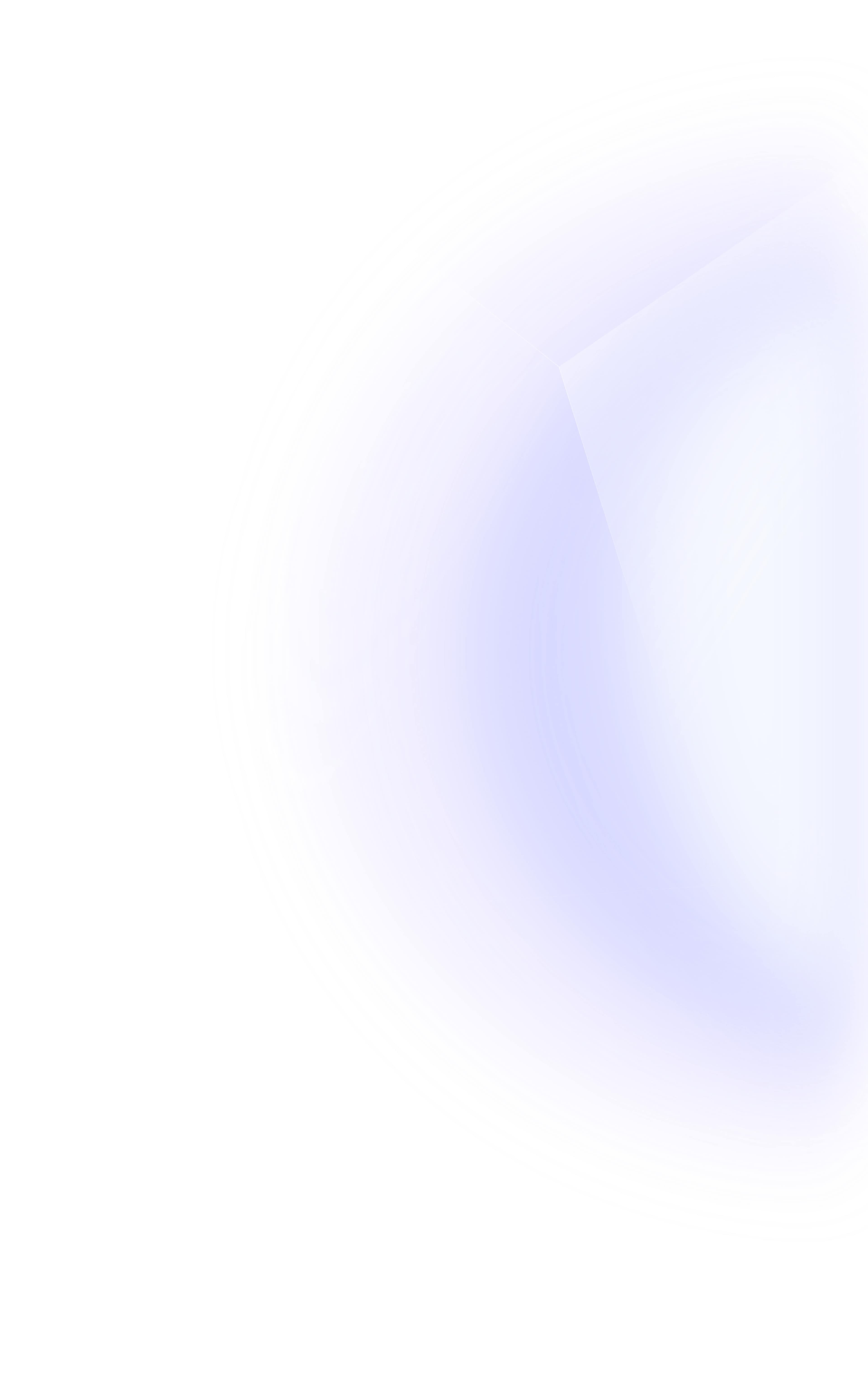
How do Yul contract calls work?

Written by Mark Jonathas

Reviewed by Brady Werkheiser
Yul is an intermediate programming language that can be used to write a form of assembly language inside smart contracts. Now that you understand syntax, storage, and how memory works in Yul, it’s time to call contracts!
How do contract calls work in Yul?
In the final section of this series we will look at how contract calls work in Yul. Before we dive into some examples we need to learn some more Yul Operations first. Let’s take a look.
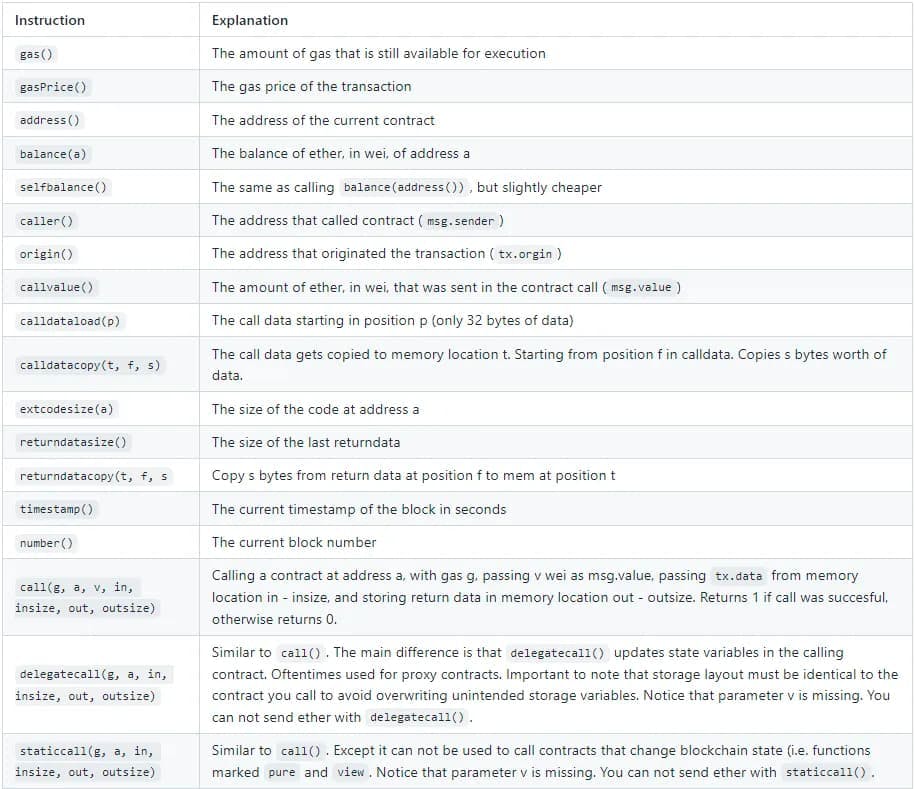
Ok, now let’s look at some new contracts for these examples. First, let’s look at the contract we will be calling.
pragma solidity^0.8.17;
contract CallMe {
uint256 public var1 = 1;
uint256 public var2 = 2;
function a(uint256 _var1, uint256 _var2) external payable returns(uint256, uint256) {
// requires 1 ether was sent to contract
require(msg.value >= 1 ether);
// updates var1 & var2
var1 = _var1;
var2 = _var2;
// returns var1 & var2
return (var1, var2);
}
function b() external view returns(uint256, uint256) {
return (var1, var2);
}
}
This contract has two storage variables var1 and var2 that are stored in storage slots 1 and 2 respectively.
Function a() requires the user to send at least 1 ether to the contract, otherwise it reverts.
Next, function a() updates var1 and var2 and returns them.
Function b() simply reads var1 and var2 and returns them.
Before we move onto our contract that calls contract CallMe, we need to take a minute to understand function selectors.
Let’s look at the following call data for a transaction 0x773d45e000000000000000000000000000000000000000000000000000000000000000010000000000000000000000000000000000000000000000000000000000000002
.
The first 4 bytes of the calldata is what's called the function selector (0x773d45e0). This is how the EVM knows what function you want to call. We derive the function selector by getting the first 4 bytes of the hash of a string of the function signature.
So function a()’s signature would be a(uint256,uint256).
Taking the hash of this string gives us: 0x773d45e097aa76a22159880d254a5f1db8365bc2d0f0987a82bda7dfd3b9c8aa
.
Looking at the first 4 bytes we see it equals 0x773d45e0.
Notice the lack of spaces in the signature. This is important because adding spaces will give us a completely different hash. You do not have to worry about getting the selectors for our code examples, I will provide them.
Let’s start by looking at the storage layout.
uint256 public var1;
uint256 public var2;
bytes4 selectorA = 0x773d45e0;
bytes4 selectorB = 0x4df7e3d0;
Notice how var1 & var2 have the same layout as contract CallMe. You may remember me saying that the layout has to be the same as our other contract for delegatecall() to work properly.
We satisfy those needs and are able to have other variables (selectorA & selectorB) as long as our new variables are appended to the end. This prevents any storage collisions.
We are now ready to make our first contract call.
How to Use staticcall() in Yul
Let’s start with something simple, staticcall(). Here is our function:
function getVars(address _callMe) external view returns(uint256, uint256) {
assembly {
// load slot 2 from memory
let slot2 := sload(2)
// shift selectorA off
let funcSelector := shr( 32, slot2)
// store selectorB to memory location 0x80
mstore(0x00, funcSelector)
// static call CallMe
let result := staticcall(gas(), _callMe, 0x1c, 0x20, 0x80, 0xc0)
// check if call was succesfull, else revert
if iszero(result) {
revert(0,0)
}
// return values from memory
return (0x80, 0xc0)
}
}
Here’s what is happening:
Retrieve b()’s function selector from storage by loading slot 2 (both selectors are packed into one slot)
Shift right by 4 bytes (32 bits) to isolate selectorB
Store the function selector in the scratch space of memory
Make our static call
Pass in gas() (you can also specify the amount of gas)
Pass in the parameter _callMe for the contract address*
Check if the function call was successful, otherwise we return with no data.
Return our data from memory and see the values 1 and 2
Note: *0x1c and 0x20 say we want to pass the last 4 bytes of what we stored to the scratch space. The last two staticcall() parameters specify we want to store the return data in memory locations 0x80 - 0xc0.
How to Use call() in Yul
Next let’s look at call(). We are going to call function a() from CallMe. Remember to send at least 1 ether to the contract! I will be passing in 3 and 4 as _var1 & _var2 for this example.
Here is the code:
function callA(address _callMe, uint256 _var1, uint256 _var2) external payable returns (bytes memory) {
assembly {
// load slot 2
let slot2 := sload(2)
// isolate selectorA
let mask := 0x000000000000000000000000000000000000000000000000000000000ffffffff
let funcSelector := and(mask, slot2)
// store function selectorA
mstore(0x80, funcSelector)
// copies calldata to memory location 0xa0
// leaves out function selector and _callMe
calldatacopy(0xa0, 0x24, sub( calldatasize(), 0x20 ) )
// call contract
let result := call(gas(), _callMe, callvalue(), 0x9c, 0xe0, 0x100, 0x120 )
// check if call was succesfull, else revert
if iszero(result) {
revert(0,0)
}
// return values from memory
return (0x100, 0x120)
}
}
Ok, so similar to our last example we have to load slot2. This time, however, we are going to mask selectorB to isolate selectorA.
Now we will store the selector at 0x80.
Since we need parameters from the calldata, we are going to use calldatacopy(). We are telling calldatacopy() to:
store our calldata at memory location 0xa0
skip the first 36 bytes*
store the size of the calldata minus 36 bytes
Note: *the first 4 bytes we skip are the function selector for callA() and the next 32 bytes is the address of callMe.
Now we are ready to make our contract call!
Like last time, we pass in gas() and _callMe. However, this time we pass in our call data from 0x9c (last 4 bytes of 0x80 memory series) - 0xe0, and store our data in memory location 0x100 - 0x120.
Again, we check if the call was successful and return our output. If we check contract CallMe we see the values were successfully updated to 3 and 4.
For extra clarification for what’s happening, here is the memory layout right before we return:
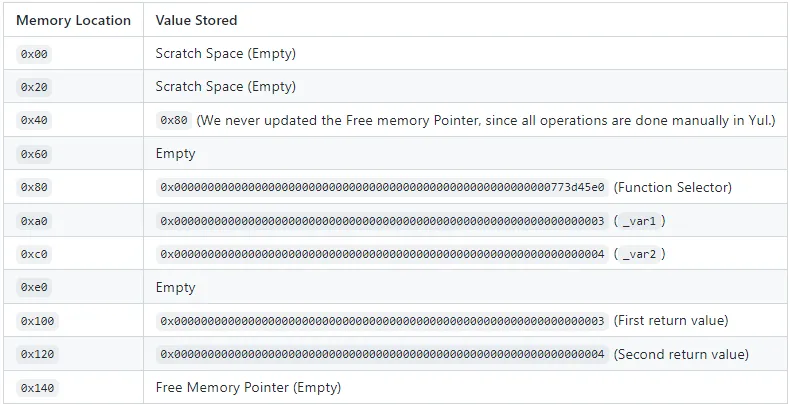
How to Use Delegate Call in Yul
In our last section we will look at delegatecall(). The code will look almost identical to call() with only one change.
function delgatecallA(address _callMe, uint256 _var1, uint256 _var2) external payable returns (bytes memory) {
assembly {
// load slot 2
let slot2 := sload(2)
// isolate selectorA
let mask := 0x000000000000000000000000000000000000000000000000000000000ffffffff
let funcSelector := and(mask, slot2)
// store function selectorA
mstore(0x80, funcSelector)
// copies calldata to memory location 0xa0
// leaves out function selector and _callMe
calldatacopy(0xa0, 0x24, sub( calldatasize(), 0x20 ) )
// call contract
let result := delegatecall(gas(), _callMe, 0x9c, 0xe0, 0x100, 0x120 )
// check if call was successful, else revert
if iszero(result) {
revert(0,0)
}
// return values from memory
return (0x100, 0x120)
}
}
The only change we made was changing call() to delegatecall() and removing callvalue().
We do not need a callvalue(), because delegate call executes the code from CallMe inside its own state. Therefore the require() statement in a() is checking if ether was sent to our Caller contract. If we check var1 and var2 in CallMe, we see no changes. However, var1 and var2 in our Caller contract was updated successfully.
This wraps up our section on contract calls! Next, learn how Memory, Storage, and Smart Contract Calls work in Yul.
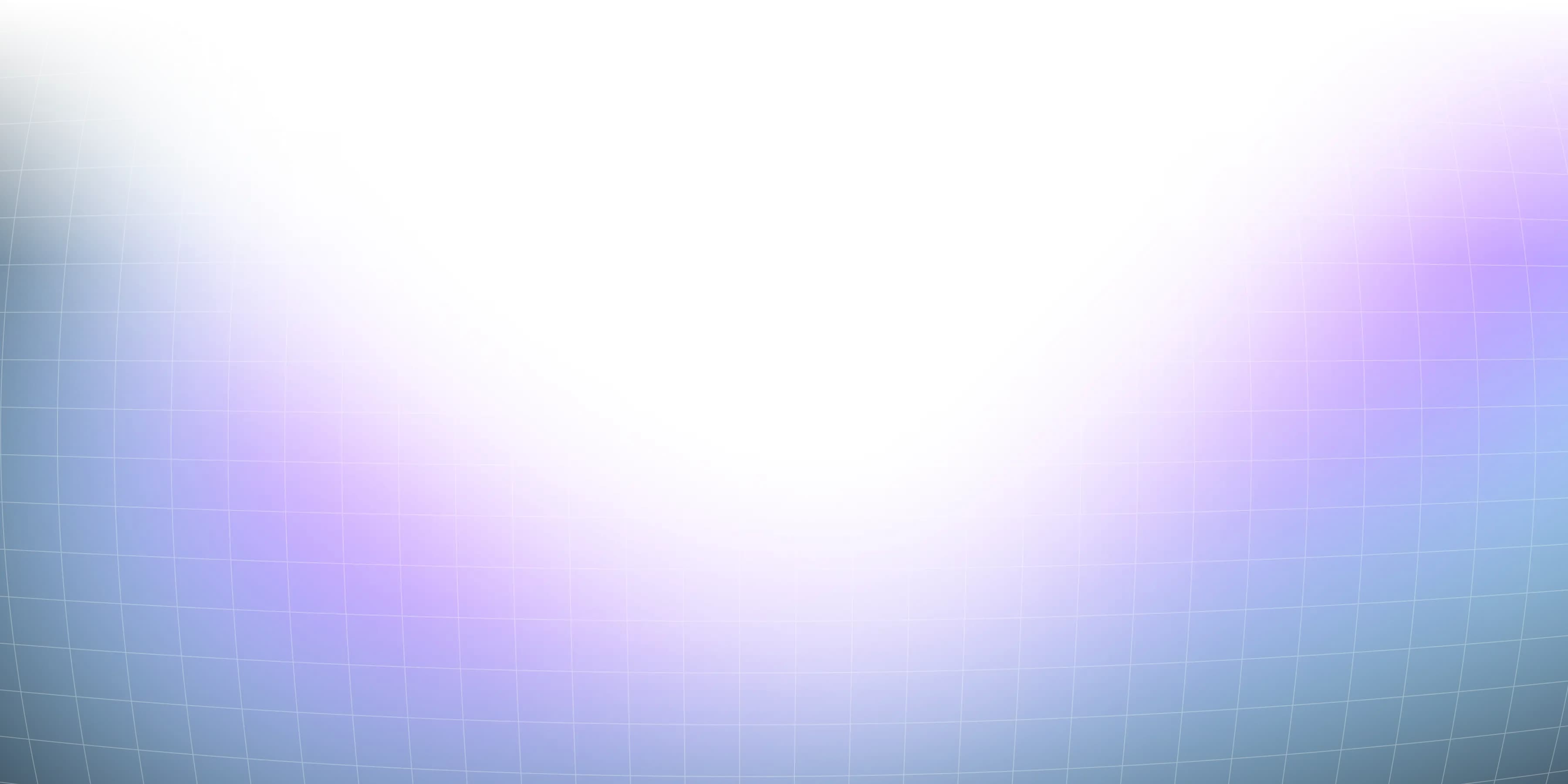
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
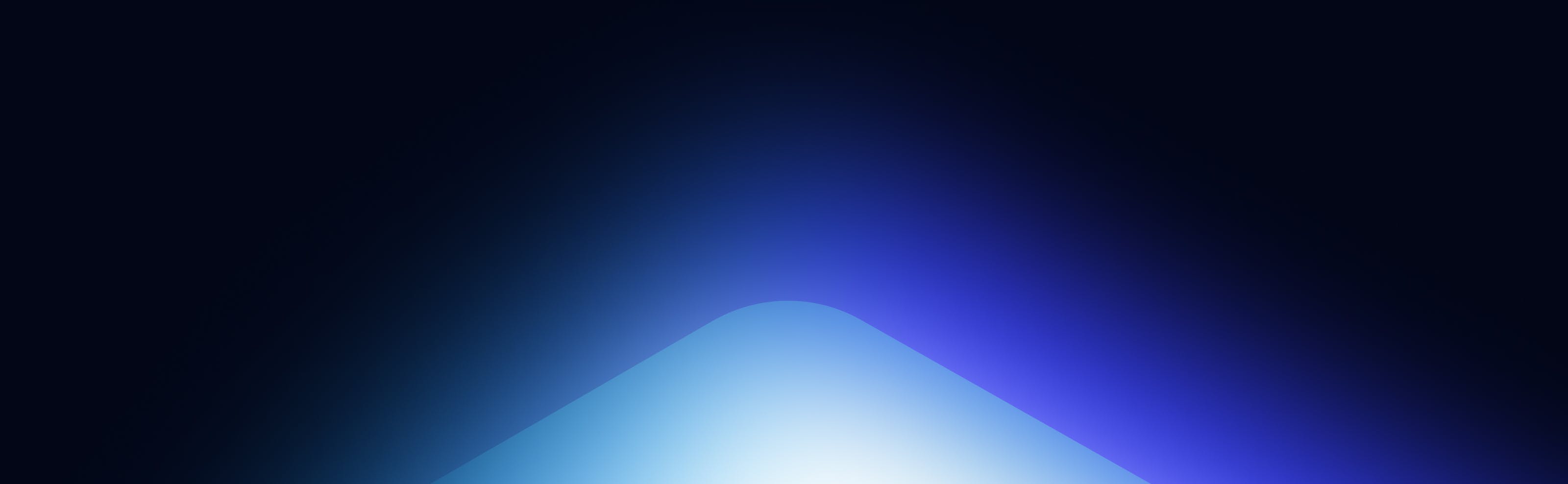