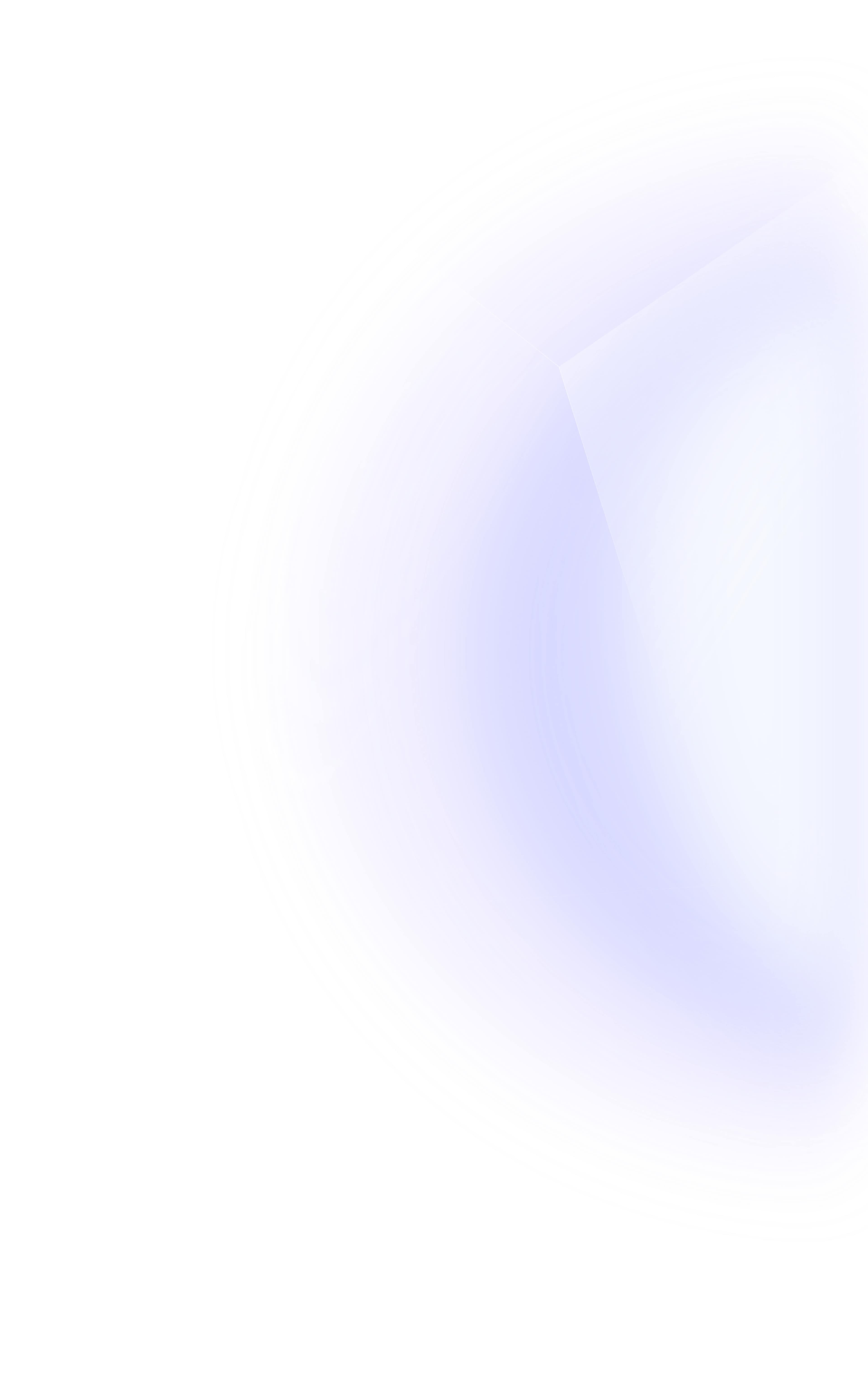
What is a struct in Solidity?
Written by John Favole

Reviewed by Brady Werkheiser
There are different basic data types in Solidity such as uint (unsigned integers), bool, and string, but as a blockchain developer you may need a flexible data type that you can define. A struct is a data structure format in Solidity where variables of diverse data types can be bundled into one variable or a custom-made type.
This article will introduce you to structs in Solidity, demonstrate what they do and how they work. Finally, we will explain how to use structs to create more robust smart contracts.
What is a Solidity struct?
A struct is a creative data structure format in Solidity where variables of diverse data types can be bundled into one variable or a custom-made type. Once the data types are grouped into a struct, the struct name represents the subsets of variables in it.
Imagine structs to be containers that contain different types of objects so when you move the container, all the items within it also move. Therefore, when a Solidity developer declares or calls the name of a struct, the struct responds in line with the data types within it.
The following is an example of a Solidity struct:
contract MyVault{
struct Vault{
address creator;
string name;
address users;
uint amount;
}
}
The struct example above contains variables for address creator, string name, address users, and the uint amount.
Solidity Struct Code Examples
This section will show you sample code for defining and creating structs in Solidity. We’ll also demonstrate the two options available for struct declaration. Finally, we’ll show you how to import and initialize structs.
How to Define and Create a Struct in Solidity
Struct code is similar to how object declaration works in Javascript. While the semblance is striking, the syntaxes work differently. A Solidity struct is always in the following format:
struct name{
string theWord;
uint theFigure;
bool polar;
}
Here is how to define and create a Solidity struct:
Create a struct by writing the struct keyword, which tells the Solidity compiler that the preceding type is a custom type
Name the struct, which will be co-referential to the packed variables in the struct
Use curly brackets, because any other form of brackets such as a box or round brackets won't compile
If you don't use curly brackets, you will generate this ParserError message on Remix.
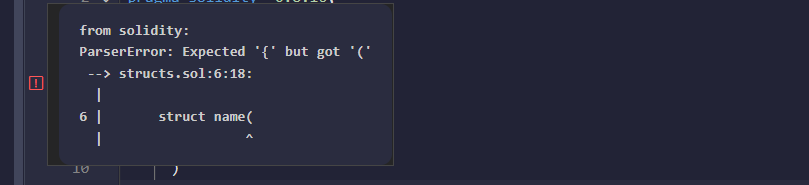
4. Declare your data types along with their corresponding variables
In the above example, these were string theWord, uint theFigure, and bool polarData. This is where you get to declare the subsets of your structs. After the struct is declared in the smart contract, we are able to call the name of the struct later in the code.
Developer Tips:
Only use curly brackets
End each member of a struct with a semicolon
Solidity will throw an error if you declare a data type and input a variable that doesn’t fit its class
Smart contracts can contain multiple structs, which are differentiated by their keyword
How to Declare a Solidity Struct
There are two places where you can declare structs: within a contract or outside a contract. Knowing where to declare your Solidity structs depends on what you want to do. Let us take a look at the two options of struct declaration.
Declare a Struct Outside a Contract
pragma solidity ^0.8.16;
// this is a struct that is declared outside of a contract
struct floorOverlay{
string carpet;
string rug;
}
In the above example, the floorOverlay struct was declared outside the smart contract, and all the contracts in the codebase can call it. It is best to declare a struct outside of a smart contract to create a more applicable struct for all of your contracts in contrast to individual contracts, which may have specific structs just for them.
In addition, if you want smart contracts to tap into the same collection structs, declare it right after pragma instead of creating structs in each of smart contract separately.
Declare a Struct Inside a Contract
The carpet struct is within the smart contract, and as a result, the struct's functionality is restricted to the current contract. No other contract can call its name.
contract CarpetContract{
// this struct is declared within this contract
struct carpet{
string name;
uint length;
uint breadth;
bool shipped;
}
}
How to Import a Solidity Struct
Structs can be imported from one smart contract to another, which helps developers save time and create reusable code. To demonstrate how to import a struct, below are two smart contracts: one where the struct has been created, and another where it will be imported.
pragma solidity ^0.8.16;
// save this contract as “struct” on Remix
contract A{
struct vaccine{
string name;
uint id;
bool vaccinated;
}
}
There is an error you should avoid while importing: the name of the second contract should be the name of the struct you want to import. Otherwise, Solidity compilers would not be able to identify it, resulting in a bug.
pragma solidity ^0.8.16;
import "./struct.sol";
contract Vaccine{
// store it as a state variable
Vaccine public drug;
// go ahead to define more state variables and functions
}
How to Initialize a Struct in Solidity
Even though you have declared and created your struct, you will not be able to use it in various Solidity functions without assigning a certain initial value to it. There are 3 ways you can initialize structs: (i) the key-value pair method, (ii) defining and updating method, (iii) parenthesizing the parameters.
1. The Key Value Pair Method
In this method, you will pick each key type in the struct and assign values to them.
Vaccine memory tetanus = Vaccine({vaccinated: false, name: "Tetanus", id: 3});
The syntax of this method is not strict; you might choose not to follow the order of types in the struct.
2. The Defining and Updating Method
The syntax of this initialization method is such that you have to first input a variable to store the struct, after which you will use the variable to access and name each member of the struct. The following code is an illustration:
Vaccine memory polio
polio.name = "Polio";
polio.id = 3;
polio.vaccinated = true;
However, keep in mind that most developers do not use this method because it is longer.
3. Parenthesizing the Parameters
If you want to use this method, you will have to call the name of the struct, store its memory, and give values to the members of the struct in a serial order. Here is an example:
Vaccine memory measles = Vaccine("Measles", 2, false);
Here, “measles” is the variable storing this initialization, and we also declared the parameters as laid out in the struct. Always remember to put the name of the declaration in the parenthesis within an apostrophe so the Solidity compilers can identify it.
Solidity Struct Use Cases
This section demonstrates a use case of Solidity to track information of users within a contract. Structs are mapped within this use case.
Can structs be mapped in Solidity?
Structs can be mapped in Solidity as value types so you can track pieces of information regarding any member of your struct. Take a look at the following code for instance:
pragma solidity ^0.8.16;
contract MapStructs{
struct DaoMembers{
address owner;
string name;
string guild;
bool voted;
}
mapping(address => DaoMembers) members;
mapping (bool => mapping(address => DaoMembers)) memberStatus;
}
In this contract, the details of each member in a particular DAO are categorized. We created two mappings here: one is simple, while the other is nested.
In the simple mapping, we mapped the address of each member into the struct to make it easier to locate a particular member. In addition, a nested mapping will make it easier to track the addresses of DAO members and whether or not they have voted.
Because mapping is always in the key type => value type syntax, structs must always be declared as a value type, and not a key type. Otherwise, you will receive an error.

Summary — What is the essence of structs in Solidity?
Structs in Solidity allow developers to create custom types that are suited for what they are building. The custom types are similar to containers that hold other related data types within them. To learn more about structs, sign up for Alchemy University's Solidity bootcamp!
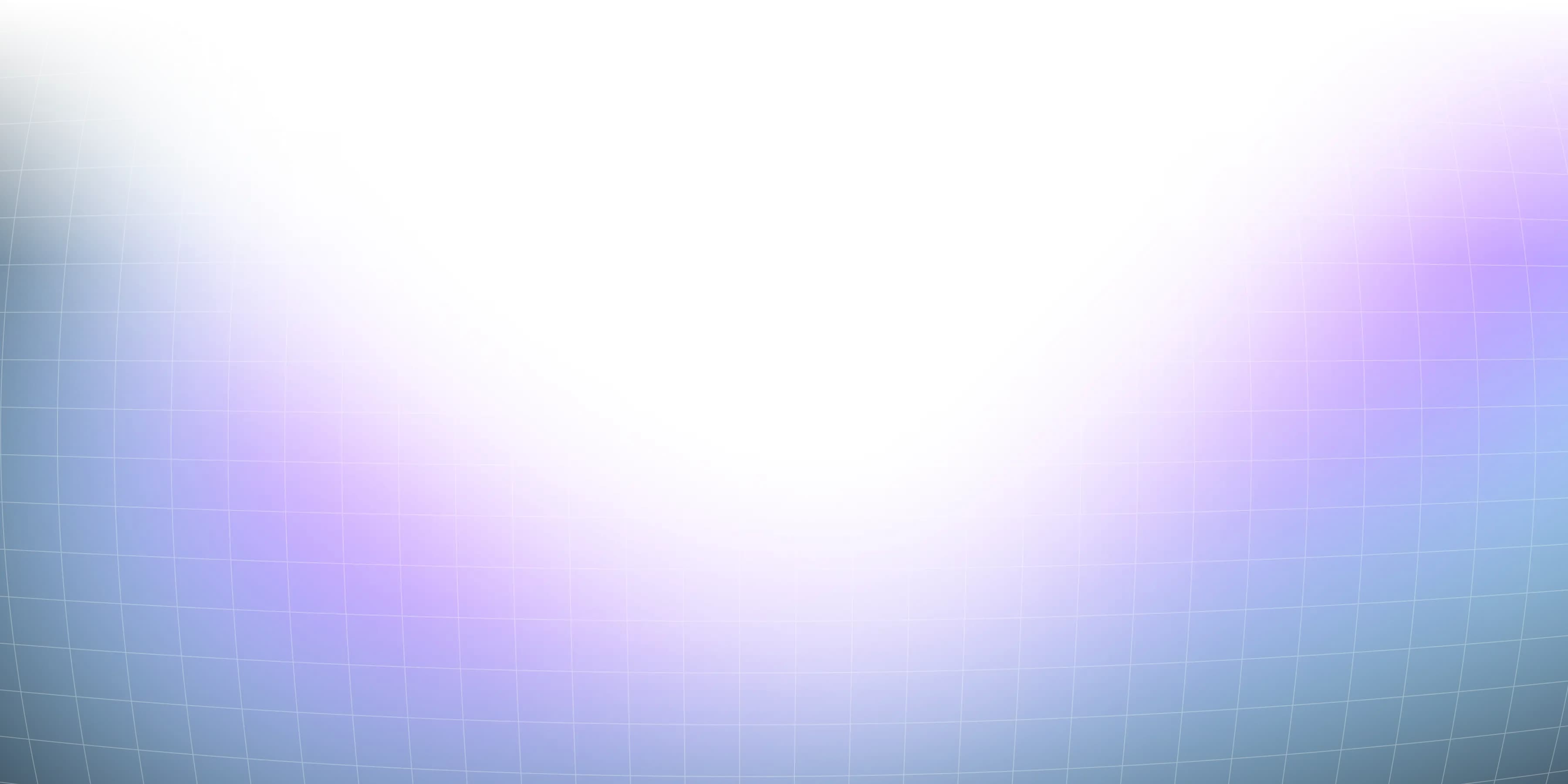
Related overviews
What it is, How it Works, and How to Get Started
Explore the Best Free and Paid Courses for Learning Solidity Development
Your Guide to Getting Started With Solidity Arrays—Functions, Declaring, and Troubleshooting
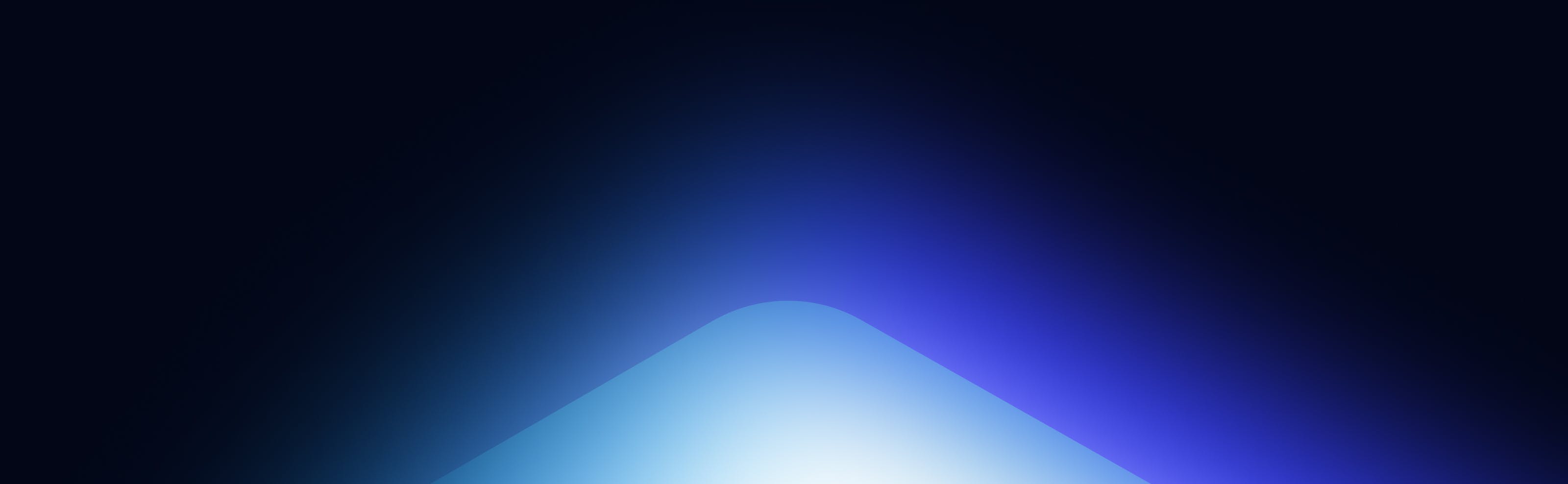