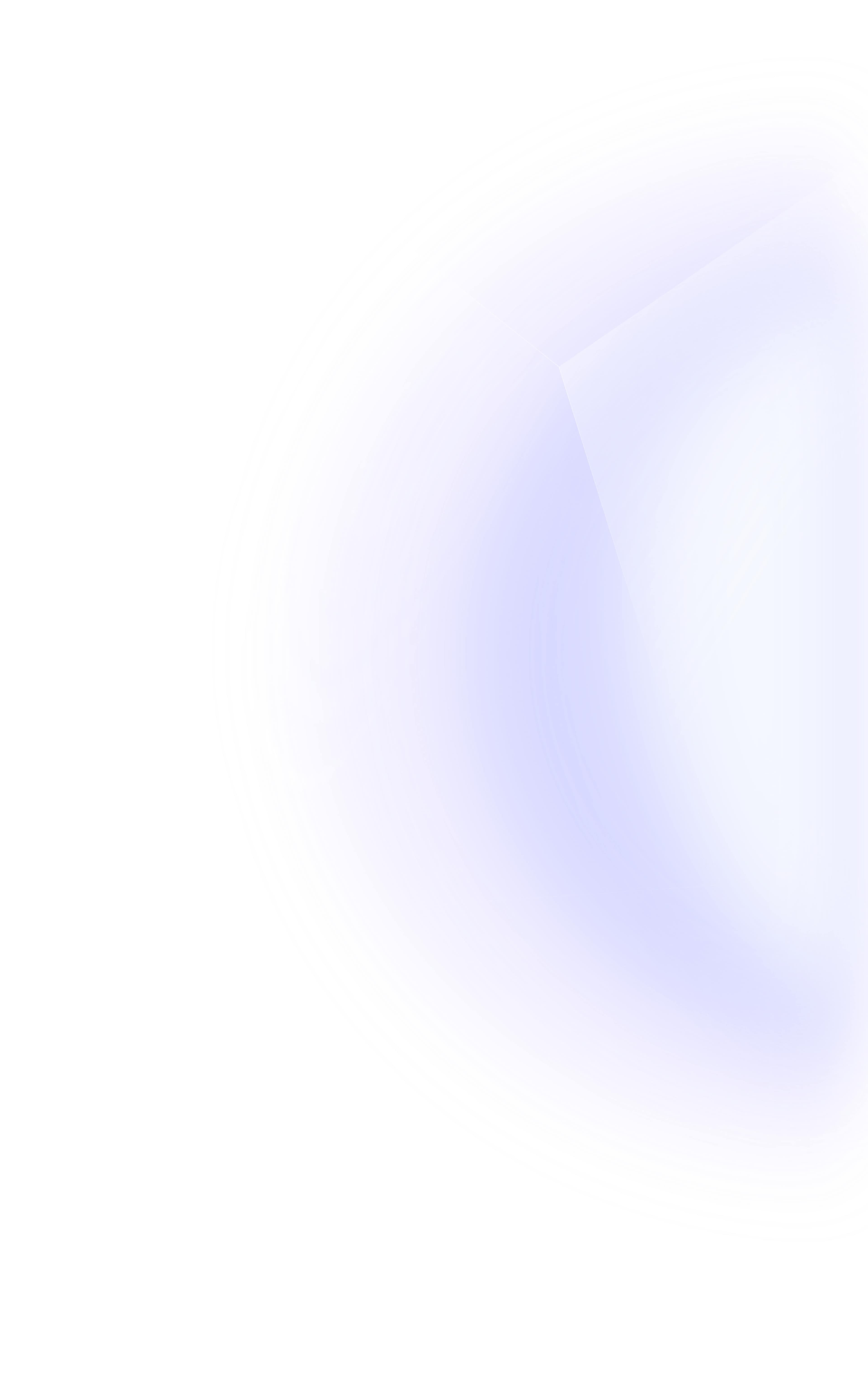
How to Read and Write Packed Storage Variables in Yul

Written by Mark Jonathas

Reviewed by Brady Werkheiser
Yul is an intermediate programming language that can be used to write a form of assembly language inside smart contracts. After learning about the syntax of Yul and how storage works, it’s important to understand how to read and write packed storage variables in Yul.
How to Read and Write Packed Storage Variables in Yul
Suppose you want to change var5 to 4. We know that var5 is located in slot 3, so you might try something like this:
function writeVar5(uint256 newVal) external {
assembly {
sstore(3, newVal)
}
}
Using getValInHex(3) we see that slot 3 has been rewritten to:
0x0000000000000000000000000000000000000000000000000000000000000004
That’s a problem because now var4 has been rewritten to 0. In this section we are going to go over how to read and write packed variables, but first we need to learn a little more about Yul syntax.
If you’re unfamiliar with these operations don’t worry, we are about to go over them with examples.
Let’s start with and(). We are going to take two bytes32 and try the and() operator and see what it returns.
function getAnd() external pure returns (bytes32) {
bytes32 randVar = 0x0000000000000000000000009acc1d6aa9b846083e8a497a661853aae07f0f00;
bytes32 mask = 0xffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff;
bytes32 ans;
assembly {
ans := and(mask, randVar)
}
return ans;
}
If you look at the output we see:
0x0000000000000000000000009acc1d6aa9b846083e8a497a661853aae07f0f00
The reason for this is because what the and() does is it looks at each bit from both inputs and compares their values.
If both bits are a 1 (think of it in terms of binary: active or inactive), then we keep the bit as it is.
Otherwise it gets set to 0.
Now look at the code for or().
function getOr() external pure returns (bytes32) {
bytes32 randVar = 0x000000000000000000000000ffffffffffffffffffffffffffffffffffffffff;
bytes32 mask = 0xffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff;
bytes32 ans;
assembly {
ans := or(mask, randVar)
}
return ans;
}
This time the output is:
0xffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff
This is because it looks to see if either bit is active.
Let’s look at what happens if we change the mask variable to:
0x00ffffffffffffffffffffff0000000000000000000000000000000000000000
As you can see the output changes to:
0x00ffffffffffffffffffffff9acc1d6aa9b846083e8a497a661853aae07f0f00
Notice the first byte is 0x00, because neither input has any active bits for the first byte.
xor() is a little bit different. It requires one bit to be active (1) and the other bit to be inactive (0).
Here is a code demonstration:
function getXor() external pure returns (bytes32) {
bytes32 randVar = 0x00000000000000000000000000000000000000000000000000000000000000ff;
bytes32 mask = 0xffffffffffffffffffffffff00000000000000000000000000000000000000ff;
bytes32 ans;
assembly {
ans := xor(mask, randVar)
}
return ans;
}
The output is:
0xffffffffffffffffffffffff0000000000000000000000000000000000000000
The key difference is apparent when we see the only active bits in the output are when 0x00 and 0xff are aligned.
shl() and shr() operate very similarly to each other. Both shift the input value by an input amount of bits. shl() shifts to the left and shr() shifts to the right.
Let’s take a look at some code!
Output:
ans1: 0x0000ffff00000000000000000000000000000000000000000000000000000000
ans2: 0x00000000000000000000000000000000000000000000000000000000ffff0000
Let’s start by looking at ans1. We perform shr() by 16 bits (2 bytes). As you can see the last two bytes change from 0xffff to 0x0000, and the first two bytes are shifted two bytes to the right. Knowing this, ans2 seems self explanatory; all that happens is the bits are shifted to the left instead of the right.
Before we write to var5, let's write a function that reads var4 and var5 first.
function readVar4AndVar5() external view returns (uint128, uint128) {
uint128 readVar4;
uint128 readVar5;
bytes32 mask = 0x00000000000000000000000000000000ffffffffffffffffffffffffffffffff;
assembly {
let slot3 := sload(3)
// the and() operation sets var5 to 0x00
readVar4 := and(slot3, mask)
// we shift var5 to var4's position
// var5's old position becomes 0x00
readVar5 := shr( mul( var5.offset, 8 ), slot3 )
}
return (readVar4, readVar5);
}
The output is 1 & 2 as expected.
For retrieving var4 we just need to use a mask to set the value to:
0x0000000000000000000000000000000000000000000000000000000000000001
Then we return a uint128 set equal to 1.
When reading var5, we need to shift var4 off by shifting right.
This leaves us with this, which we can return:
0x0000000000000000000000000000000000000000000000000000000000000002
It is important to note that sometimes you will have to shift and mask in unison to read a value that has more than 2 variables packed into a storage slot.
Ok, we’re finally ready to change the value of var5 to 4!
function writeVar5(uint256 newVal) external {
assembly {
// load slot 3
let slot3 := sload(3)
// mask for clearing var5
let mask := 0x00000000000000000000000000000000ffffffffffffffffffffffffffffffff
// isolate var4
let clearedVar5 := and(slot3, mask)
// format new value into var5 position
let shiftedVal := shl( mul( var5.offset, 8 ), newVal )
// combine new value with isolated var4
let newSlot3 := or(shiftedVal, clearedVar5)
// store new value to slot 3
sstore(3, newSlot3)
}
}
The first step is to load storage slot 3.
Next, we need to create a mask.
Similarly to when we read var4, we want to isolate the value to:
0x0000000000000000000000000000000000000000000000000000000000000001
The next step is formatting our new value to be in var5’s slot position so it looks like this:
0x0000000000000000000000000000000400000000000000000000000000000000
Unlike when we read var5, we are going to shift our value to the left this time.
Finally, we are going to use or() to combine our values into 32 bytes of hexadecimal, and store that value to slot 3.
We can check our work by calling getValInHex(3).
This is going to return this, , which is what we are expecting to see:
0x0000000000000000000000000000000400000000000000000000000000000001
Great, you now know how to read and write to packed storage slots! Next, learn how Memory, Storage, and Smart Contract Calls work in Yul.
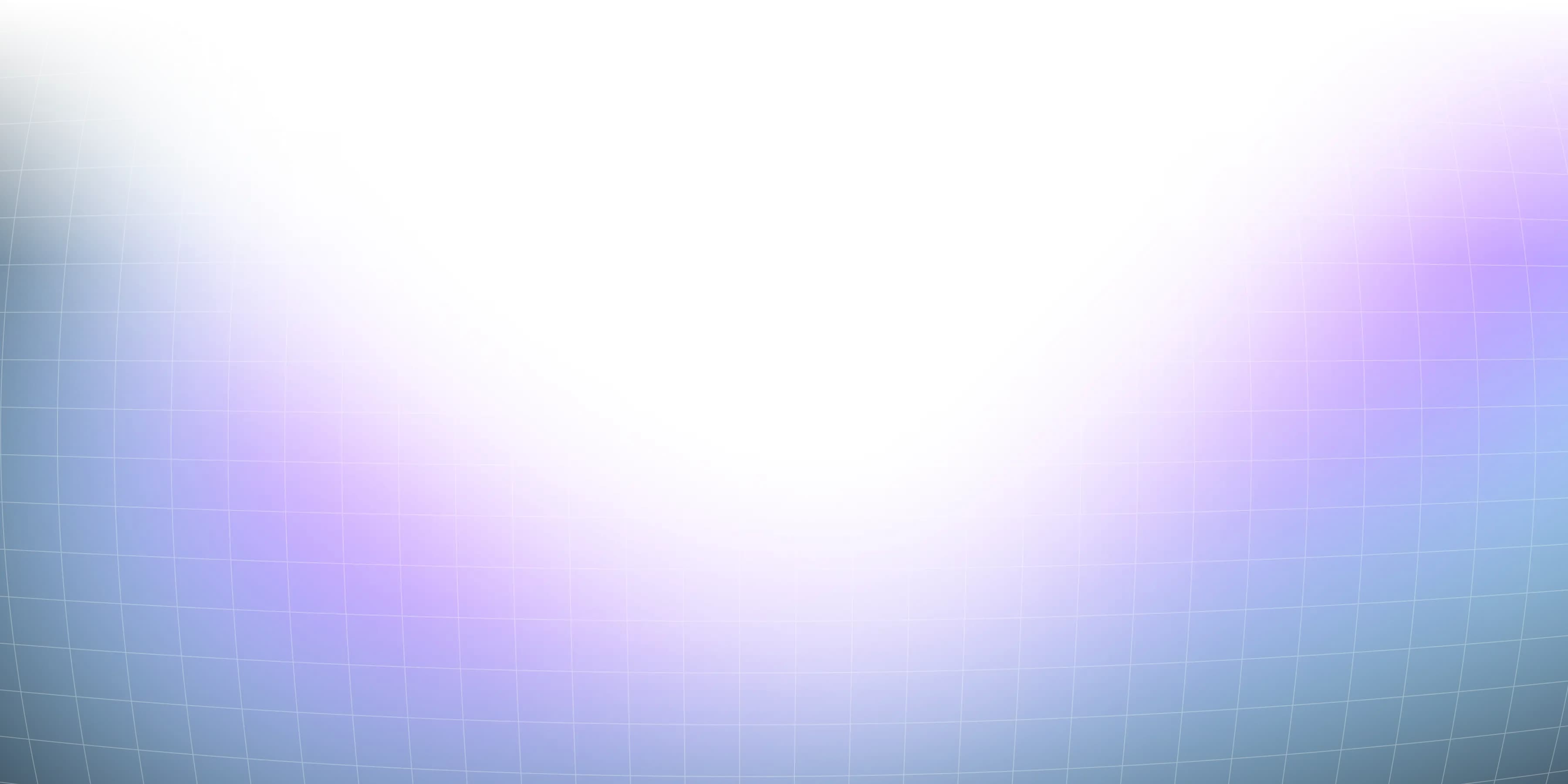
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
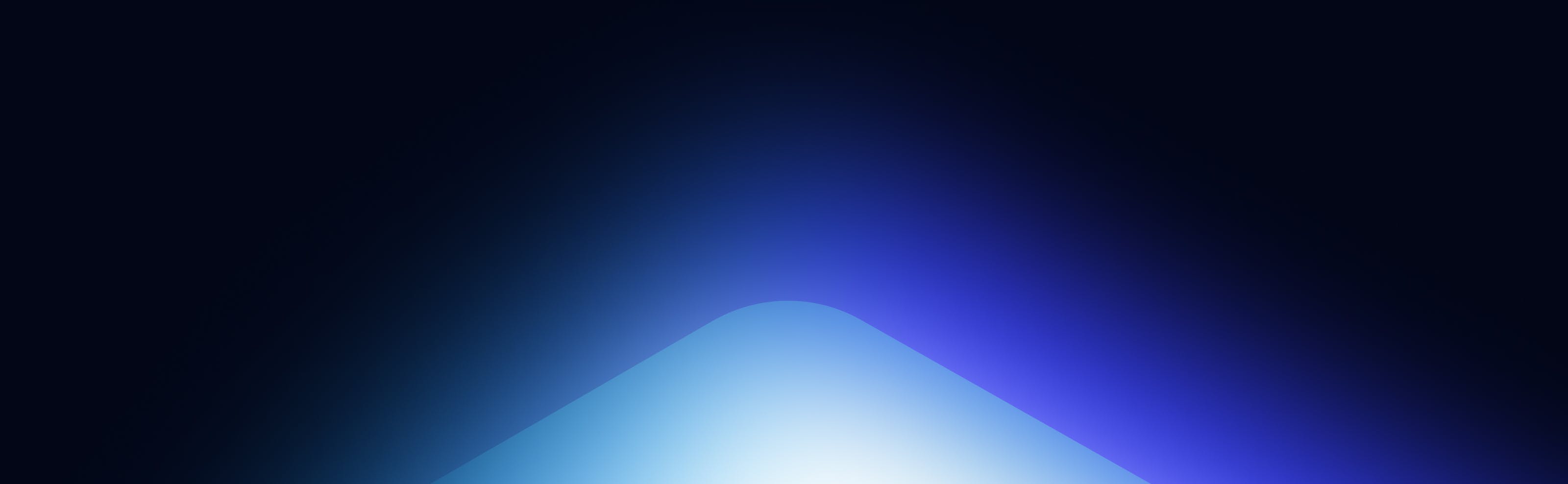
Build blockchain magic
Alchemy combines the most powerful web3 developer products and tools with resources, community and legendary support.