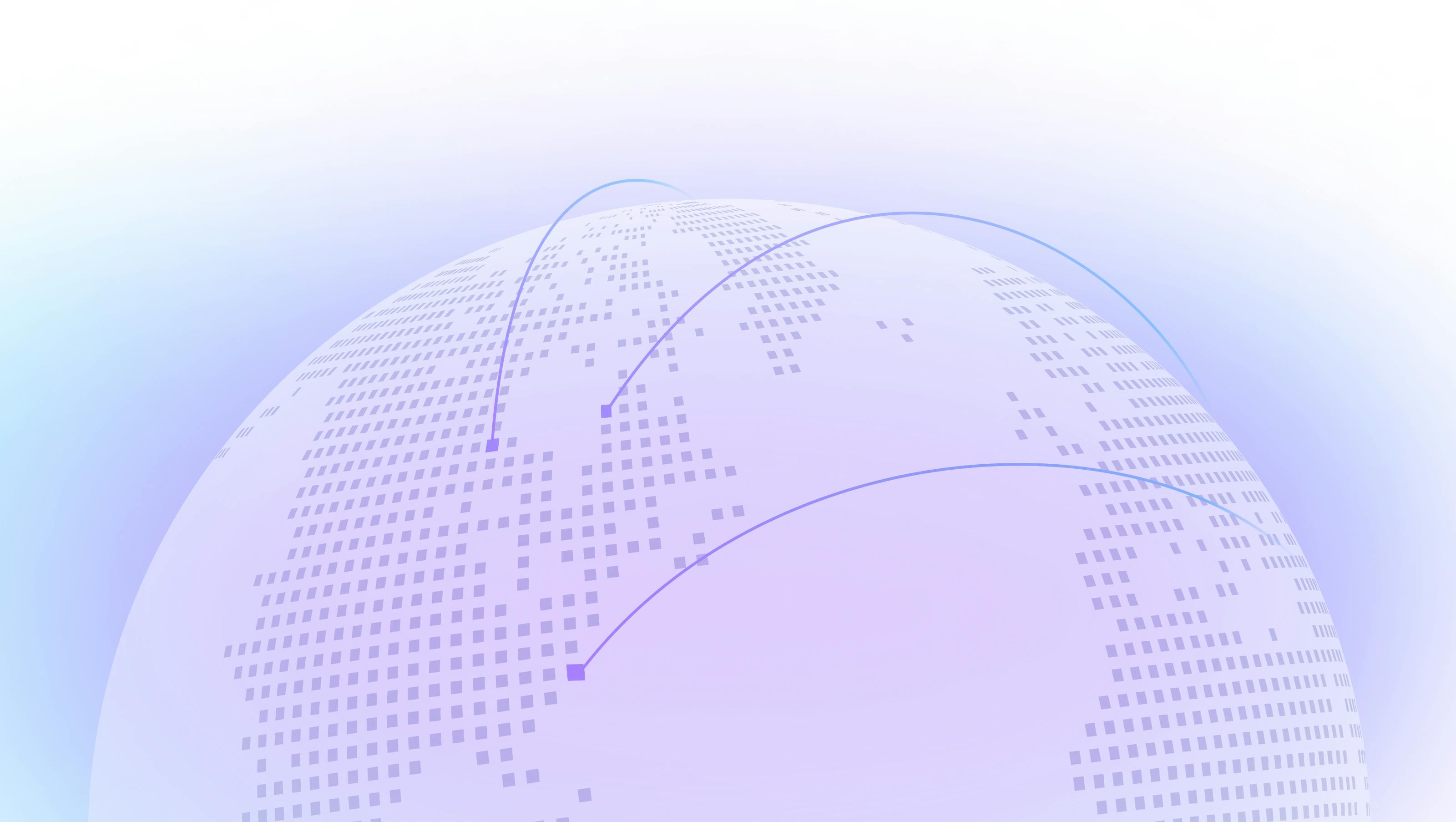
Save money. Ship faster.
2.7x more
NFTs than alternatives17x faster
than alternatives4x better
spam detection than alternatives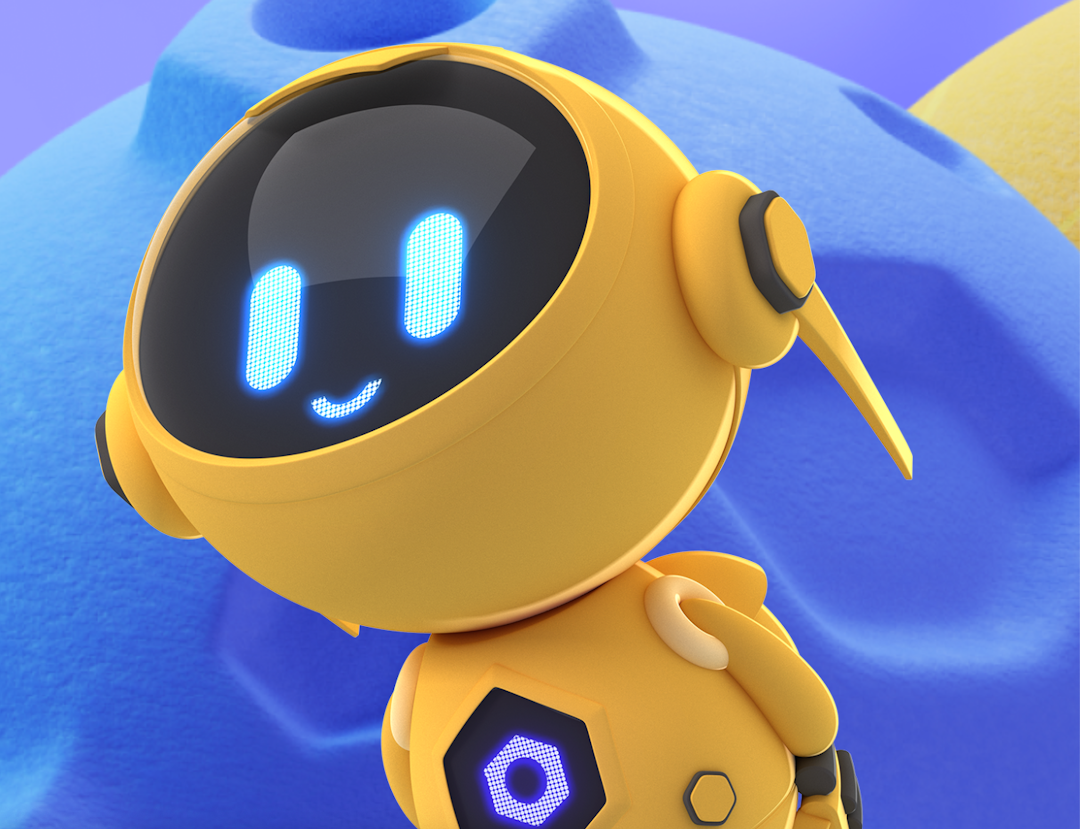
Blockchain
Infrastructure that’s both reliable and scalable, so that we can stay up when our customers need us most - that’s huge for Collab.Land. Alchemy is the GOAT here.
Former Head of Protocol | Kartik Patel
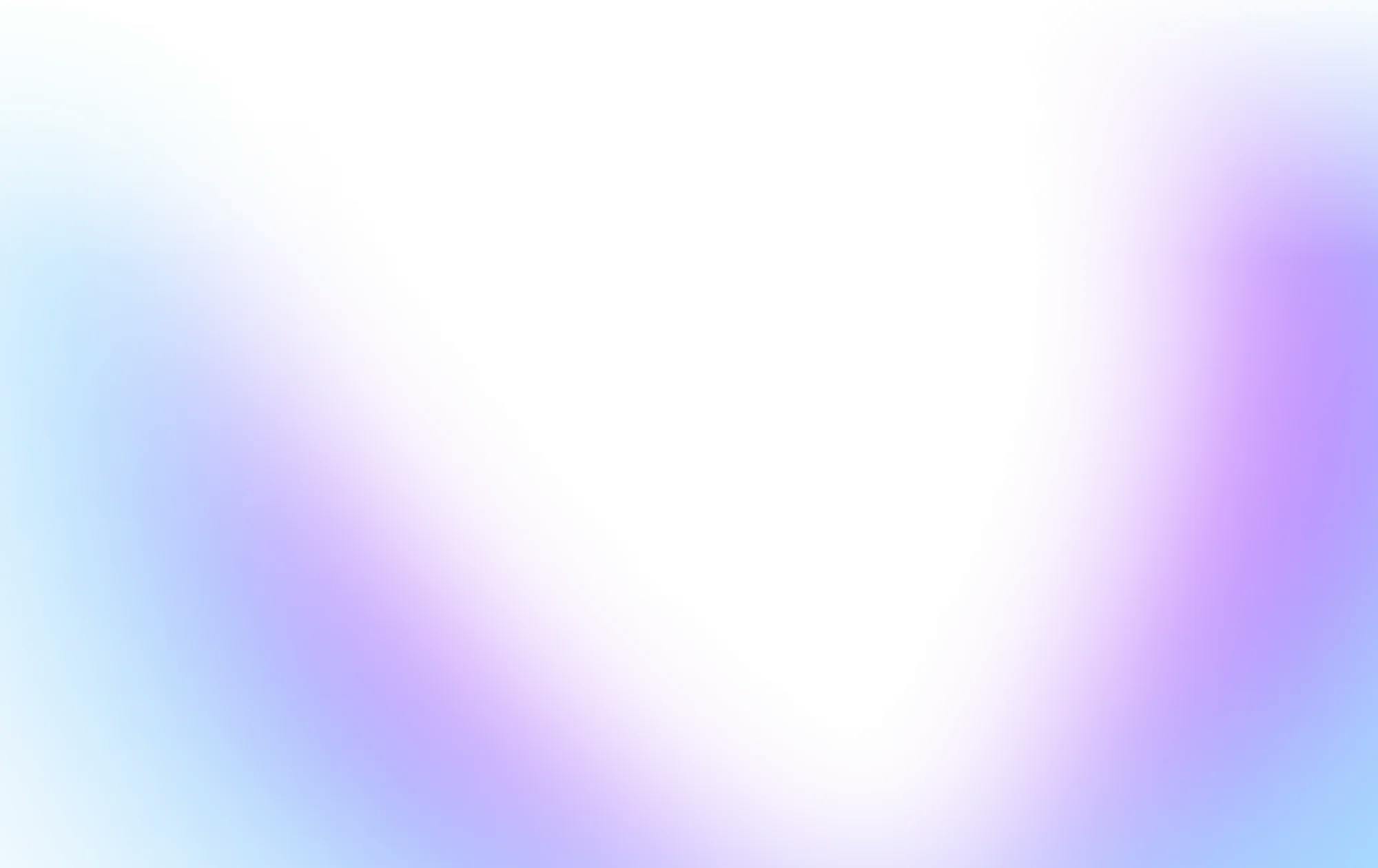
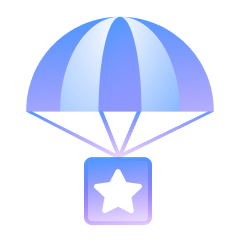
Power your NFT airdrops and create a seamless experience for your users.
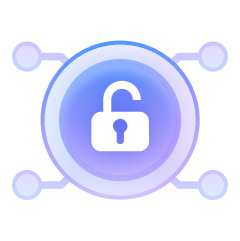
Create token gated experiences to enable exclusivity and NFT membership.
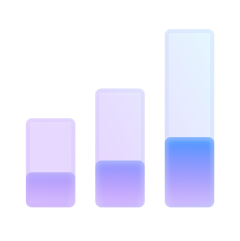
View and access all relevant NFT data onchain.
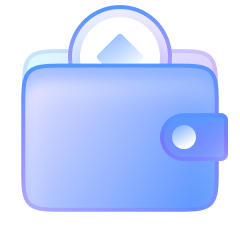
Get the right data to display the correct NFTs for the appropriate owners for your wallet app.
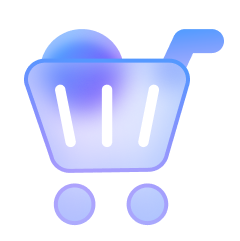
Create the next great NFT marketplace and let Alchemy handle the infrastructure.
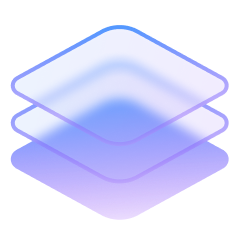
Interact with NFTs without delving deep into the complexities of smart contracts, blockchain operations, or various blockchain protocols.
"Alchemy’s NFT API will allow us to be the token verification system for every web3 community in the world - so we can quickly, accurately and reliably query NFT metadata, and our customers can create meaningful shared experiences for their users."
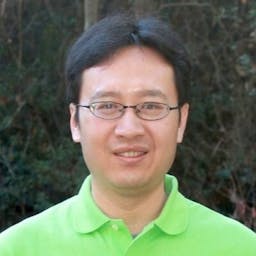
Raymond Feng
CTO and Co-founder, Collab.Land
Explore more of our developer suite
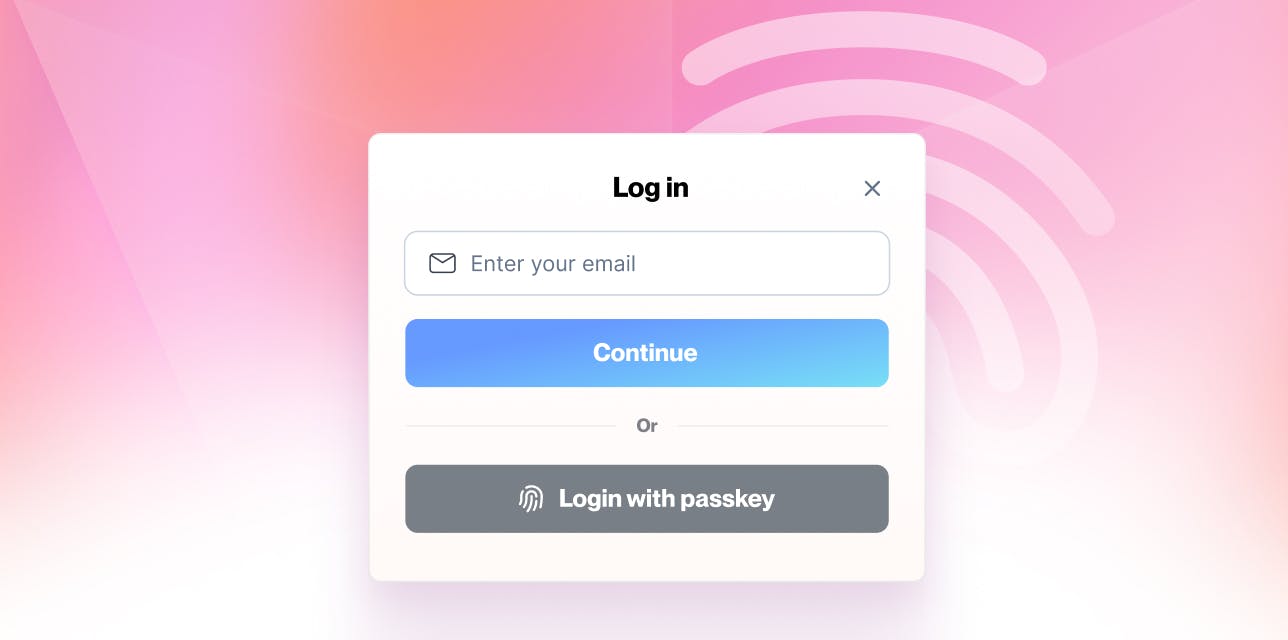
Plug-n-play embedded wallets
Make wallets invisible. Simple, non-custodial accounts to onboard users and transact with web2 UX.
Code preview
Copied
// 1. Auth Your User
const signer = new AlchemySigner({
client: {
connection: { rpcUrl },
iframeConfig: { iframeContainerId: "alchemy-signer-iframe-container" },
},
})
signer.authenticate({ type: "email", email: "[email protected]", bundle });
// 2. Create an Account
const account = await createMultiOwnerModularAccount({
transport,
chain,
signer,
});
// 3. Use web3!
account.signMessage({ message: "Hello, World!" });
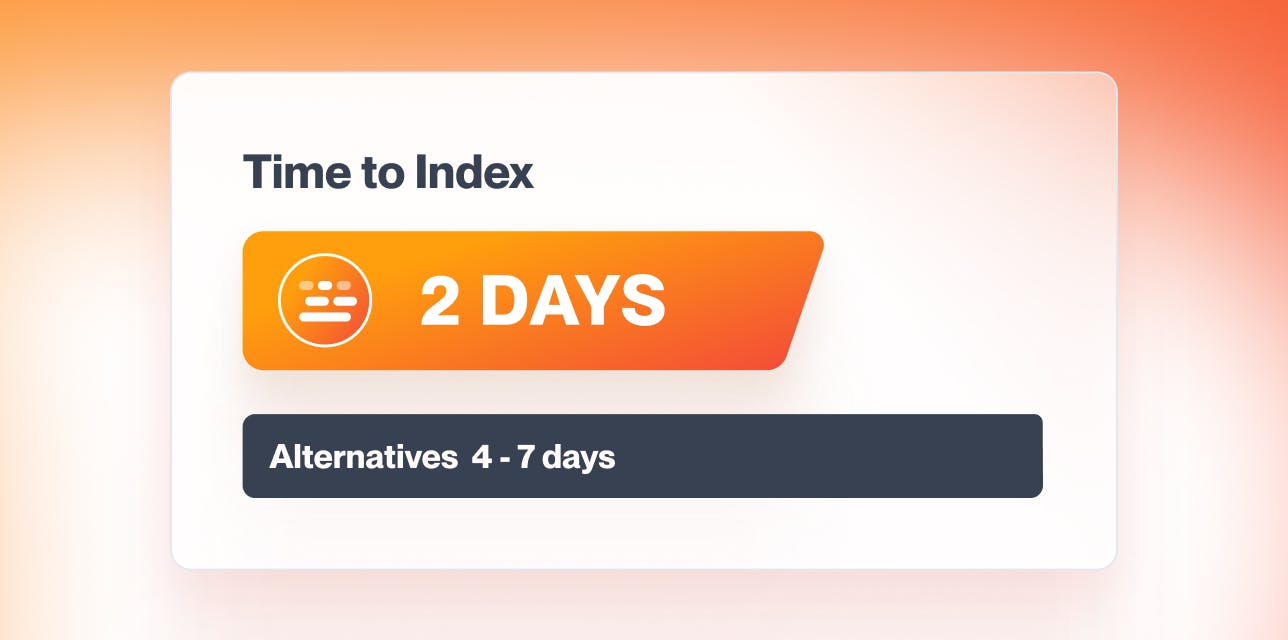
Speedy indexing for custom GraphQL APIs
Ship faster with a custom API for your onchain data. Never worry about subgraph downtime or lag again.
Code preview
Copied
cd <SUBGRAPH_DIRECTORY>
graph deploy <SUBGRAPH_NAME> \
--version-label <VERSION_NAME> \
--node https://subgraphs.alchemy.com/api/subgraphs/deploy \
--deploy-key <DEPLOY_KEY>
--ipfs https://ipfs.satsuma.xyz
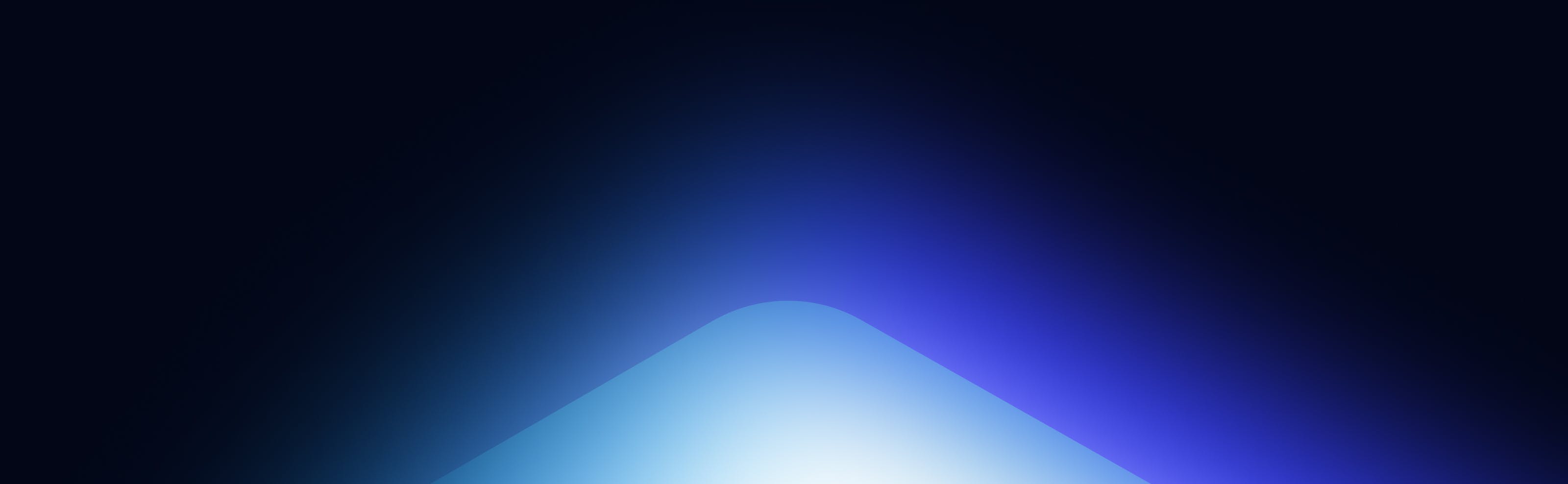