What is the Solidity console log?
Written by Turja

Reviewed by Brady Werkheiser
Debugging is a vital and reoccurring step in learning how to write Solidity smart contracts, patch vulnerabilities, and fix problematic bugs. The two typical development errors in smart contracts consist of runtime errors and logic errors.
Leveraging tools that empower the understanding of developers allows for an easier developer experience, especially when it comes to fixing errors in programs. Similar to JavaScript development, the console.log()
function is used to return an output message to the web console, which helps the debugging process.
The console.log()
accepts a parameter (i.e. array, object or message) and prints the value in the web console for developers to evaluate. This method enables developers to navigate the issue that may occur through outlining the output of the code step-by-step.
In this article, we’ll show you the main way to debug Solidity, review debugging tools in Hardhat, Foundry, Truffle, and Brownie, and then provide debugging tips.
What is the Solidity console log?
Logging to the console is a common tool used to debug code, and console.log()
is a function from a contract library provided by Hardhat. Logging outputs information to the console helps developers understand and troubleshoot issues in their program. In Solidity, this would be referred to as an event.
Ethereum offers a logging functionality which stores data in the transaction’s log data structure in the blockchain. Solidity events provide an abstraction to this functionality as it enables developers to output data on the blockchain.
Because the logs stay intact with the contract address in the blockchain and remain accessible with the block, Solidity developers can query and reference the blockchain for the specific transaction data.
Use Cases for Using Solidity Events
Solidity events can be used in a variety of methods including testing smart contracts, indexing for transaction data, referencing for frontend development and more. Most commonly, developers will use events in three uses cases:
In smart contracts to return values that can be used in the frontend
Asynchronous trigger for the smart contract to emit an event that triggers the frontend to do an action
Cheaper form of storage as the data is stored in the transaction log.
Within events, the parameters can be specified as indexed or non-indexed. The transaction data within a log will be viewed as encoded if the contract is verified, otherwise it will display as non-indexed or hashed.
In other cases, if the development process takes place within supportive environments such as Hardhat, the console.log()
function can be used in addition to events and this would function similarly to JavaScript development.
Why are console logs important?
Console logs serve as a necessary tool for developers in the smart contract debugging process. Console log is a combination of the testing environment of the program (console) and a function used to output data (log()).
In essence, console log provides an output of the program in a test environment that can be leveraged in the development and debugging process. If an error or issue arises in the program, the console log would be used as a primary step to inspect the code and identify the root cause of a bug.
While writing Solidity smart contracts, console logs similarly serve as an effective step in the debugging process to extract the code and understand the logic.
How does logging in Solidity work?
Logging, otherwise known as event-watching protocol or event, results in signals that are generated by transactions and blocks, which are used to provide information that cannot be obtained by the smart contract.
Solidity has a concept of an 'event'. Events can be emitted in smart contract functions and can have 0 to 4 topics which are indexed for quick lookup. In Solidity the 'emit' keyword is used to emit events. This is translated into one of the EVM's opcodes, LOG0, LOG1, ... , LOG4.
Because contact data lives in the States trie and event data is stored in the Transaction Receipts trie, smart contracts cannot read event data.
The transaction data or log entries consist of the:
Address - the address of the contract
Topic - the indexed values of the event
Data - the ABI encoded values or non-indexed values of the event
Solidity Debugging Tools in Hardhat
Hardhat is an Ethereum development environment focused on enabling developers to locally develop Solidity smart contracts and empower them with essential Solidity debugging tools.
Hardhat provides a suite of tools for testing, compiling, deploying and debugging dApps. By leveraging the Hardhat Network, developers are able to create, compile, and deploy smart contracts from their local machine through the Solidity interface.
1. Console.log
Within the Hardhat Network, the console.log()
function can be used as a reliable tool to output logging messages and extract details directly from the Solidity code. The Hardhat Network is designed to provide flexible and comprehensive execution inspection regardless of transaction failures.
How to Print to Solidity Console Logs
Solidity console logs can be printed in a structure similar to JavaScript. Once the import is completed, the console.log()
function can be used within the function to print specific output depending on the context of the smart contract. The following code outlines the process.
First, import hardhat/console.sol into the smart contract
pragma solidity ^0.8.9;
import "hardhat/console.sol";
contract BookStore {
//...
}
Next, add the console.log()
function into a specific function within the smart contract:
function transfer(address to, uint256 amount) external {
require(balances[msg.sender] >= amount, "Not enough tokens");
console.log(
"Transferring %s tokens to %s",
Amount,
to,
msg.sender
);
balances[msg.sender] -= amount;
balances[to] += amount;
emit Transfer(amount, to, msg.sender);
}
Note: Hardhat's console log tool can only be used once hardhat/console.sol is imported into the smart contract.
2. Solidity Stack Traces
Hardhat's stack trace tool is a combination of JavaScript and Solidity that provides a report when a transaction occurs or a call fails. This automated error reporting provides developers with valuable information to evaluate and debug their smart contract.
3. Explicit Errors
Hardhat provides a variety of possible errors that can help developers simplify the debugging process including:
general
network
task definition
arguments
dependencies resolution
built-in tasks
artifacts
plugins
internal
source names
contract names
Solidity Console Log Tip: Use Events and Functions to Improve Debugging
Events can be used to output data from the transaction logs to the console for both debugging and production stages. An event serves as a marker to indicate if a process takes place. Additionally, events will indicate if an issue has occurred at particular steps. Such a process is similar to the “require” keyword in Solidity, which checks if a condition is true and allows code to flow only if and when the required condition is true.
To start using events and functions to improve debugging, create an event that is properly defined and emit the event in the function. These two steps ensure that the event will occur once the function is called.
Additional Solidity Console Log Tools
While Hardhat is the industry standard Solidity development tool for building, testing, and debugging smart contracts, there are a few alternative tools for troubleshooting Solidity code including Foundry, Truffle, and Brownie.
1. Foundry
Foundry offers a suite of Ethereum smart contract development tools that enables the management of dependencies, smart contract compilation, deployment, testing, and on-chain interactions.
The Foundry toolkit can be integrated with Hardhat to leverage its console.log() function for the debugging process. Developers can call the function with up to 4 parameters, including uint, string, bool and address.
Forge
Forge is a command-line (CLI) tool integrated with Foundry to test, build, and deploy smart contracts. This testing framework enables developers to create tests in Solidity using JavaScript or TypeScript as well as unlock numerous features for the debugging process.
The forge test
command automates the Solidity tests and further provides a summary of the results including logs and stack traces. The forge debug
command is also an interactive debugger that reviews a single smart contract as a script to identify any errors in the program.
Cast
Cast is a command-line interface (CLI) tool that interacts with Ethereum RPC calls. This consists of smart contracts calls, transactions, or chain data retrieval via the command line.
Anvil
Anvil is a local testnet node integrated with Foundry to enable smart contract deploying and testing from the frontend or via interactions over RPC (Remote Procedure Calls) with EVM (Ethereum Virtual Machine) compatible networks.
2. Truffle
Truffle offers three Ethereum smart contract developer tools: a development environment, a testing framework and an asset pipeline for blockchains. Truffle’s inclusive ecosystem provides developers with various tools to create, test, and debug end-to-end decentralized applications (dApps).
These tool sets offered by Truffle are:
Smart contract toolkit - compilation, linking, deployment, etc.
Debugging - breakpoints, variable analysis, step functionality, automated contract testing
Management - network, package, migrations frameworks
Learn More: https://trufflesuite.com/docs/truffle/
3. Brownie
Brownie is a Python-based development and testing framework for smart contracts in Ethereum with support for Solidity and Vyper, and it is divided into 4 categories: testing, debugging, interaction and deployment. Each step supports developers in creating flexible programs.
Developers can access various debugging tools to gather detailed information about transaction failures and to locate, replicate, and troubleshoot Solidity errors.
TransactionReceipt.revert_ms - get a direct explanation message for the transaction failure
TransactionReceipt.error() - locate the root cause and section of the smart contract that caused a failed transaction
TransactionReceipt.events - view events that fired in reverted transactions
TransactionReceipt.trace - view a list of dictionaries containing the transaction information
TransactionReceipt.call_trace() - view a full map (trace) of the transaction steps
Developers can also leverage pytest for unit testing smart contracts, then review the stack trace report that is provided.
Learn More: https://eth-brownie.readthedocs.io/en/v1.2.1/index.html
Learn About the Solidity Console Log with Alchemy University
This article has introduced you to the most popular logging and debugging tools for developing Solidity smart contracts. If you’re learning Solidity, selecting the best tools for smart contract debugging is essential for solving the issues you face in Solidity development.
To start or accelerate your Solidity development training, explore Alchemy University's free, 7-week Solidity developer bootcamp, originally a $3,000 certification course taught by ChainShot, is now fully integrated with Alchemy! If you are new to development in general, Alchemy's 3-week JavaScript crash course is a great prerequisite before starting an Ethereum bootcamp.
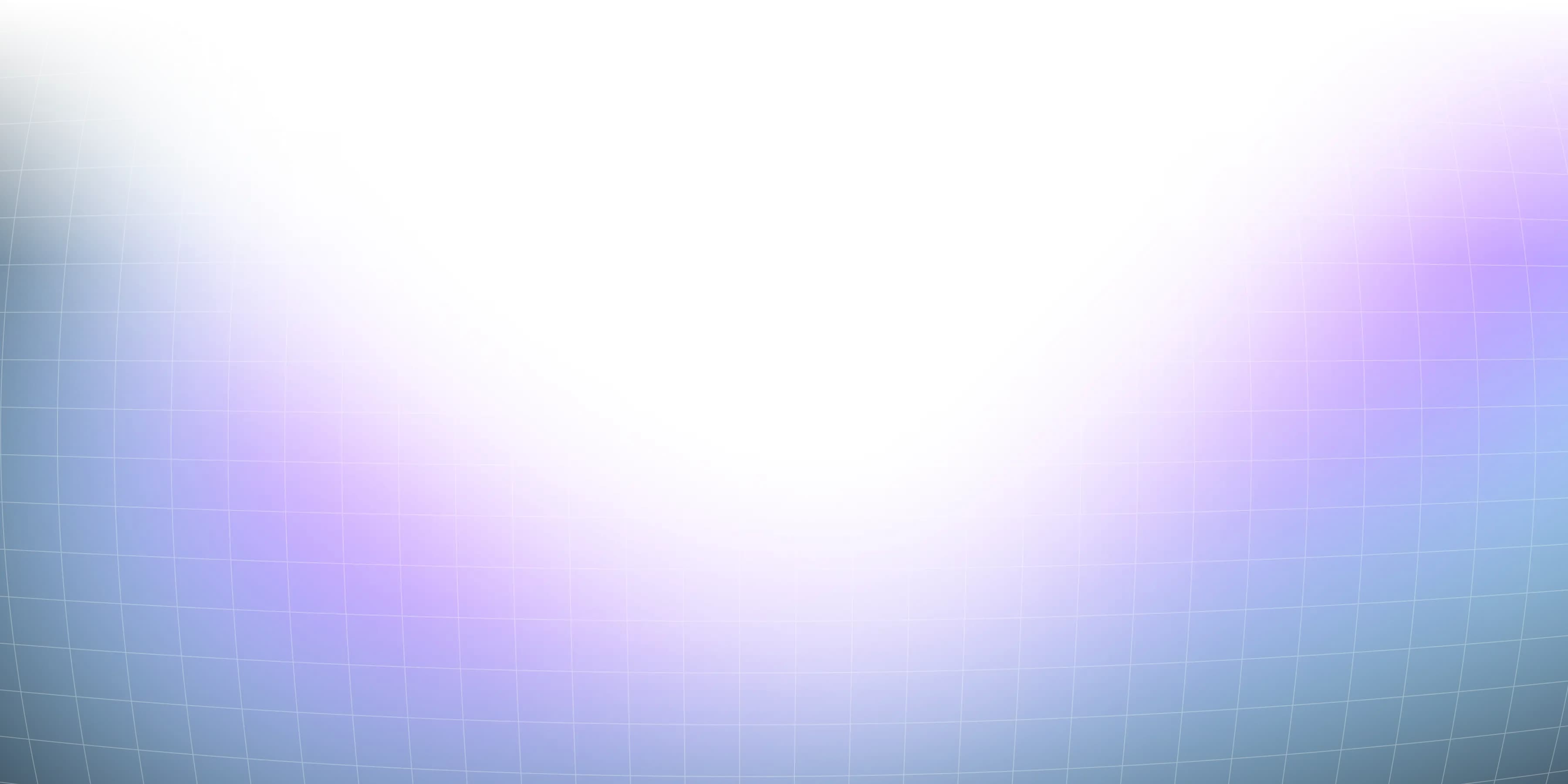
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
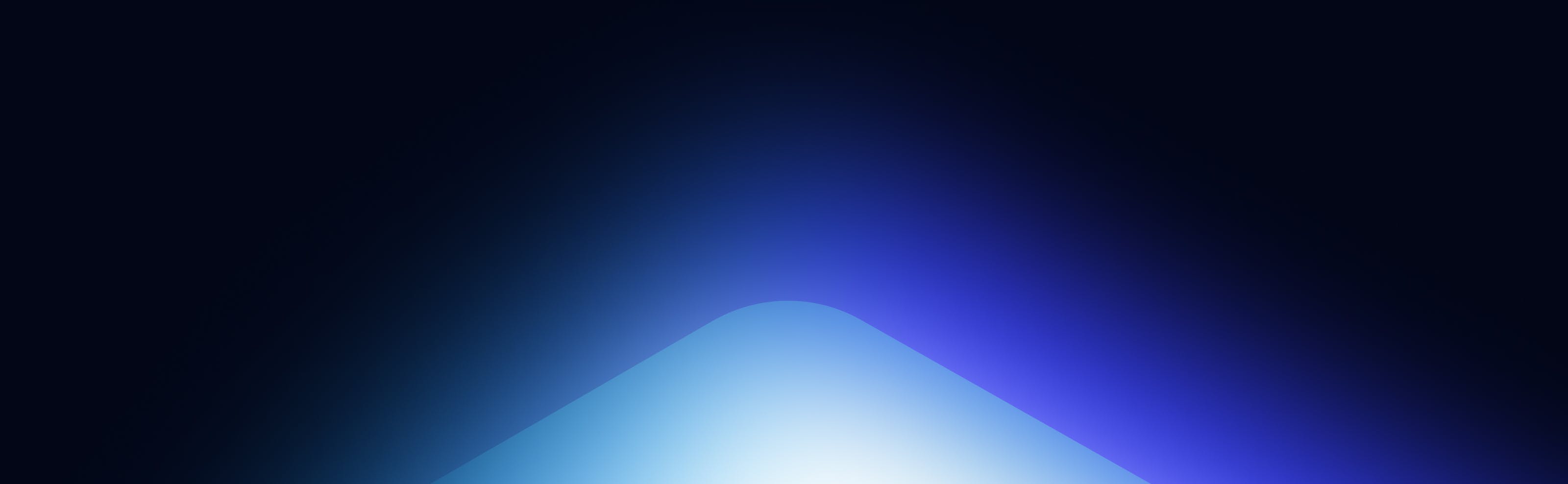