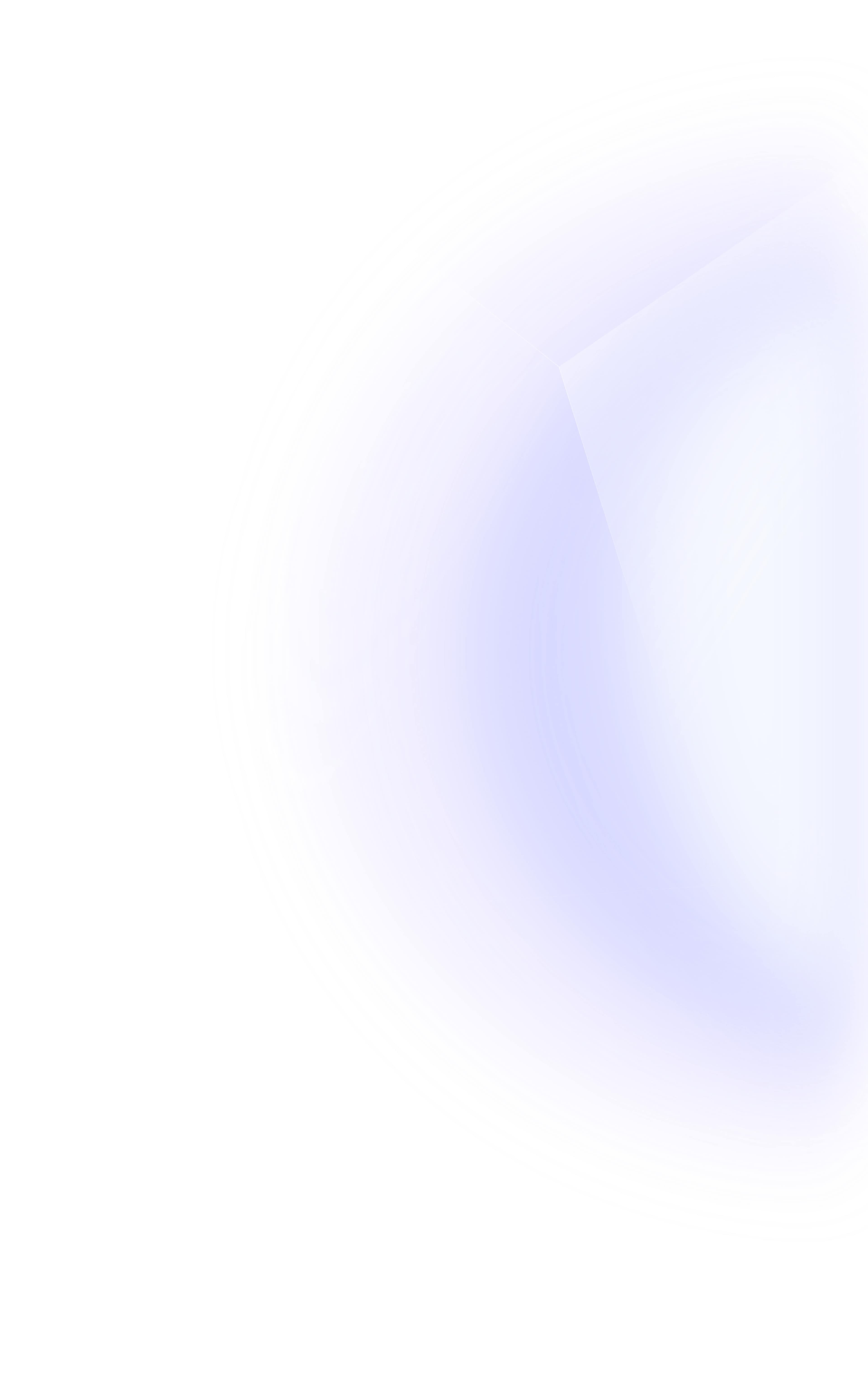
What is the Solidity contract interface?

Written by Alchemy
Solidity is an object-oriented, high-level language for implementing smart contracts. While writing smart contracts in Solidity, you may want to use interfaces to interact with other smart contracts. Knowing how to use interfaces will help you increase your knowledge of the Solidity language and may give you some new ideas on other interesting Solidity smart contracts you can build.
In this article, we explain what a Solidity contract interface is and show you how to create one. We provide some examples for interface implementation and declaration to serve as guides as you write one.
What is the Solidity interface?
A Solidity contract interface is a list of function definitions without implementation. In other words, an interface is a description of all functions that an object must have for it to operate. The interface enforces a defined set of properties and functions on a contract.
Solidity allows you to interact with other contracts without having their code by using their interface. For example, if you want to interact with another contract from your own contract, you provide your calls with an interface wrapper. By declaring an interface, you can interact with other contracts, and call functions in another contract.
Interfaces are usually found at the top of a Solidity contract, and they are identified using the “interface” keyword. Because interfaces reduce code duplication and overhead, they are most useful when decentralized applications require extensibility and want to avoid complexity.
Solidity Interface Characteristics
The Solidity interface can inherit from other interfaces
Contracts can inherit interfaces as they would inherit other contracts
You can override an interface function
Data types defined inside interfaces can be accessed from other contracts
All functions that inherit from the interface must set the override modifier on every function that overrides an interface function. Otherwise, the Solidity compiler will throw an error.
Abstract Contracts vs. Interfaces
Abstract contracts and interfaces are two ways web3 developers can build larger, more complex distributed applications because they allow for extensibility within Solidity.
Abstract contracts possess at least one function that lacks implementation, and as a result, they cannot be compiled. However, abstract contracts can be used as base contracts from which other contracts can inherit.
Interfaces are similar to abstract contracts, but they cannot have any functions implemented. Additionally, interfaces are limited to what the contract’s Application Binary Interface (ABI) can represent. The conversion between the ABI and an interface is possible without any information loss.
How to Create a Solidity Interface
Interfaces are usually at the top of your program and declared with the “interface” keyword. Then you can use that interface to communicate with another contract, or you can implement the interface.
Suppose you were writing a smart contract wallet, it might look something like this:
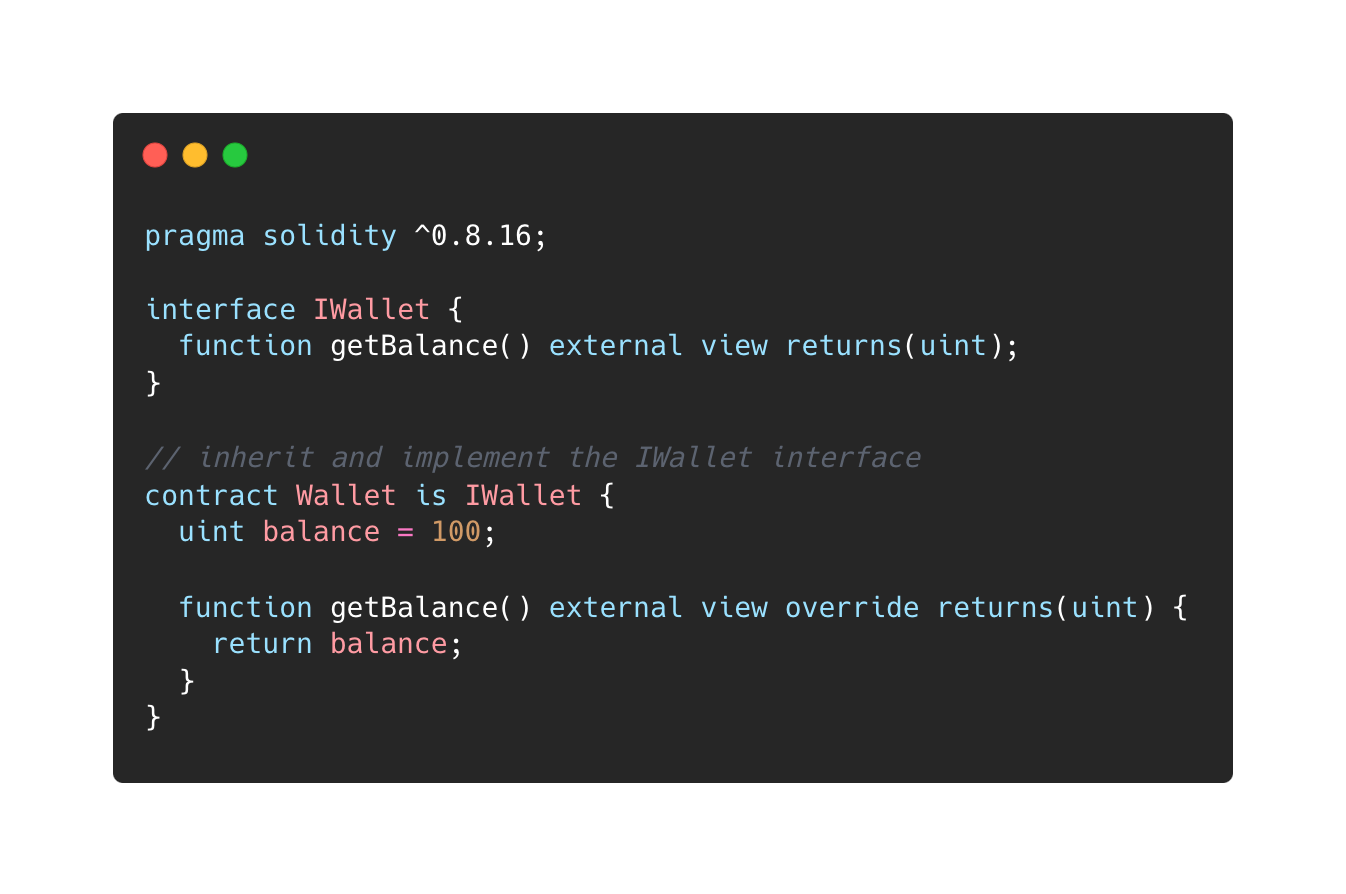
Notice the use of “is IWallet
” here. In this case we are inheriting the IWallet
interface and implementing it in our contract, Wallet
.
Then, if you had some smart contract that wanted to be able to communicate with wallets (WalletFriendlyContract
here), you could re-use the interface:
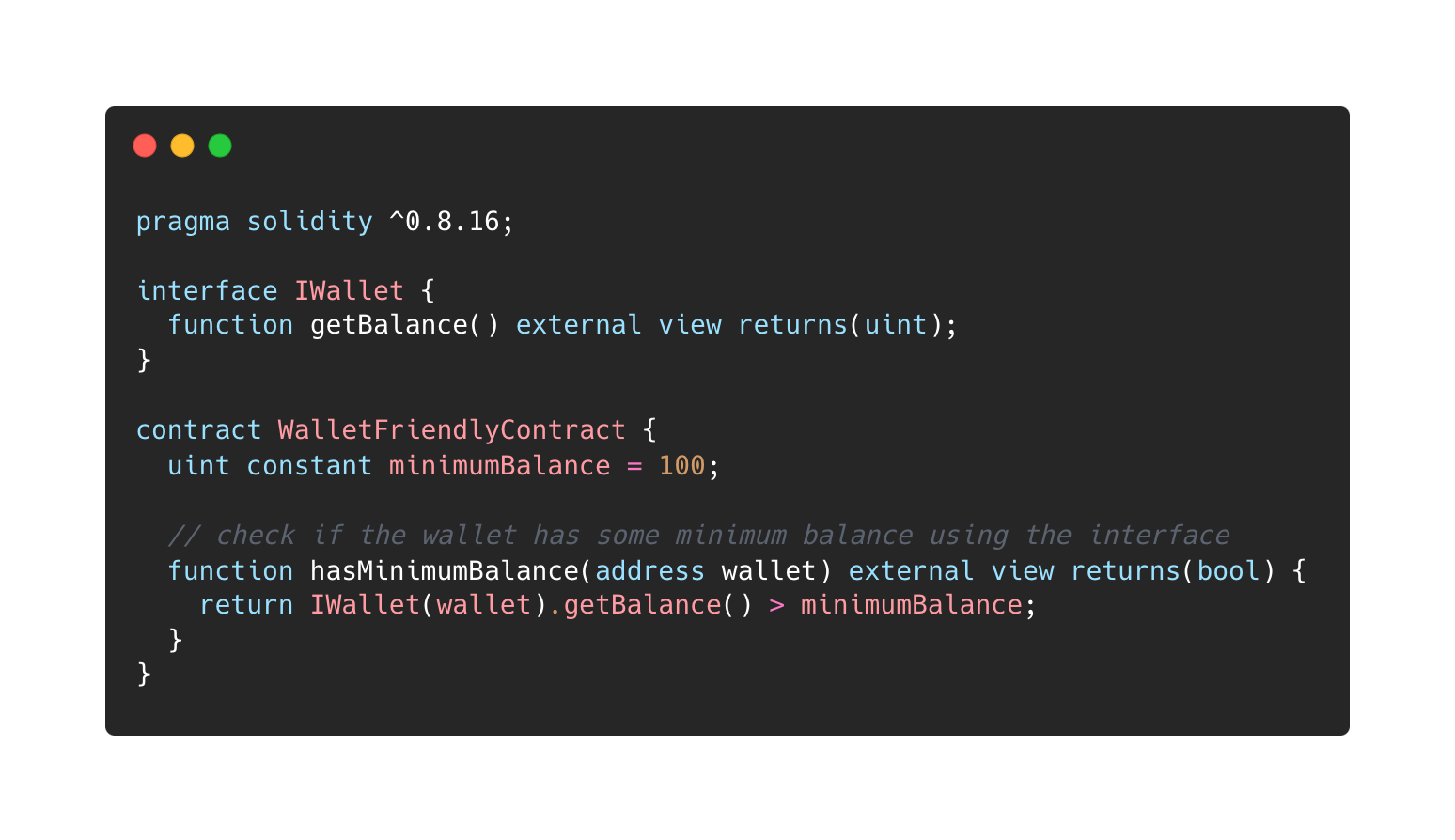
Solidity Interface Requirements
There are some restrictions when creating interfaces in Solidity, and developers should remember this list of the main interface requirements:
The interface cannot have any functions implemented
Functions of an interface can be only of type external
The interface cannot declare a constructor
The interface cannot declare state variables
Solidity Interface Examples
This section contains some examples to guide you in writing Solidity interface code.
The following example interface code, taken from the Solidity documentation, creates an interface named “Token” for retrieving information about transactions. It contains a function to access information about the address recipient and the amount transferred from other contracts.
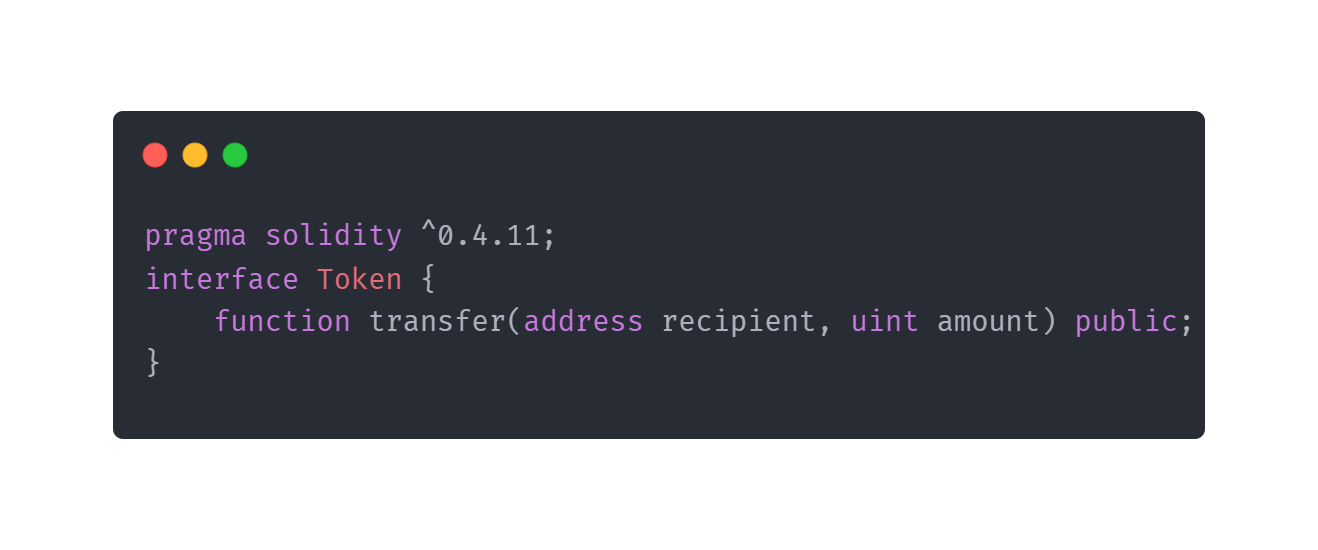
The next Solidity interface example, taken from Solidity by Example, shows a full contract with interface declaration and implementation.
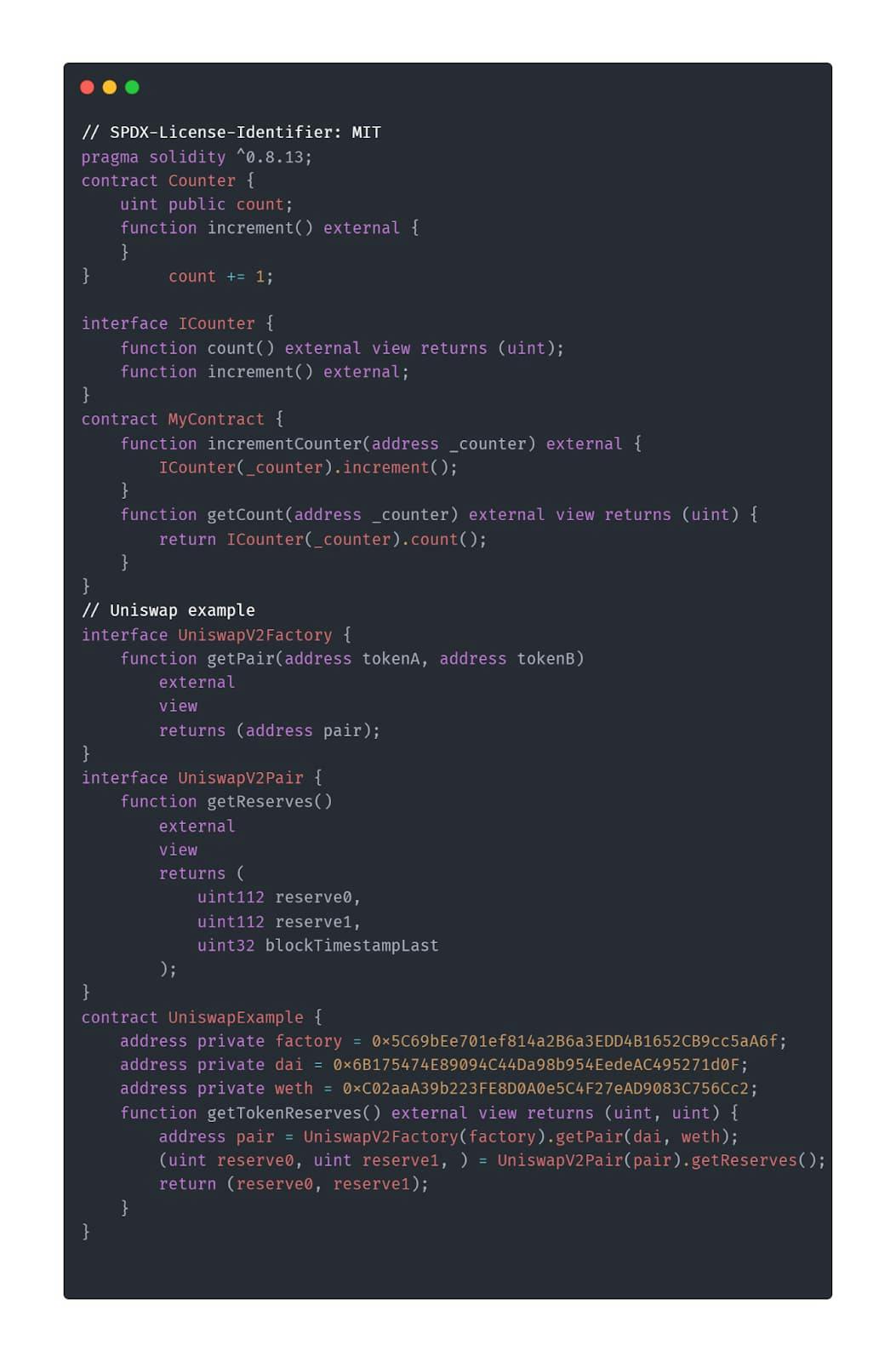
Start Building Solidity Apps with Alchemy
This article introduced you to Solidity interfaces and demonstrated how developers can use interfaces to save time and reduce complexity when building applications in Solidity. To continue learning about Solidity, explore Alchemy University's free Ethereum Developer Bootcamp, that explains the core concepts of Solidity development over a 7-week, self-paced course packed with coding challenges, video lessons, and the best resources for mastering Solidity.
If developers are new to development in general, Alchemy University's 3-week JavaScript crash course is a great prerequisite before starting an Ethereum bootcamp.
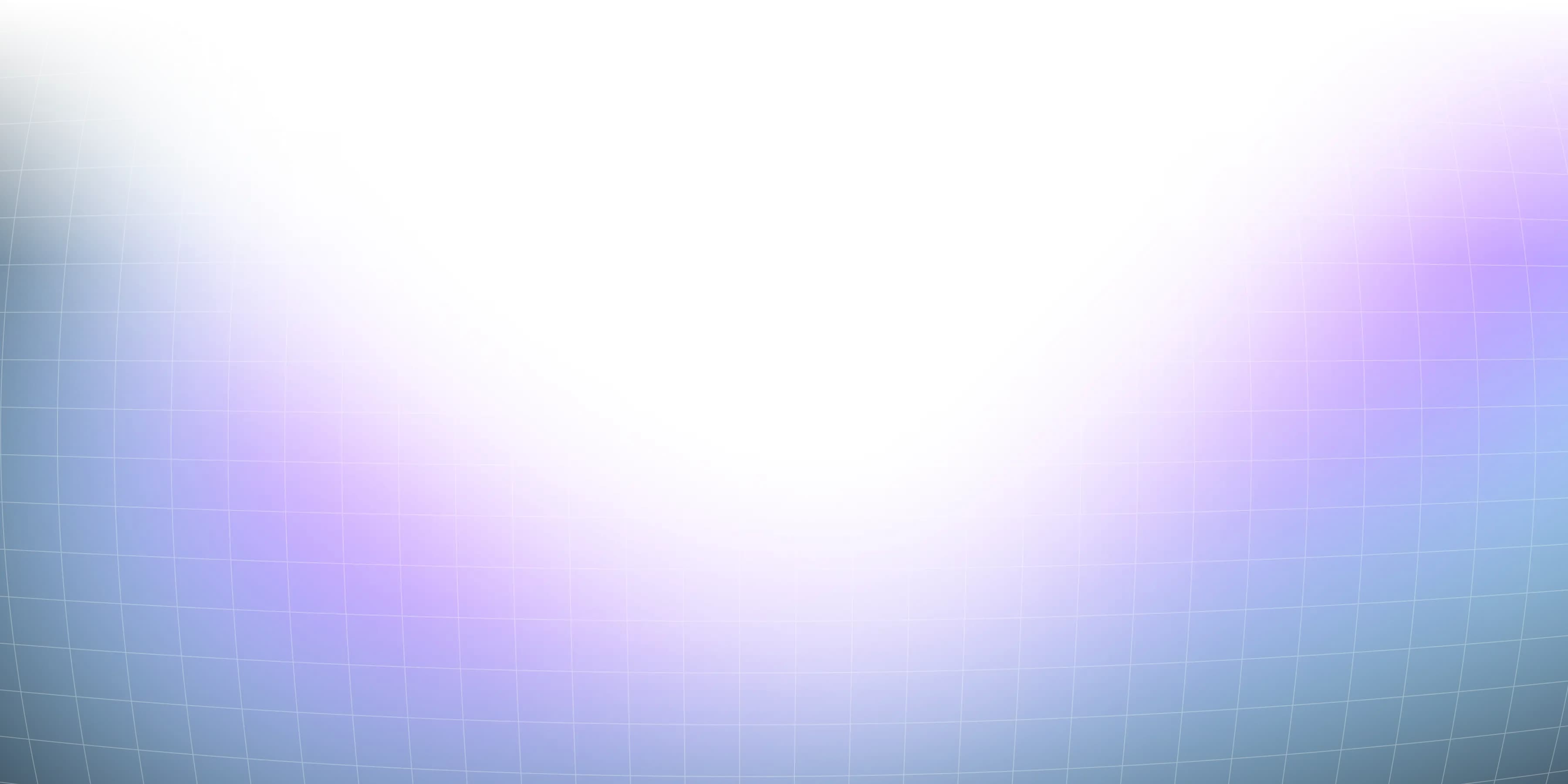
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
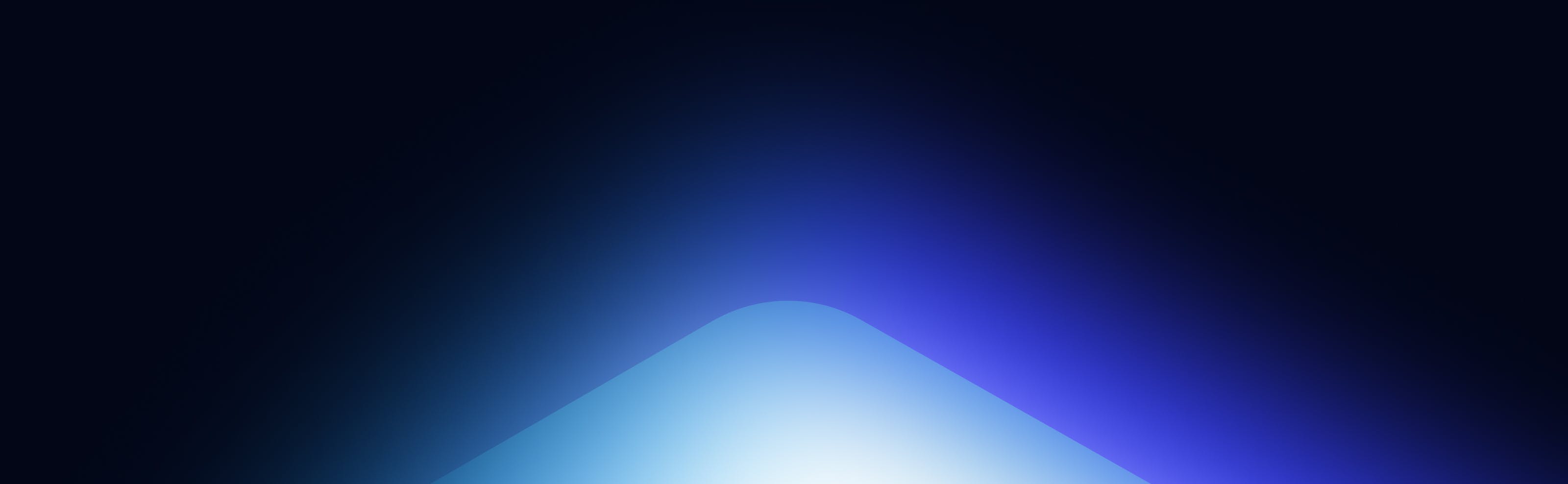
Build blockchain magic
Alchemy combines the most powerful web3 developer products and tools with resources, community and legendary support.