- Verified, Router
- ENS
- Router
The following smart contract is called ETHRegistrarController. It is used to manage the registration and renewal of Ethereum domain names. It uses a BaseRegistrar contract to manage the ownership of domain names and a PriceOracle contract to determine the cost of registration and renewal. The ETHRegistrarController contract allows users to register and renew domain names, set the price oracle, and withdraw funds. It also includes functions for making and committing to a domain name, as well as checking the availability and validity of a domain name.
// File: @ensdomains/ethregistrar/contracts/PriceOracle.sol
pragma solidity >=0.4.24;
interface PriceOracle {
/**
* @dev Returns the price to register or renew a name.
* @param name The name being registered or renewed.
* @param expires When the name presently expires (0 if this is a new registration).
* @param duration How long the name is being registered or extended for, in seconds.
* @return The price of this renewal or registration, in wei.
*/
function price(string calldata name, uint expires, uint duration) external view returns(uint);
}
// File: @ensdomains/ens/contracts/ENS.sol
pragma solidity >=0.4.24;
interface ENS {
// Logged when the owner of a node assigns a new owner to a subnode.
event NewOwner(bytes32 indexed node, bytes32 indexed label, address owner);
// Logged when the owner of a node transfers ownership to a new account.
event Transfer(bytes32 indexed node, address owner);
// Logged when the resolver for a node changes.
event NewResolver(bytes32 indexed node, address resolver);
// Logged when the TTL of a node changes
event NewTTL(bytes32 indexed node, uint64 ttl);
// Logged when an operator is added or removed.
event ApprovalForAll(address indexed owner, address indexed operator, bool approved);
function setRecord(bytes32 node, address owner, address resolver, uint64 ttl) external;
function setSubnodeRecord(bytes32 node, bytes32 label, address owner, address resolver, uint64 ttl) external;
function setSubnodeOwner(bytes32 node, bytes32 label, address owner) external returns(bytes32);
function setResolver(bytes32 node, address resolver) external;
function setOwner(bytes32 node, address owner) external;
function setTTL(bytes32 node, uint64 ttl) external;
function setApprovalForAll(address operator, bool approved) external;
function owner(bytes32 node) external view returns (address);
function resolver(bytes32 node) external view returns (address);
function ttl(bytes32 node) external view returns (uint64);
function recordExists(bytes32 node) external view returns (bool);
function isApprovedForAll(address owner, address operator) external view returns (bool);
}
// File: openzeppelin-solidity/contracts/introspection/IERC165.sol
pragma solidity ^0.5.0;
/**
* @title IERC165
* @dev https://github.com/ethereum/EIPs/blob/master/EIPS/eip-165.md
*/
interface IERC165 {
/**
* @notice Query if a contract implements an interface
* @param interfaceId The interface identifier, as specified in ERC-165
* @dev Interface identification is specified in ERC-165. This function
* uses less than 30,000 gas.
*/
function supportsInterface(bytes4 interfaceId) external view returns (bool);
}
// File: openzeppelin-solidity/contracts/token/ERC721/IERC721.sol
pragma solidity ^0.5.0;
/**
* @title ERC721 Non-Fungible Token Standard basic interface
* @dev see https://github.com/ethereum/EIPs/blob/master/EIPS/eip-721.md
*/
contract IERC721 is IERC165 {
event Transfer(address indexed from, address indexed to, uint256 indexed tokenId);
event Approval(address indexed owner, address indexed approved, uint256 indexed tokenId);
event ApprovalForAll(address indexed owner, address indexed operator, bool approved);
function balanceOf(address owner) public view returns (uint256 balance);
function ownerOf(uint256 tokenId) public view returns (address owner);
function approve(address to, uint256 tokenId) public;
function getApproved(uint256 tokenId) public view returns (address operator);
function setApprovalForAll(address operator, bool _approved) public;
function isApprovedForAll(address owner, address operator) public view returns (bool);
function transferFrom(address from, address to, uint256 tokenId) public;
function safeTransferFrom(address from, address to, uint256 tokenId) public;
function safeTransferFrom(address from, address to, uint256 tokenId, bytes memory data) public;
}
// File: openzeppelin-solidity/contracts/ownership/Ownable.sol
pragma solidity ^0.5.0;
/**
* @title Ownable
* @dev The Ownable contract has an owner address, and provides basic authorization control
* functions, this simplifies the implementation of "user permissions".
*/
contract Ownable {
address private _owner;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
/**
* @dev The Ownable constructor sets the original `owner` of the contract to the sender
* account.
*/
constructor () internal {
_owner = msg.sender;
emit OwnershipTransferred(address(0), _owner);
}
/**
* @return the address of the owner.
*/
function owner() public view returns (address) {
return _owner;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(isOwner());
_;
}
/**
* @return true if `msg.sender` is the owner of the contract.
*/
function isOwner() public view returns (bool) {
return msg.sender == _owner;
}
/**
* @dev Allows the current owner to relinquish control of the contract.
* @notice Renouncing to ownership will leave the contract without an owner.
* It will not be possible to call the functions with the `onlyOwner`
* modifier anymore.
*/
function renounceOwnership() public onlyOwner {
emit OwnershipTransferred(_owner, address(0));
_owner = address(0);
}
/**
* @dev Allows the current owner to transfer control of the contract to a newOwner.
* @param newOwner The address to transfer ownership to.
*/
function transferOwnership(address newOwner) public onlyOwner {
_transferOwnership(newOwner);
}
/**
* @dev Transfers control of the contract to a newOwner.
* @param newOwner The address to transfer ownership to.
*/
function _transferOwnership(address newOwner) internal {
require(newOwner != address(0));
emit OwnershipTransferred(_owner, newOwner);
_owner = newOwner;
}
}
// File: @ensdomains/ethregistrar/contracts/BaseRegistrar.sol
pragma solidity >=0.4.24;
contract BaseRegistrar is IERC721, Ownable {
uint constant public GRACE_PERIOD = 90 days;
event ControllerAdded(address indexed controller);
event ControllerRemoved(address indexed controller);
event NameMigrated(uint256 indexed id, address indexed owner, uint expires);
event NameRegistered(uint256 indexed id, address indexed owner, uint expires);
event NameRenewed(uint256 indexed id, uint expires);
// The ENS registry
ENS public ens;
// The namehash of the TLD this registrar owns (eg, .eth)
bytes32 public baseNode;
// A map of addresses that are authorised to register and renew names.
mapping(address=>bool) public controllers;
// Authorises a controller, who can register and renew domains.
function addController(address controller) external;
// Revoke controller permission for an address.
function removeController(address controller) external;
// Set the resolver for the TLD this registrar manages.
function setResolver(address resolver) external;
// Returns the expiration timestamp of the specified label hash.
function nameExpires(uint256 id) external view returns(uint);
// Returns true iff the specified name is available for registration.
function available(uint256 id) public view returns(bool);
/**
* @dev Register a name.
*/
function register(uint256 id, address owner, uint duration) external returns(uint);
function renew(uint256 id, uint duration) external returns(uint);
/**
* @dev Reclaim ownership of a name in ENS, if you own it in the registrar.
*/
function reclaim(uint256 id, address owner) external;
}
// File: @ensdomains/ethregistrar/contracts/StringUtils.sol
pragma solidity >=0.4.24;
library StringUtils {
/**
* @dev Returns the length of a given string
*
* @param s The string to measure the length of
* @return The length of the input string
*/
function strlen(string memory s) internal pure returns (uint) {
uint len;
uint i = 0;
uint bytelength = bytes(s).length;
for(len = 0; i < bytelength; len++) {
byte b = bytes(s)[i];
if(b < 0x80) {
i += 1;
} else if (b < 0xE0) {
i += 2;
} else if (b < 0xF0) {
i += 3;
} else if (b < 0xF8) {
i += 4;
} else if (b < 0xFC) {
i += 5;
} else {
i += 6;
}
}
return len;
}
}
// File: @ensdomains/resolver/contracts/Resolver.sol
pragma solidity >=0.4.25;
/**
* A generic resolver interface which includes all the functions including the ones deprecated
*/
interface Resolver{
event AddrChanged(bytes32 indexed node, address a);
event AddressChanged(bytes32 indexed node, uint coinType, bytes newAddress);
event NameChanged(bytes32 indexed node, string name);
event ABIChanged(bytes32 indexed node, uint256 indexed contentType);
event PubkeyChanged(bytes32 indexed node, bytes32 x, bytes32 y);
event TextChanged(bytes32 indexed node, string indexed indexedKey, string key);
event ContenthashChanged(bytes32 indexed node, bytes hash);
/* Deprecated events */
event ContentChanged(bytes32 indexed node, bytes32 hash);
function ABI(bytes32 node, uint256 contentTypes) external view returns (uint256, bytes memory);
function addr(bytes32 node) external view returns (address);
function addr(bytes32 node, uint coinType) external view returns(bytes memory);
function contenthash(bytes32 node) external view returns (bytes memory);
function dnsrr(bytes32 node) external view returns (bytes memory);
function name(bytes32 node) external view returns (string memory);
function pubkey(bytes32 node) external view returns (bytes32 x, bytes32 y);
function text(bytes32 node, string calldata key) external view returns (string memory);
function interfaceImplementer(bytes32 node, bytes4 interfaceID) external view returns (address);
function setABI(bytes32 node, uint256 contentType, bytes calldata data) external;
function setAddr(bytes32 node, address addr) external;
function setAddr(bytes32 node, uint coinType, bytes calldata a) external;
function setContenthash(bytes32 node, bytes calldata hash) external;
function setDnsrr(bytes32 node, bytes calldata data) external;
function setName(bytes32 node, string calldata _name) external;
function setPubkey(bytes32 node, bytes32 x, bytes32 y) external;
function setText(bytes32 node, string calldata key, string calldata value) external;
function setInterface(bytes32 node, bytes4 interfaceID, address implementer) external;
function supportsInterface(bytes4 interfaceID) external pure returns (bool);
/* Deprecated functions */
function content(bytes32 node) external view returns (bytes32);
function multihash(bytes32 node) external view returns (bytes memory);
function setContent(bytes32 node, bytes32 hash) external;
function setMultihash(bytes32 node, bytes calldata hash) external;
}
// File: @ensdomains/ethregistrar/contracts/ETHRegistrarController.sol
pragma solidity ^0.5.0;
/**
* @dev A registrar controller for registering and renewing names at fixed cost.
*/
contract ETHRegistrarController is Ownable {
using StringUtils for *;
uint constant public MIN_REGISTRATION_DURATION = 28 days;
bytes4 constant private INTERFACE_META_ID = bytes4(keccak256("supportsInterface(bytes4)"));
bytes4 constant private COMMITMENT_CONTROLLER_ID = bytes4(
keccak256("rentPrice(string,uint256)") ^
keccak256("available(string)") ^
keccak256("makeCommitment(string,address,bytes32)") ^
keccak256("commit(bytes32)") ^
keccak256("register(string,address,uint256,bytes32)") ^
keccak256("renew(string,uint256)")
);
bytes4 constant private COMMITMENT_WITH_CONFIG_CONTROLLER_ID = bytes4(
keccak256("registerWithConfig(string,address,uint256,bytes32,address,address)") ^
keccak256("makeCommitmentWithConfig(string,address,bytes32,address,address)")
);
BaseRegistrar base;
PriceOracle prices;
uint public minCommitmentAge;
uint public maxCommitmentAge;
mapping(bytes32=>uint) public commitments;
event NameRegistered(string name, bytes32 indexed label, address indexed owner, uint cost, uint expires);
event NameRenewed(string name, bytes32 indexed label, uint cost, uint expires);
event NewPriceOracle(address indexed oracle);
constructor(BaseRegistrar _base, PriceOracle _prices, uint _minCommitmentAge, uint _maxCommitmentAge) public {
require(_maxCommitmentAge > _minCommitmentAge);
base = _base;
prices = _prices;
minCommitmentAge = _minCommitmentAge;
maxCommitmentAge = _maxCommitmentAge;
}
function rentPrice(string memory name, uint duration) view public returns(uint) {
bytes32 hash = keccak256(bytes(name));
return prices.price(name, base.nameExpires(uint256(hash)), duration);
}
function valid(string memory name) public pure returns(bool) {
return name.strlen() >= 3;
}
function available(string memory name) public view returns(bool) {
bytes32 label = keccak256(bytes(name));
return valid(name) && base.available(uint256(label));
}
function makeCommitment(string memory name, address owner, bytes32 secret) pure public returns(bytes32) {
return makeCommitmentWithConfig(name, owner, secret, address(0), address(0));
}
function makeCommitmentWithConfig(string memory name, address owner, bytes32 secret, address resolver, address addr) pure public returns(bytes32) {
bytes32 label = keccak256(bytes(name));
if (resolver == address(0) && addr == address(0)) {
return keccak256(abi.encodePacked(label, owner, secret));
}
require(resolver != address(0));
return keccak256(abi.encodePacked(label, owner, resolver, addr, secret));
}
function commit(bytes32 commitment) public {
require(commitments[commitment] + maxCommitmentAge < now);
commitments[commitment] = now;
}
function register(string calldata name, address owner, uint duration, bytes32 secret) external payable {
registerWithConfig(name, owner, duration, secret, address(0), address(0));
}
function registerWithConfig(string memory name, address owner, uint duration, bytes32 secret, address resolver, address addr) public payable {
bytes32 commitment = makeCommitmentWithConfig(name, owner, secret, resolver, addr);
uint cost = _consumeCommitment(name, duration, commitment);
bytes32 label = keccak256(bytes(name));
uint256 tokenId = uint256(label);
uint expires;
if(resolver != address(0)) {
// Set this contract as the (temporary) owner, giving it
// permission to set up the resolver.
expires = base.register(tokenId, address(this), duration);
// The nodehash of this label
bytes32 nodehash = keccak256(abi.encodePacked(base.baseNode(), label));
// Set the resolver
base.ens().setResolver(nodehash, resolver);
// Configure the resolver
if (addr != address(0)) {
Resolver(resolver).setAddr(nodehash, addr);
}
// Now transfer full ownership to the expeceted owner
base.reclaim(tokenId, owner);
base.transferFrom(address(this), owner, tokenId);
} else {
require(addr == address(0));
expires = base.register(tokenId, owner, duration);
}
emit NameRegistered(name, label, owner, cost, expires);
// Refund any extra payment
if(msg.value > cost) {
msg.sender.transfer(msg.value - cost);
}
}
function renew(string calldata name, uint duration) external payable {
uint cost = rentPrice(name, duration);
require(msg.value >= cost);
bytes32 label = keccak256(bytes(name));
uint expires = base.renew(uint256(label), duration);
if(msg.value > cost) {
msg.sender.transfer(msg.value - cost);
}
emit NameRenewed(name, label, cost, expires);
}
function setPriceOracle(PriceOracle _prices) public onlyOwner {
prices = _prices;
emit NewPriceOracle(address(prices));
}
function setCommitmentAges(uint _minCommitmentAge, uint _maxCommitmentAge) public onlyOwner {
minCommitmentAge = _minCommitmentAge;
maxCommitmentAge = _maxCommitmentAge;
}
function withdraw() public onlyOwner {
msg.sender.transfer(address(this).balance);
}
function supportsInterface(bytes4 interfaceID) external pure returns (bool) {
return interfaceID == INTERFACE_META_ID ||
interfaceID == COMMITMENT_CONTROLLER_ID ||
interfaceID == COMMITMENT_WITH_CONFIG_CONTROLLER_ID;
}
function _consumeCommitment(string memory name, uint duration, bytes32 commitment) internal returns (uint256) {
// Require a valid commitment
require(commitments[commitment] + minCommitmentAge <= now);
// If the commitment is too old, or the name is registered, stop
require(commitments[commitment] + maxCommitmentAge > now);
require(available(name));
delete(commitments[commitment]);
uint cost = rentPrice(name, duration);
require(duration >= MIN_REGISTRATION_DURATION);
require(msg.value >= cost);
return cost;
}
}
[{"inputs":[{"internalType":"contract BaseRegistrar","name":"_base","type":"address"},{"internalType":"contract PriceOracle","name":"_prices","type":"address"},{"internalType":"uint256","name":"_minCommitmentAge","type":"uint256"},{"internalType":"uint256","name":"_maxCommitmentAge","type":"uint256"}],"payable":false,"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"string","name":"name","type":"string"},{"indexed":true,"internalType":"bytes32","name":"label","type":"bytes32"},{"indexed":true,"internalType":"address","name":"owner","type":"address"},{"indexed":false,"internalType":"uint256","name":"cost","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"expires","type":"uint256"}],"name":"NameRegistered","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"string","name":"name","type":"string"},{"indexed":true,"internalType":"bytes32","name":"label","type":"bytes32"},{"indexed":false,"internalType":"uint256","name":"cost","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"expires","type":"uint256"}],"name":"NameRenewed","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"oracle","type":"address"}],"name":"NewPriceOracle","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"previousOwner","type":"address"},{"indexed":true,"internalType":"address","name":"newOwner","type":"address"}],"name":"OwnershipTransferred","type":"event"},{"constant":true,"inputs":[],"name":"MIN_REGISTRATION_DURATION","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[{"internalType":"string","name":"name","type":"string"}],"name":"available","outputs":[{"internalType":"bool","name":"","type":"bool"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"internalType":"bytes32","name":"commitment","type":"bytes32"}],"name":"commit","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"internalType":"bytes32","name":"","type":"bytes32"}],"name":"commitments","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[],"name":"isOwner","outputs":[{"internalType":"bool","name":"","type":"bool"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"address","name":"owner","type":"address"},{"internalType":"bytes32","name":"secret","type":"bytes32"}],"name":"makeCommitment","outputs":[{"internalType":"bytes32","name":"","type":"bytes32"}],"payable":false,"stateMutability":"pure","type":"function"},{"constant":true,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"address","name":"owner","type":"address"},{"internalType":"bytes32","name":"secret","type":"bytes32"},{"internalType":"address","name":"resolver","type":"address"},{"internalType":"address","name":"addr","type":"address"}],"name":"makeCommitmentWithConfig","outputs":[{"internalType":"bytes32","name":"","type":"bytes32"}],"payable":false,"stateMutability":"pure","type":"function"},{"constant":true,"inputs":[],"name":"maxCommitmentAge","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[],"name":"minCommitmentAge","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[],"name":"owner","outputs":[{"internalType":"address","name":"","type":"address"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"address","name":"owner","type":"address"},{"internalType":"uint256","name":"duration","type":"uint256"},{"internalType":"bytes32","name":"secret","type":"bytes32"}],"name":"register","outputs":[],"payable":true,"stateMutability":"payable","type":"function"},{"constant":false,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"address","name":"owner","type":"address"},{"internalType":"uint256","name":"duration","type":"uint256"},{"internalType":"bytes32","name":"secret","type":"bytes32"},{"internalType":"address","name":"resolver","type":"address"},{"internalType":"address","name":"addr","type":"address"}],"name":"registerWithConfig","outputs":[],"payable":true,"stateMutability":"payable","type":"function"},{"constant":false,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"uint256","name":"duration","type":"uint256"}],"name":"renew","outputs":[],"payable":true,"stateMutability":"payable","type":"function"},{"constant":false,"inputs":[],"name":"renounceOwnership","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"internalType":"string","name":"name","type":"string"},{"internalType":"uint256","name":"duration","type":"uint256"}],"name":"rentPrice","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"internalType":"uint256","name":"_minCommitmentAge","type":"uint256"},{"internalType":"uint256","name":"_maxCommitmentAge","type":"uint256"}],"name":"setCommitmentAges","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":false,"inputs":[{"internalType":"contract PriceOracle","name":"_prices","type":"address"}],"name":"setPriceOracle","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"internalType":"bytes4","name":"interfaceID","type":"bytes4"}],"name":"supportsInterface","outputs":[{"internalType":"bool","name":"","type":"bool"}],"payable":false,"stateMutability":"pure","type":"function"},{"constant":false,"inputs":[{"internalType":"address","name":"newOwner","type":"address"}],"name":"transferOwnership","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"internalType":"string","name":"name","type":"string"}],"name":"valid","outputs":[{"internalType":"bool","name":"","type":"bool"}],"payable":false,"stateMutability":"pure","type":"function"},{"constant":false,"inputs":[],"name":"withdraw","outputs":[],"payable":false,"stateMutability":"nonpayable","type":"function"}]
608060405234801561001057600080fd5b5060405161267f38038061267f8339818101604052608081101561003357600080fd5b8101908080519060200190929190805190602001909291908051906020019092919080519060200190929190505050336000806101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff1602179055506000809054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16600073ffffffffffffffffffffffffffffffffffffffff167f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e060405160405180910390a381811161012a57600080fd5b83600160006101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff16021790555082600260006101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff1602179055508160038190555080600481905550505050506124b2806101cd6000396000f3fe60806040526004361061012a5760003560e01c80638d839ffe116100ab578063aeb8ce9b1161006f578063aeb8ce9b146107cc578063ce1e09c0146108ac578063f14fcbc8146108d7578063f2fde38b14610912578063f49826be14610963578063f7a1696314610a695761012a565b80638d839ffe146105b85780638da5cb5b146105e35780638f32d59b1461063a5780639791c09714610669578063acf1a841146107495761012a565b80637e324479116100f25780637e32447914610366578063839df945146103ab57806383e7f6ff146103fa57806385f6d155146104e05780638a95b09f1461058d5761012a565b806301ffc9a71461012f5780633ccfd60b146101a15780633d86c52f146101b8578063530e784f146102fe578063715018a61461034f575b600080fd5b34801561013b57600080fd5b506101876004803603602081101561015257600080fd5b8101908080357bffffffffffffffffffffffffffffffffffffffffffffffffffffffff19169060200190929190505050610b98565b604051808215151515815260200191505060405180910390f35b3480156101ad57600080fd5b506101b6610de7565b005b3480156101c457600080fd5b506102e8600480360360a08110156101db57600080fd5b81019080803590602001906401000000008111156101f857600080fd5b82018360208201111561020a57600080fd5b8035906020019184600183028401116401000000008311171561022c57600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f820116905080830192505050505050509192919290803573ffffffffffffffffffffffffffffffffffffffff16906020019092919080359060200190929190803573ffffffffffffffffffffffffffffffffffffffff169060200190929190803573ffffffffffffffffffffffffffffffffffffffff169060200190929190505050610e41565b6040518082815260200191505060405180910390f35b34801561030a57600080fd5b5061034d6004803603602081101561032157600080fd5b81019080803573ffffffffffffffffffffffffffffffffffffffff169060200190929190505050611044565b005b34801561035b57600080fd5b506103646110fe565b005b34801561037257600080fd5b506103a96004803603604081101561038957600080fd5b8101908080359060200190929190803590602001909291905050506111ce565b005b3480156103b757600080fd5b506103e4600480360360208110156103ce57600080fd5b81019080803590602001909291905050506111f1565b6040518082815260200191505060405180910390f35b34801561040657600080fd5b506104ca6004803603604081101561041d57600080fd5b810190808035906020019064010000000081111561043a57600080fd5b82018360208201111561044c57600080fd5b8035906020019184600183028401116401000000008311171561046e57600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f82011690508083019250505050505050919291929080359060200190929190505050611209565b6040518082815260200191505060405180910390f35b61058b600480360360808110156104f657600080fd5b810190808035906020019064010000000081111561051357600080fd5b82018360208201111561052557600080fd5b8035906020019184600183028401116401000000008311171561054757600080fd5b9091929391929390803573ffffffffffffffffffffffffffffffffffffffff16906020019092919080359060200190929190803590602001909291905050506113f2565b005b34801561059957600080fd5b506105a261144c565b6040518082815260200191505060405180910390f35b3480156105c457600080fd5b506105cd611453565b6040518082815260200191505060405180910390f35b3480156105ef57600080fd5b506105f8611459565b604051808273ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200191505060405180910390f35b34801561064657600080fd5b5061064f611482565b604051808215151515815260200191505060405180910390f35b34801561067557600080fd5b5061072f6004803603602081101561068c57600080fd5b81019080803590602001906401000000008111156106a957600080fd5b8201836020820111156106bb57600080fd5b803590602001918460018302840111640100000000831117156106dd57600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f8201169050808301925050505050505091929192905050506114d9565b604051808215151515815260200191505060405180910390f35b6107ca6004803603604081101561075f57600080fd5b810190808035906020019064010000000081111561077c57600080fd5b82018360208201111561078e57600080fd5b803590602001918460018302840111640100000000831117156107b057600080fd5b9091929391929390803590602001909291905050506114ef565b005b3480156107d857600080fd5b50610892600480360360208110156107ef57600080fd5b810190808035906020019064010000000081111561080c57600080fd5b82018360208201111561081e57600080fd5b8035906020019184600183028401116401000000008311171561084057600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f8201169050808301925050505050505091929192905050506116fe565b604051808215151515815260200191505060405180910390f35b3480156108b857600080fd5b506108c16117d5565b6040518082815260200191505060405180910390f35b3480156108e357600080fd5b50610910600480360360208110156108fa57600080fd5b81019080803590602001909291905050506117db565b005b34801561091e57600080fd5b506109616004803603602081101561093557600080fd5b81019080803573ffffffffffffffffffffffffffffffffffffffff169060200190929190505050611819565b005b34801561096f57600080fd5b50610a536004803603606081101561098657600080fd5b81019080803590602001906401000000008111156109a357600080fd5b8201836020820111156109b557600080fd5b803590602001918460018302840111640100000000831117156109d757600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f820116905080830192505050505050509192919290803573ffffffffffffffffffffffffffffffffffffffff16906020019092919080359060200190929190505050611836565b6040518082815260200191505060405180910390f35b610b96600480360360c0811015610a7f57600080fd5b8101908080359060200190640100000000811115610a9c57600080fd5b820183602082011115610aae57600080fd5b80359060200191846001830284011164010000000083111715610ad057600080fd5b91908080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f820116905080830192505050505050509192919290803573ffffffffffffffffffffffffffffffffffffffff1690602001909291908035906020019092919080359060200190929190803573ffffffffffffffffffffffffffffffffffffffff169060200190929190803573ffffffffffffffffffffffffffffffffffffffff16906020019092919050505061184f565b005b600060405180807f737570706f727473496e74657266616365286279746573342900000000000000815250601901905060405180910390207bffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916827bffffffffffffffffffffffffffffffffffffffffffffffffffffffff19161480610d66575060405180807f72656e657728737472696e672c75696e743235362900000000000000000000008152506015019050604051809103902060405180806123ee602891396028019050604051809103902060405180807f636f6d6d69742862797465733332290000000000000000000000000000000000815250600f01905060405180910390206040518080612416602691396026019050604051809103902060405180807f617661696c61626c6528737472696e67290000000000000000000000000000008152506011019050604051809103902060405180807f72656e74507269636528737472696e672c75696e7432353629000000000000008152506019019050604051809103902018181818187bffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916827bffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916145b80610de0575060405180806123ae6040913960400190506040518091039020604051808061243c6042913960420190506040518091039020187bffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916827bffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916145b9050919050565b610def611482565b610df857600080fd5b3373ffffffffffffffffffffffffffffffffffffffff166108fc479081150290604051600060405180830381858888f19350505050158015610e3e573d6000803e3d6000fd5b50565b60008086805190602001209050600073ffffffffffffffffffffffffffffffffffffffff168473ffffffffffffffffffffffffffffffffffffffff16148015610eb65750600073ffffffffffffffffffffffffffffffffffffffff168373ffffffffffffffffffffffffffffffffffffffff16145b15610f2957808686604051602001808481526020018373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1660601b815260140182815260200193505050506040516020818303038152906040528051906020012091505061103b565b600073ffffffffffffffffffffffffffffffffffffffff168473ffffffffffffffffffffffffffffffffffffffff161415610f6357600080fd5b8086858588604051602001808681526020018573ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1660601b81526014018473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1660601b81526014018373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1660601b815260140182815260200195505050505050604051602081830303815290604052805190602001209150505b95945050505050565b61104c611482565b61105557600080fd5b80600260006101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff160217905550600260009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff167ff261845a790fe29bbd6631e2ca4a5bdc83e6eed7c3271d9590d97287e00e912360405160405180910390a250565b611106611482565b61110f57600080fd5b600073ffffffffffffffffffffffffffffffffffffffff166000809054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff167f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e060405160405180910390a360008060006101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff160217905550565b6111d6611482565b6111df57600080fd5b81600381905550806004819055505050565b60056020528060005260406000206000915090505481565b60008083805190602001209050600260009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff166350e9a71585600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1663d6e4fa868560001c6040518263ffffffff1660e01b81526004018082815260200191505060206040518083038186803b1580156112cb57600080fd5b505afa1580156112df573d6000803e3d6000fd5b505050506040513d60208110156112f557600080fd5b8101908080519060200190929190505050866040518463ffffffff1660e01b81526004018080602001848152602001838152602001828103825285818151815260200191508051906020019080838360005b83811015611362578082015181840152602081019050611347565b50505050905090810190601f16801561138f5780820380516001836020036101000a031916815260200191505b5094505050505060206040518083038186803b1580156113ae57600080fd5b505afa1580156113c2573d6000803e3d6000fd5b505050506040513d60208110156113d857600080fd5b810190808051906020019092919050505091505092915050565b61144585858080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f8201169050808301925050505050505084848460008061184f565b5050505050565b6224ea0081565b60035481565b60008060009054906101000a900473ffffffffffffffffffffffffffffffffffffffff16905090565b60008060009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff163373ffffffffffffffffffffffffffffffffffffffff1614905090565b600060036114e683612097565b10159050919050565b600061153f84848080601f016020809104026020016040519081016040528093929190818152602001838380828437600081840152601f19601f8201169050808301925050505050505083611209565b90508034101561154e57600080fd5b60008484604051808383808284378083019250505092505050604051809103902090506000600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1663c475abff8360001c866040518363ffffffff1660e01b81526004018083815260200182815260200192505050602060405180830381600087803b1580156115f357600080fd5b505af1158015611607573d6000803e3d6000fd5b505050506040513d602081101561161d57600080fd5b8101908080519060200190929190505050905082341115611682573373ffffffffffffffffffffffffffffffffffffffff166108fc8434039081150290604051600060405180830381858888f19350505050158015611680573d6000803e3d6000fd5b505b817f3da24c024582931cfaf8267d8ed24d13a82a8068d5bd337d30ec45cea4e506ae8787868560405180806020018481526020018381526020018281038252868682818152602001925080828437600081840152601f19601f8201169050808301925050509550505050505060405180910390a2505050505050565b60008082805190602001209050611714836114d9565b80156117cd5750600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff166396e494e88260001c6040518263ffffffff1660e01b81526004018082815260200191505060206040518083038186803b15801561179157600080fd5b505afa1580156117a5573d6000803e3d6000fd5b505050506040513d60208110156117bb57600080fd5b81019080805190602001909291905050505b915050919050565b60045481565b42600454600560008481526020019081526020016000205401106117fe57600080fd5b42600560008381526020019081526020016000208190555050565b611821611482565b61182a57600080fd5b6118338161220d565b50565b6000611846848484600080610e41565b90509392505050565b600061185e8787868686610e41565b9050600061186d888784612305565b905060008880519060200120905060008160001c905060008073ffffffffffffffffffffffffffffffffffffffff168773ffffffffffffffffffffffffffffffffffffffff1614611e4c57600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1663fca247ac83308c6040518463ffffffff1660e01b8152600401808481526020018373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020018281526020019350505050602060405180830381600087803b15801561196957600080fd5b505af115801561197d573d6000803e3d6000fd5b505050506040513d602081101561199357600080fd5b810190808051906020019092919050505090506000600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1663ddf7fcb06040518163ffffffff1660e01b815260040160206040518083038186803b158015611a1057600080fd5b505afa158015611a24573d6000803e3d6000fd5b505050506040513d6020811015611a3a57600080fd5b8101908080519060200190929190505050846040516020018083815260200182815260200192505050604051602081830303815290604052805190602001209050600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16633f15457f6040518163ffffffff1660e01b815260040160206040518083038186803b158015611ae357600080fd5b505afa158015611af7573d6000803e3d6000fd5b505050506040513d6020811015611b0d57600080fd5b810190808051906020019092919050505073ffffffffffffffffffffffffffffffffffffffff16631896f70a828a6040518363ffffffff1660e01b8152600401808381526020018273ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200192505050600060405180830381600087803b158015611ba457600080fd5b505af1158015611bb8573d6000803e3d6000fd5b50505050600073ffffffffffffffffffffffffffffffffffffffff168773ffffffffffffffffffffffffffffffffffffffff1614611c90578773ffffffffffffffffffffffffffffffffffffffff1663d5fa2b0082896040518363ffffffff1660e01b8152600401808381526020018273ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200192505050600060405180830381600087803b158015611c7757600080fd5b505af1158015611c8b573d6000803e3d6000fd5b505050505b600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff166328ed4f6c848d6040518363ffffffff1660e01b8152600401808381526020018273ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200192505050600060405180830381600087803b158015611d3957600080fd5b505af1158015611d4d573d6000803e3d6000fd5b50505050600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff166323b872dd308d866040518463ffffffff1660e01b8152600401808473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020018373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020018281526020019350505050600060405180830381600087803b158015611e2e57600080fd5b505af1158015611e42573d6000803e3d6000fd5b5050505050611f74565b600073ffffffffffffffffffffffffffffffffffffffff168673ffffffffffffffffffffffffffffffffffffffff1614611e8557600080fd5b600160009054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1663fca247ac838c8c6040518463ffffffff1660e01b8152600401808481526020018373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020018281526020019350505050602060405180830381600087803b158015611f3657600080fd5b505af1158015611f4a573d6000803e3d6000fd5b505050506040513d6020811015611f6057600080fd5b810190808051906020019092919050505090505b8973ffffffffffffffffffffffffffffffffffffffff16837fca6abbe9d7f11422cb6ca7629fbf6fe9efb1c621f71ce8f02b9f2a230097404f8d87856040518080602001848152602001838152602001828103825285818151815260200191508051906020019080838360005b83811015611ffc578082015181840152602081019050611fe1565b50505050905090810190601f1680156120295780820380516001836020036101000a031916815260200191505b5094505050505060405180910390a38334111561208a573373ffffffffffffffffffffffffffffffffffffffff166108fc8534039081150290604051600060405180830381858888f19350505050158015612088573d6000803e3d6000fd5b505b5050505050505050505050565b6000806000809050600084519050600092505b808210156122025760008583815181106120c057fe5b602001015160f81c60f81b9050608060f81b817effffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff19161015612106576001830192506121f4565b60e060f81b817effffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff1916101561213f576002830192506121f3565b60f060f81b817effffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff19161015612178576003830192506121f2565b60f8801b817effffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff191610156121b0576004830192506121f1565b60fc60f81b817effffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff191610156121e9576005830192506121f0565b6006830192505b5b5b5b5b5082806001019350506120aa565b829350505050919050565b600073ffffffffffffffffffffffffffffffffffffffff168173ffffffffffffffffffffffffffffffffffffffff16141561224757600080fd5b8073ffffffffffffffffffffffffffffffffffffffff166000809054906101000a900473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff167f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e060405160405180910390a3806000806101000a81548173ffffffffffffffffffffffffffffffffffffffff021916908373ffffffffffffffffffffffffffffffffffffffff16021790555050565b600042600354600560008581526020019081526020016000205401111561232b57600080fd5b426004546005600085815260200190815260200160002054011161234e57600080fd5b612357846116fe565b61236057600080fd5b600560008381526020019081526020016000206000905560006123838585611209565b90506224ea0084101561239557600080fd5b803410156123a257600080fd5b80915050939250505056fe6d616b65436f6d6d69746d656e7457697468436f6e66696728737472696e672c616464726573732c627974657333322c616464726573732c6164647265737329726567697374657228737472696e672c616464726573732c75696e743235362c62797465733332296d616b65436f6d6d69746d656e7428737472696e672c616464726573732c6279746573333229726567697374657257697468436f6e66696728737472696e672c616464726573732c75696e743235362c627974657333322c616464726573732c6164647265737329a265627a7a72315820ae5aec11a7c9126de666c7630771a2e6e95d684de2539cfca1b14cb30a427a4264736f6c6343000510003200000000000000000000000057f1887a8bf19b14fc0df6fd9b2acc9af147ea85000000000000000000000000b9d374d0fe3d8341155663fae31b7beae0ae233a000000000000000000000000000000000000000000000000000000000000003c0000000000000000000000000000000000000000000000000000000000015180
Checkout more smart contracts
- Lido
- Proposer Fee Recipient
- Router
- Verified, Router
- Router
- Verified, Router
- Router

LidoExecutionLayerRewardsVault
The following smart contract is called LidoExecutionLayerRewardsVault. It is used to manage rewards for the Lido protocol. The contract allows Lido to withdraw rewards, recover ERC20 and ERC721 tokens, and receive ETH. The contract uses the SafeERC20 library to ensure safe transfers of ERC20 tokens. The purpose of this contract is to provide a secure and efficient way to manage rewards for the Lido protocol.

Registry
The following smart contract is a Registry contract that manages routes for cross-chain transfers. It allows adding, disabling, and executing routes for middleware and bridge contracts. It also includes a function for rescuing funds and uses OpenZeppelin libraries for access control and ERC20 token handling.

GasRefunder
The following smart contract is a GasRefunder contract that refunds gas costs to specified refundees. It allows the owner to set common parameters such as maximum refundee balance, extra gas margin, calldata cost, maximum gas tip, maximum gas cost, and maximum single gas usage. The contract also allows the owner to set allowed contracts and refundees, and withdraw funds.
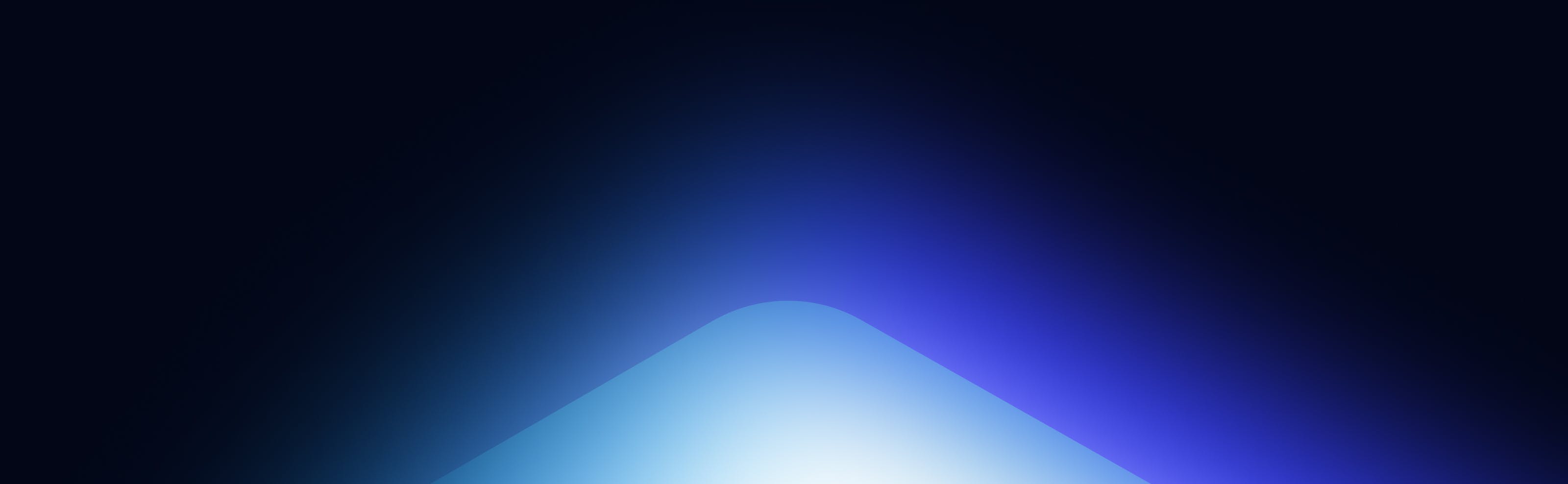