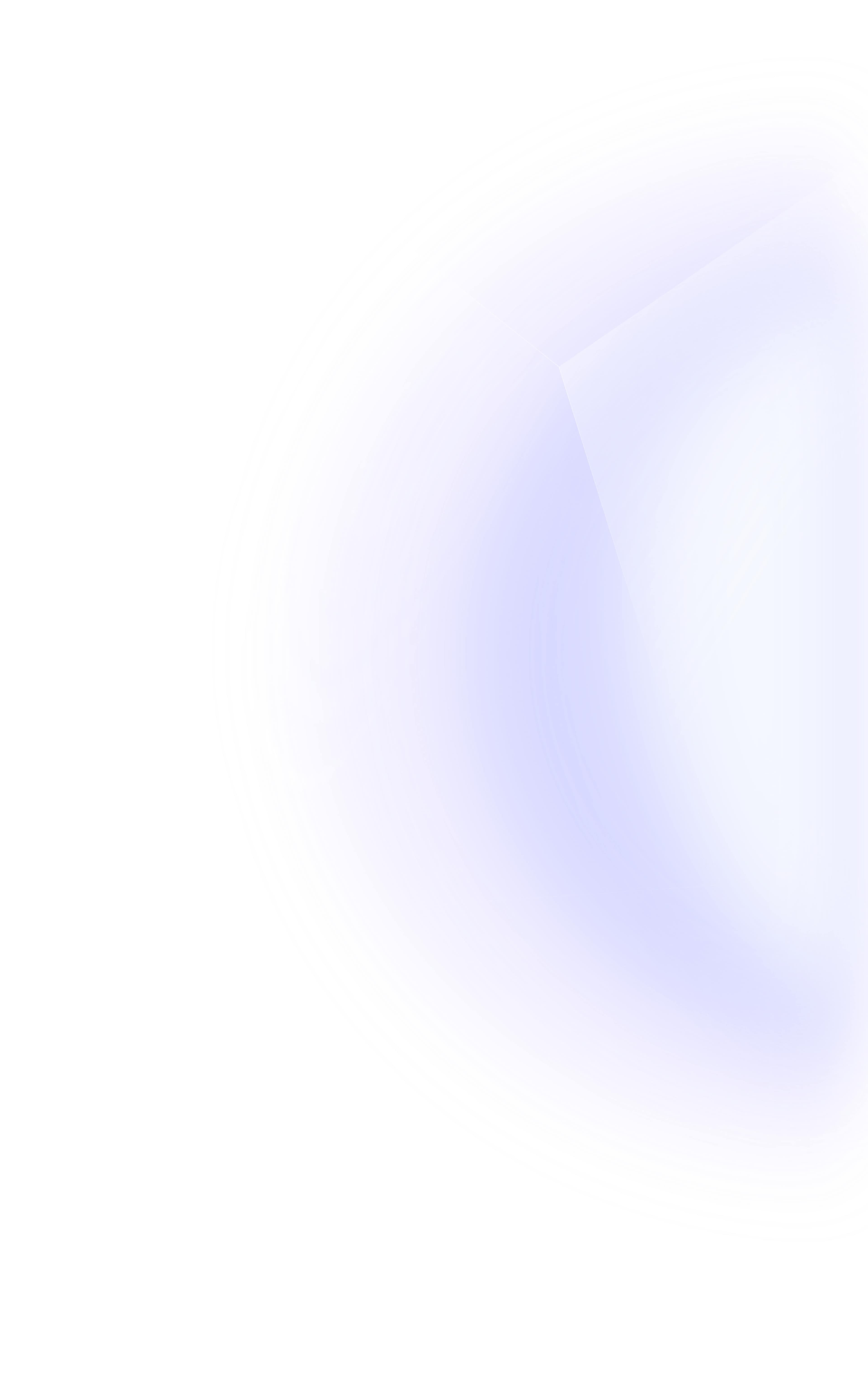
The Complete Guide to ERC-20 Tokens and Solidity (2024)
Written by Turja

Reviewed by Brady Werkheiser
The Ethereum ecosystem contains a wide variety of standards that represent the functionality of smart contracts from creation to deployment. The most common standards are ERC-20, ERC-721, ERC-777, and ERC-1155 with each serving its own main objective.
This article defines ERC-20 tokens, gives some of their uses, and outlines some of the mandatory functions in the Solidity programming language developers need to use to stay in line with the standard. If you want to create your own ERC-20 token using Solidity, the guidelines and definitions within this article will give you a better understanding of how to do that.
What is an ERC-20 token?
The ERC-20 is a popular fungible cryptocurrency token compatible with Ethereum or EVM-compatible blockchains. It serves as a digital asset that can represent anything on the blockchain, which enables flexibility for many use cases.
This preset smart contract contains a specific interface that allows it to be used as a technical standard for development. Developers can leverage the ERC-20 token and its associated token rules for an easier developer experience. Popular decentralized exchange protocols like Uniswap and SushiSwap both leverage the ERC-20 token standard as building blocks for its seamless compatibility and integration into their ecosystems.
How are ERC-20 tokens used?
As the ERC-20 token has no restrictions on what it can represent, it can expand further beyond a typical cryptocurrency (i.e. ETH). As a requirement, ERC-20 assets are fungible, transferable, and can be limited to a max supply. This is useful when creating rewards, physical objects, shares of a company, and much more.
Designed to standardize the development of tokens, the ERC-20 token can represent any fungible asset on the Ethereum blockchain. By providing a few variables to represent a particular asset (e.g. name, symbol and supply) anyone can launch an ERC20 token with the standard behavior and interface.
ERC-20 tokens are fungible as each token is exactly equal to any other token. With no special rights or behavior associated with any individual token, this equality of value among ERC-20 tokens makes them useful in such applications as a medium of exchange, currency, voting rights, and staking.
Why is the ERC-20 token standard important?
The ERC-20 token standard introduces a specific approach for fungible tokens, ensuring that they all share similar properties.
Since its proposal in 2015, the development of the ERC-20 token standard allows for other protocols, platforms, and developers to create smart contracts that can use any token following the standard without creating special logic for each new token. The standardization for smart contracts enhances the development process and in turn, greatly benefits the entire crypto ecosystem.
What Solidity functions are mandatory for all ERC-20 tokens?
The ERC-20 standard contains a set of methods and events that must be present in every implementation of the standard, including methods to transfer value, lookup balances for addresses and retrieve other metadata.
An understanding of the distinctions of the Solidity language and a practical knowledge of how to use functions to create ERC-20 tokens on Ethereum can accelerate your journey to become a Solidity developer.
1. totalSupply
The totalSupply method denotes the current circulating total supply of the tokens. Here is an example:
function totalSupply() external view returns (uint256);
2. balanceOf
The balanceOf method calculates the number of tokens contained in a particular wallet address. Here is an example:
function balanceOf(address account) external view returns (uint256);
3. transfer
The transfer method sends tokens from one address to another, where the sender is the origin of the transaction. Here is an example:
function transfer(address recipient, uint256 amount) external returns (bool);
4. approve
The approve method allows another address to spend tokens on your behalf. This is useful when interacting with smart contracts that need access to your tokens. A popular use case for the approve method is a decentralized exchange. Here is an example:
function approve(address spender, uint256 amount) external returns (bool);
5. transferFrom
The transferFrom method is useful together with the approve method. Once you approve someone to spend your tokens, they can transferFrom your account to another account as part of a transaction. A good use case is a decentralized exchange. Here is an example:
function transferFrom(address sender, address recipient, uint256 amount) external returns (bool);
6. allowance
The allowance method is the amount one address can spend on behalf of another address. Here is an example:
function allowance(address owner, address spender) external view returns (uint256);
What Solidity functions are optional for all ERC-20 tokens?
Names, symbols, and decimals are all optional functions serving as an extension to the base interface of the ERC-20 token contract. Although these functions are not required, they provide additional details to the contract beneficial to understanding its objective. This core interface can serve as the base for additional customization and extensions to the smart contract.
1. Name
The name is a human-readable name that defines the purpose of the contract. Here is an example:
string public constant name = "ERC20Basic";
2. Symbol
The symbol is a human-readable ticker of the token that can be used to represent it. Similar to ETH, BTC, etc. Here is an example:
string public constant symbol = "ERC";
3. Decimals
Decimals define the denomination of the smallest unit of the currency. It is most commonly 18, which is the same denomination for ether (the smallest unit of ether is wei: one ether is 1e18 wei).
uint8 public constant decimals = 18;balances (owner_address => balance_amount)
What are ERC-20 data structures?
ERC-20 data structures like tables of balances and allowances facilitate the organization and implementation of operations on the blockchain.
Balances
An internal table of token balances is used to track total ownership by a wallet address. Each transfer is a deduction from one balance and an addition to another balance.
mapping(address => uint256) balances;
Allowances
An internal table of token allowances are used to track delegated spending by a wallet address. Using nested mapping, the primary key is the address of the token owner which maps to a spender address and provides the delegated amount to spend.
function allowance(address owner, address spender) external view returns (uint256);
Start Building with Alchemy
The ERC-20 standard is the most important standard to emerge from the Ethereum ecosystem as it is widely adopted and used across the most important smart contract protocols. As a developer, it is highly recommended you spend time getting to know this standard and deploy your own ERC-20 token.
To learn about ERC-20 tokens and other Solidity programming fundamentals, secure your spot in Alchemy University's Ethereum Developer Bootcamp.
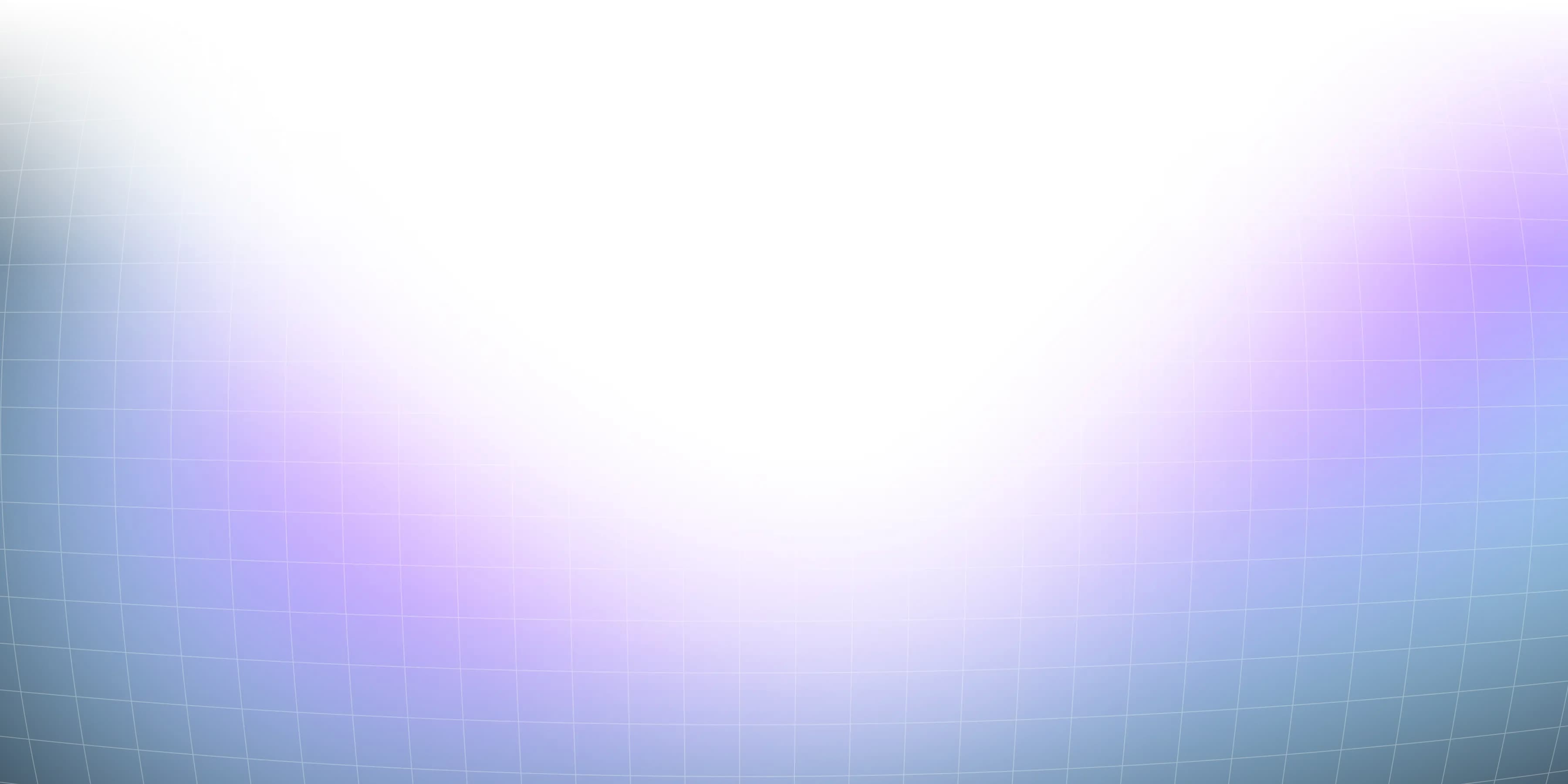
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
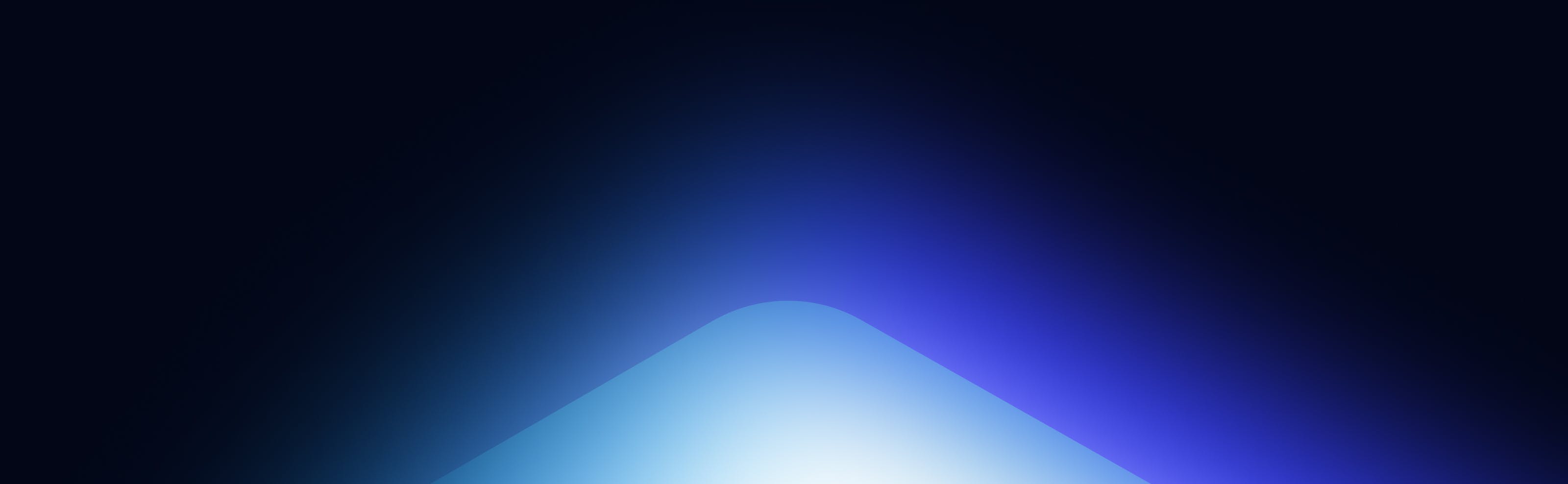