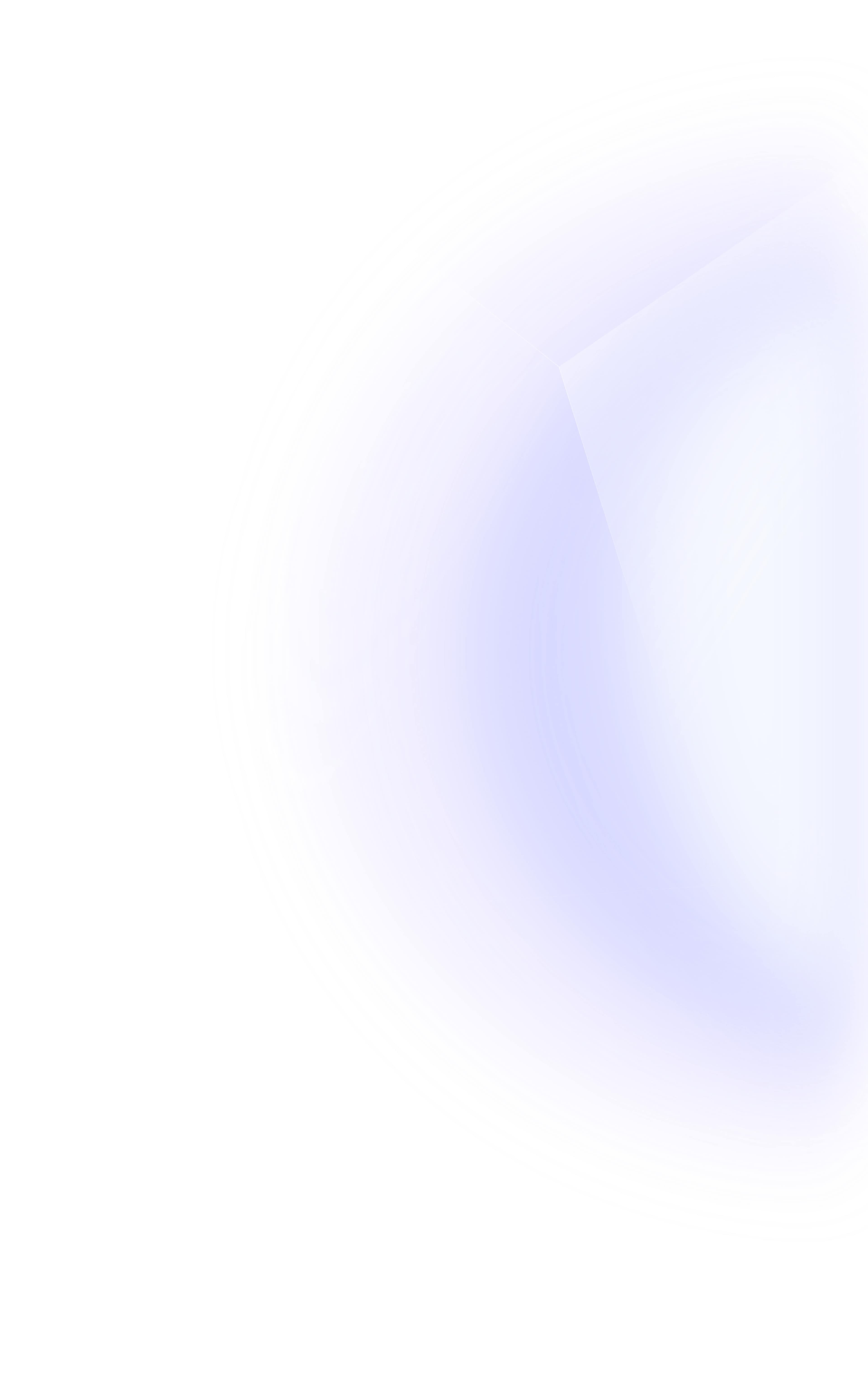
What is the Solidity call function?

Written by Alchemy

Reviewed by Brady Werkheiser
The Solidity programming language is primarily used to create smart contracts on the Ethereum blockchain. This article will cover all the details you need to know about what a Solidity call function is.
What is the Solidity call function?
The call
function in Solidity is a low level function developers use to interact with other contracts.
When building a Solidity smart contract, the call
method should be used anytime you want to interact with another contract from your own contract.
Calls can also be used to execute other functions in the recipient smart contract, using Ether provided by the caller to pay for the transaction. The call
function also has the advantage of returning the transaction status as a boolean with the return value sent as a variable.
What is calldata?
Calldata
is a type of temporary storage, containing the data specified in a function’s arguments. The difference between it and memory, another type of temporary storage, is that calldata’s immutability—whatever is stored inside calldata
cannot be changed.
How does the Solidity call function work?
The Solidity call function works by taking calldata
, which can be zero in the case of a native ETH transfer, and executing that calldata
on the intended recipient based on the low-level EVM opcode CALL.
When data (i.e. the function to be called in recipient smart contract) and gas are provided, the call
method is able to use these two to execute functions inside smart contracts.
How do you use the call method to send Ether?
As one of your Solidity functions, you can use the following code into your Solidity IDE of choice and replace the address payable _to
with the recipient address.
contract SendEther {
function sendViaCall(address payable _to) public payable {
// Call returns a boolean value indicating success or failure.
(bool sent, bytes memory data) = _to.call{value: msg.value}("");
require(sent, "Failed to send Ether");
}
}
Below is code which creates a contract that is capable of receiving Ether from calls.
contract ReceiveEther {
// Function to receive Ether. msg.data must be empty
receive() external payable {}
// Fallback function is called when msg.data is not empty
fallback() external payable {}
function getBalance() public view returns (uint) {
return address(this).balance;
}
}
What's the difference between call and delegatecall?
The difference between call
and delegatecall
is that delegatecall
will execute the called function as if its code was entirely part of the smart contract that is doing the calling. In contrast, the call
method will call the function as it is, as a part of another smart contract. In practice, this means the called function will use the caller's storage, msg.sender
, and msg.value
.
What's the difference between call and transfer?
Transfers have an unchangeable gas limit and will cancel on failure. Calls have a customizable gas limit by using someAddress.call.value(ethAmount).gas(gasAmount)()
in place of the usual call will return false if the transaction fails.
The transfer
method is no longer a recommended to use. However, historically, transfer
was preferred because it uses a built-in limit on gas, which helped prevent reentrancy exploits. The immutable gas limit on the transfer method also made it a better choice for computations where you wished to set an upper limit of 2300 gas.
How to Learn More About the Solidity Call Method
To continue learning about Solidity calls, secure your spot in Alchemy University's free, online Solidity developer crash course. This 7-week, asynchronous Ethereum bootcamp has been redesigned after Alchemy's acquisition of the leading Ethereum education company, ChainShot.
If developers are new to development in general, Alchemy University's 3-week JavaScript crash course is a great prerequisite before starting an Ethereum bootcamp.
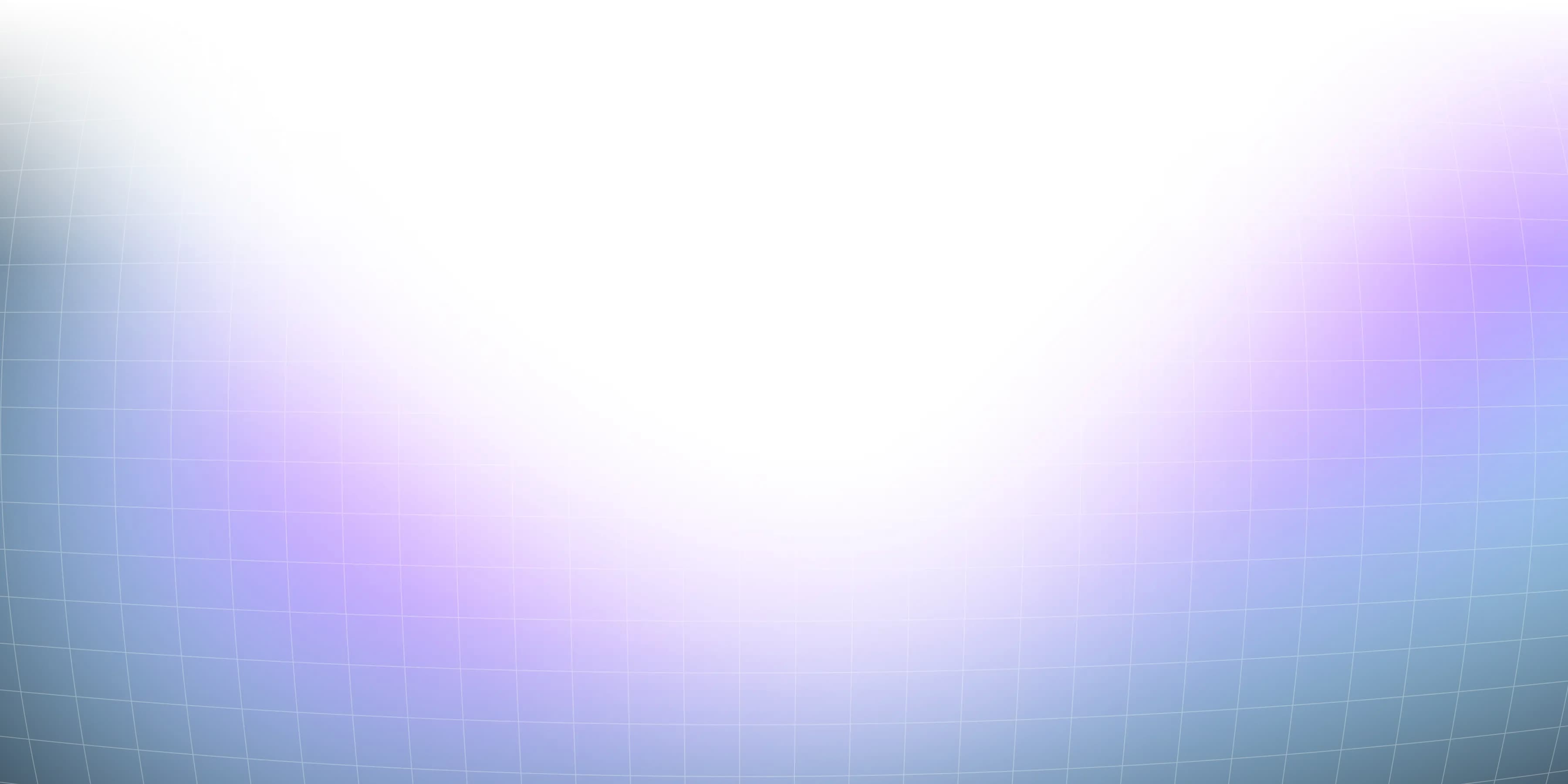
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
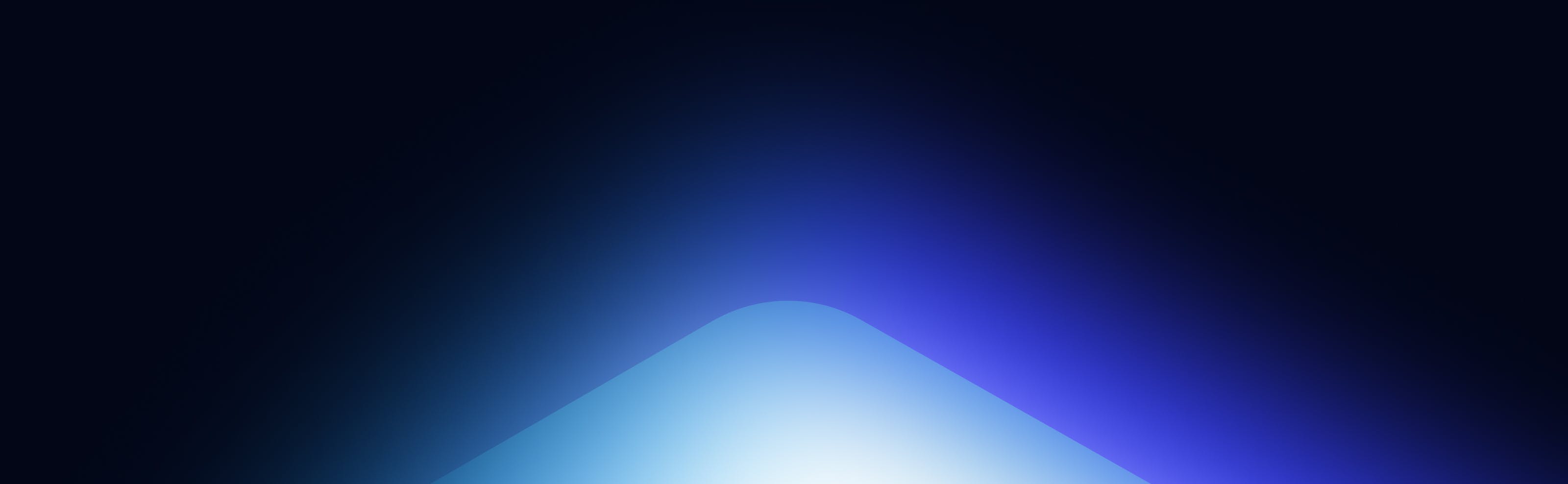