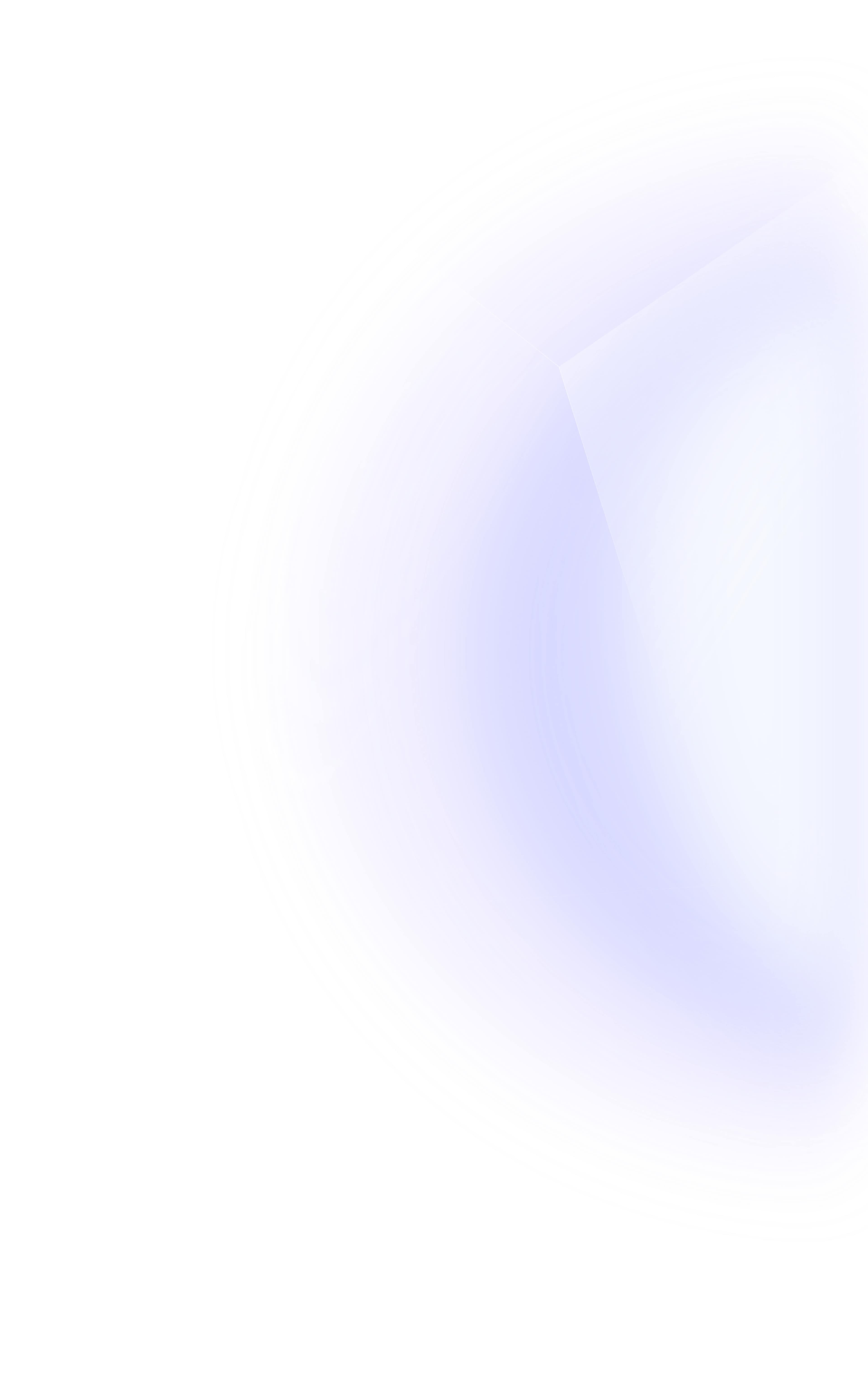
Solidity Data Types: Signed (int) and Unsigned Integers (uint)
Written by Turja

Reviewed by Brady Werkheiser
Solidity is a high-level, object-oriented, and statically typed language that developers use to deploy applications on the Ethereum blockchain. As a statically typed language, Solidity requires the developer to define the structure and data type of every state and local variable at compile time.
Thus, Solidity data types are essential to understand while learning Solidity smart contracts. Data types classify information stored in variables into different types such as integers, booleans, addresses, literals, arrays, and so on.
This article defines what data types in Solidity are and elaborates on common integer types such as uint and int.
What are Solidity data types?
Similar to other statically typed languages, Solidity data types specify the information stored in a variable, such as a specific value or a reference address of a memory location.
Data types in Solidity are flexible in that you can combine elementary types to form complex types such as integer arrays or structures with differently typed variables.
However, one of the key restrictions in Solidity is that the developer needs to specify the data type of every variable at compile time. Once declared, the compiler knows exactly how much space it needs to reserve for the variable.
Solidity data types can be broadly categorized into two main groups: value types and reference types.
What are value types?
Solidity value types, such as integers, are variables that store data within a defined memory space and pass a duplicated value when used within a function or an assignment. This value type stores a separate copy of the duplicated data type, so any change to the value of the copied value type will not alter the original value type.
Solidity has eight value types: signed/unsigned integers, booleans, fixed point numbers, addresses, byte arrays, literals, enums, and contract and function types. Here’s a quick cheatsheet of what they stand for:
Signed/Unsigned integers - Integer data types store whole numbers, with signed integers storing both positive and negative values and unsigned integers storing non-negative values.
Booleans - Boolean data type is declared with the bool keyword, and can hold only two possible constant values, true or false.
Fixed-point numbers - Fixed point numbers represent decimal numbers in Solidity, although they aren’t fully supported by the Ethereum virtual machine yet.
Addresses - The address type is used to store Ethereum wallet or smart contract addresses, typically around 20 bytes. An address type can be suffixed with the keyword “payable”, which restricts it to store only wallet addresses and use the transfer and send crypto functions.
Byte arrays - Byte arrays, declared with the keyword “bytes”, is a fixed-size array used to store a predefined number of bytes up to 32, usually declared along with the keyword (bytes1, bytes2).
Literals - Literals are immutable values such as addresses, rationals and integers, strings, unicode and hexadecimals, which can be stored in a variable.
Enums - Enums, short for Enumerable, are a user-defined data type, that restrict the value of the variable to a particular set of constants defined within the program.
Contract & Function Types - Similar to other object oriented languages, contract and function types are used to represent classes and their functions respectively. Contracts contain functions that can modify the contract’s state variables.
What are reference types?
Solidity reference types, such as fixed and dynamic arrays, are variables that point to the memory address of stored data. Unlike value types, reference types do not store any value but serve as a location tracker of the intended data. Reference types are primarily categorized into four different types, as follows:
Fixed arrays - Fixed arrays are arrays with a pre-defined size at runtime, declared during initialization.
Dynamic arrays - Dynamic arrays are used to allocate size dynamically at runtime depending on data the programs requires it to store.
Structs - Structs in Solidity are a complex data type which allow you to create a custom type containing members of other types, usually used to group linked data.
Mappings - Mappings store data in key-value pairs similar to dictionaries in other object-oriented languages, with the key being a value data type, and value being any type.
What is a signed integer (int)?
A signed integer is a value data type declared with the int keyword to store positive or negative integer values, ranging from -2 to the 255th power and 2 to the 255th power - 1.
Solidity Integer Sizes
The keyword int serves as an abbreviation for int256, an integer data value that can store up to a 256-bit integers or data units. This value data type can also be specified into smaller data values in steps of 8, such as int8, int16, int32, int64, int128 and int256. Developers can use the smaller data sizes such as int8, int16, int32, or int64 if they want to restrict the size of the variable and optimize performance.
What is an unsigned integer (uint)?
An unsigned integer is a value data type declared with the uint keyword which stores a integer value equal to or greater than zero, ranging from 0 to 2to the 255th power - 1.
Uint Data Sizes
Similar to a signed integer, the keyword uint servers an abbreviation for uint256, an unsigned integer data value that can store up to a 256-bit integers or data units.
An unsigned integer value data type can also be specified into smaller data values in steps of 8 from a minimum range of uint8 to maximum range of uint256. The denominations are uint8, uint16, uint32, uint64, uint128 and uint256.
What is the difference between signed and unsigned integers?
The key difference between signed and unsigned integers is that signed integers can store both positive and negative values, while unsigned integers can store only non-negative values. Thus, although both signed and unsigned integers are both value data types, unsigned integers are restricted in the range of values it can store.
In smart contracts, this difference places an emphasis to the developer to prioritize the value that is stored in the data type to avoid an error.
What is the difference between uint and uint256?
Both uint and uint256 share the same definition as they are aliases, but by explicitly stating the size of the integer data size, uint256 adheres to the best practices of creating smart contracts, improving the readability and consistency of the code.
Other than that, there is no difference between the uint and uint256 keywords. The distinction resides on the developer who chooses to use one over another to provide the code with more detail.
Start Using Signed and Unsigned Integers (uint) in Your Smart Contracts
Using the correct Solidity data types is crucial to developing efficient and readable smart contracts. These data types help the compiler understand the amount and type of memory it needs to allocate for specific variables, such as integers or arrays. Allocating space on the blockchain uses gas, so you want to be careful while declaring the size and type of your variables.
If you want to continue learning about Solidity and its features, secure your spot in Alchemy University's 7-week Ethereum Developer Bootcamp.
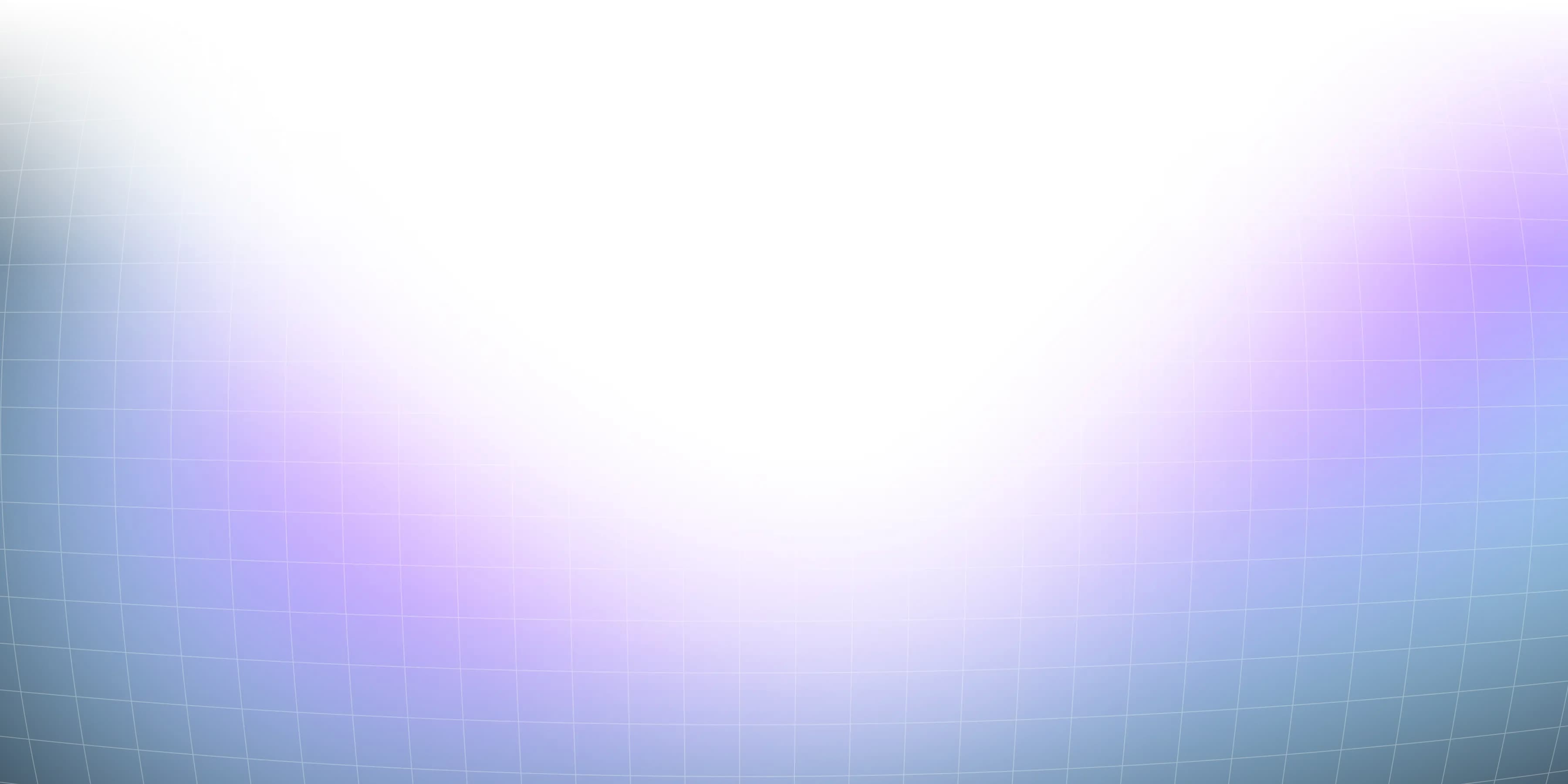
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
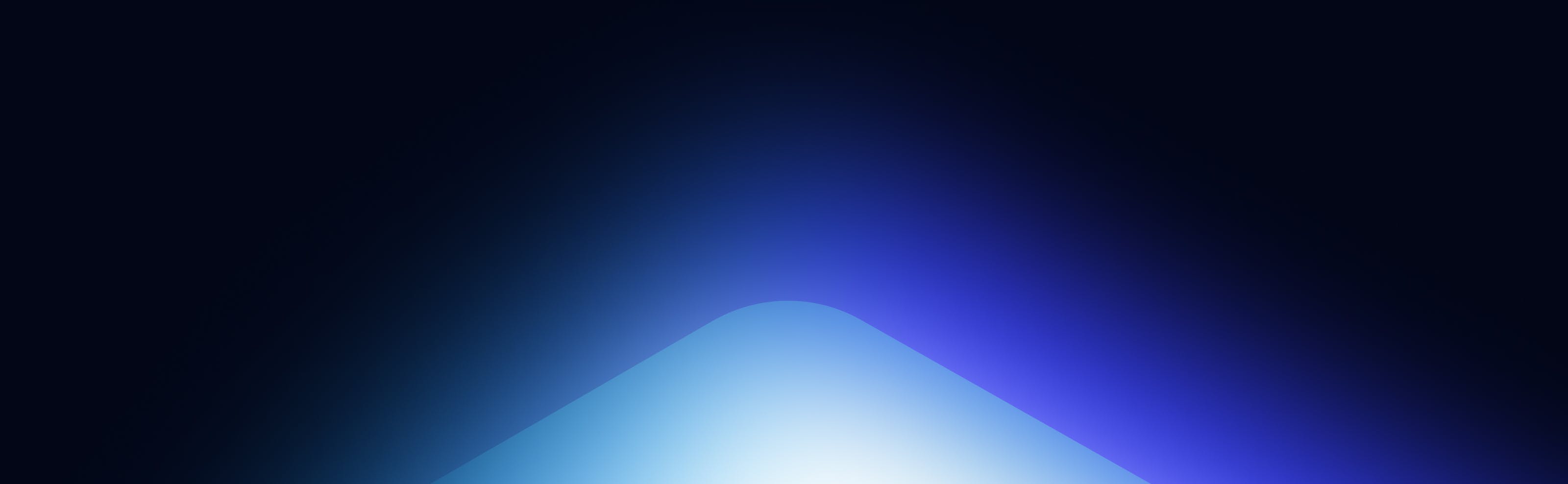