- Verified, Router
- Router
The following smart contract is a GasRefunder contract that refunds gas costs to specified refundees. It allows the owner to set common parameters such as maximum refundee balance, extra gas margin, calldata cost, maximum gas tip, maximum gas cost, and maximum single gas usage. The contract also allows the owner to set allowed contracts and refundees, and withdraw funds.
// SPDX-License-Identifier: Apache-2.0
/*
* Copyright 2021, Offchain Labs, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
pragma solidity ^0.8.7;
import "./IGasRefunder.sol";
import "@openzeppelin/contracts-0.8/access/Ownable.sol";
contract GasRefunder is IGasRefunder, Ownable {
mapping(address => bool) public allowedContracts;
mapping(address => bool) public allowedRefundees;
address public disallower;
struct CommonParameters {
uint128 maxRefundeeBalance;
uint32 extraGasMargin;
uint8 calldataCost;
uint64 maxGasTip;
uint64 maxGasCost;
uint32 maxSingleGasUsage;
}
CommonParameters public commonParams;
enum CommonParameterKey {
MAX_REFUNDEE_BALANCE,
EXTRA_GAS_MARGIN,
CALLDATA_COST,
MAX_GAS_TIP,
MAX_GAS_COST,
MAX_SINGLE_GAS_USAGE
}
enum RefundDenyReason {
CONTRACT_NOT_ALLOWED,
REFUNDEE_NOT_ALLOWED,
REFUNDEE_ABOVE_MAX_BALANCE,
OUT_OF_FUNDS
}
event RefundedGasCosts(
address indexed refundee,
address indexed contractAddress,
bool indexed success,
uint256 gas,
uint256 gasPrice,
uint256 amountPaid
);
event RefundGasCostsDenied(
address indexed refundee,
address indexed contractAddress,
RefundDenyReason indexed reason,
uint256 gas
);
event Deposited(address sender, uint256 amount);
event Withdrawn(address initiator, address destination, uint256 amount);
event ContractAllowedSet(address indexed addr, bool indexed allowed);
event RefundeeAllowedSet(address indexed addr, bool indexed allowed);
event DisallowerSet(address indexed addr);
event CommonParameterSet(CommonParameterKey indexed parameter, uint256 value);
constructor() Ownable() {
commonParams = CommonParameters({
maxRefundeeBalance: 0, // no limit
extraGasMargin: 4000, // 4k gas
calldataCost: 12, // Between 4 for zero bytes and 16 for non-zero bytes
maxGasTip: 2 gwei,
maxGasCost: 120 gwei,
maxSingleGasUsage: 2e6 // 2 million gas
});
}
function setDisallower(address addr) external onlyOwner {
disallower = addr;
emit DisallowerSet(addr);
}
function allowContracts(address[] calldata addresses) external onlyOwner {
setContractsAllowedImpl(addresses, true);
}
function disallowContracts(address[] calldata addresses) external {
require(msg.sender == owner() || msg.sender == disallower, "NOT_AUTHORIZED");
setContractsAllowedImpl(addresses, false);
}
function setContractsAllowedImpl(address[] calldata addresses, bool allow) internal {
for (uint256 i = 0; i < addresses.length; i++) {
address addr = addresses[i];
allowedContracts[addr] = allow;
emit ContractAllowedSet(addr, allow);
}
}
function allowRefundees(address[] calldata addresses) external onlyOwner {
setRefundeesAllowedImpl(addresses, true);
}
function disallowRefundees(address[] calldata addresses) external {
require(msg.sender == owner() || msg.sender == disallower, "NOT_AUTHORIZED");
setRefundeesAllowedImpl(addresses, false);
}
function setRefundeesAllowedImpl(address[] calldata addresses, bool allow) internal {
for (uint256 i = 0; i < addresses.length; i++) {
address addr = addresses[i];
allowedRefundees[addr] = allow;
emit RefundeeAllowedSet(addr, allow);
}
}
function setMaxRefundeeBalance(uint128 newValue) external onlyOwner {
commonParams.maxRefundeeBalance = newValue;
emit CommonParameterSet(CommonParameterKey.MAX_REFUNDEE_BALANCE, newValue);
}
function setExtraGasMargin(uint32 newValue) external onlyOwner {
commonParams.extraGasMargin = newValue;
emit CommonParameterSet(CommonParameterKey.EXTRA_GAS_MARGIN, newValue);
}
function setCalldataCost(uint8 newValue) external onlyOwner {
commonParams.calldataCost = newValue;
emit CommonParameterSet(CommonParameterKey.CALLDATA_COST, newValue);
}
function setMaxGasTip(uint64 newValue) external onlyOwner {
commonParams.maxGasTip = newValue;
emit CommonParameterSet(CommonParameterKey.MAX_GAS_TIP, newValue);
}
function setMaxGasCost(uint64 newValue) external onlyOwner {
commonParams.maxGasCost = newValue;
emit CommonParameterSet(CommonParameterKey.MAX_GAS_COST, newValue);
}
function setMaxSingleGasUsage(uint32 newValue) external onlyOwner {
commonParams.maxSingleGasUsage = newValue;
emit CommonParameterSet(CommonParameterKey.MAX_SINGLE_GAS_USAGE, newValue);
}
receive() external payable {
emit Deposited(msg.sender, msg.value);
}
function withdraw(address payable destination, uint256 amount) external onlyOwner {
// It's expected that destination is an EOA
(bool success, ) = destination.call{ value: amount }("");
require(success, "WITHDRAW_FAILED");
emit Withdrawn(msg.sender, destination, amount);
}
function onGasSpent(
address payable refundee,
uint256 gasUsed,
uint256 calldataSize
) external override returns (bool success) {
uint256 startGasLeft = gasleft();
uint256 ownBalance = address(this).balance;
if (ownBalance == 0) {
emit RefundGasCostsDenied(refundee, msg.sender, RefundDenyReason.OUT_OF_FUNDS, gasUsed);
return false;
}
if (!allowedContracts[msg.sender]) {
emit RefundGasCostsDenied(
refundee,
msg.sender,
RefundDenyReason.CONTRACT_NOT_ALLOWED,
gasUsed
);
return false;
}
if (!allowedRefundees[refundee]) {
emit RefundGasCostsDenied(
refundee,
msg.sender,
RefundDenyReason.REFUNDEE_NOT_ALLOWED,
gasUsed
);
return false;
}
uint256 estGasPrice = block.basefee + commonParams.maxGasTip;
if (tx.gasprice < estGasPrice) {
estGasPrice = tx.gasprice;
}
if (commonParams.maxGasCost != 0 && estGasPrice > commonParams.maxGasCost) {
estGasPrice = commonParams.maxGasCost;
}
// Retrieve these variables before measuring gasleft()
uint256 refundeeBalance = refundee.balance;
uint256 maxRefundeeBalance = commonParams.maxRefundeeBalance;
uint256 maxSingleGasUsage = commonParams.maxSingleGasUsage;
// Add in a bit of a buffer for the tx costs not measured with gasleft
gasUsed +=
startGasLeft +
commonParams.extraGasMargin +
(calldataSize * commonParams.calldataCost);
// Split this up into two statements so that gasleft() comes after the storage loads
gasUsed -= gasleft();
if (maxSingleGasUsage != 0 && gasUsed > maxSingleGasUsage) {
gasUsed = maxSingleGasUsage;
}
uint256 refundAmount = estGasPrice * gasUsed;
if (maxRefundeeBalance != 0 && refundeeBalance + refundAmount > maxRefundeeBalance) {
if (refundeeBalance > maxRefundeeBalance) {
// The refundee is already above their max balance
emit RefundGasCostsDenied(
refundee,
msg.sender,
RefundDenyReason.REFUNDEE_ABOVE_MAX_BALANCE,
gasUsed
);
return false;
} else {
refundAmount = maxRefundeeBalance - refundeeBalance;
}
}
if (refundAmount > ownBalance) {
refundAmount = ownBalance;
}
// It's expected that refundee is an EOA
(success, ) = refundee.call{ value: refundAmount }("");
emit RefundedGasCosts(refundee, msg.sender, success, gasUsed, estGasPrice, refundAmount);
}
}
// SPDX-License-Identifier: Apache-2.0
/*
* Copyright 2021, Offchain Labs, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
pragma solidity >=0.6.11 <0.7.0 || >=0.8.7 <0.9.0;
interface IGasRefunder {
function onGasSpent(
address payable spender,
uint256 gasUsed,
uint256 calldataSize
) external returns (bool success);
}
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts v4.4.1 (access/Ownable.sol)
pragma solidity ^0.8.0;
import "../utils/Context.sol";
/**
* @dev Contract module which provides a basic access control mechanism, where
* there is an account (an owner) that can be granted exclusive access to
* specific functions.
*
* By default, the owner account will be the one that deploys the contract. This
* can later be changed with {transferOwnership}.
*
* This module is used through inheritance. It will make available the modifier
* `onlyOwner`, which can be applied to your functions to restrict their use to
* the owner.
*/
abstract contract Ownable is Context {
address private _owner;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
/**
* @dev Initializes the contract setting the deployer as the initial owner.
*/
constructor() {
_transferOwnership(_msgSender());
}
/**
* @dev Returns the address of the current owner.
*/
function owner() public view virtual returns (address) {
return _owner;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(owner() == _msgSender(), "Ownable: caller is not the owner");
_;
}
/**
* @dev Leaves the contract without owner. It will not be possible to call
* `onlyOwner` functions anymore. Can only be called by the current owner.
*
* NOTE: Renouncing ownership will leave the contract without an owner,
* thereby removing any functionality that is only available to the owner.
*/
function renounceOwnership() public virtual onlyOwner {
_transferOwnership(address(0));
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`).
* Can only be called by the current owner.
*/
function transferOwnership(address newOwner) public virtual onlyOwner {
require(newOwner != address(0), "Ownable: new owner is the zero address");
_transferOwnership(newOwner);
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`).
* Internal function without access restriction.
*/
function _transferOwnership(address newOwner) internal virtual {
address oldOwner = _owner;
_owner = newOwner;
emit OwnershipTransferred(oldOwner, newOwner);
}
}
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts v4.4.1 (utils/Context.sol)
pragma solidity ^0.8.0;
/**
* @dev Provides information about the current execution context, including the
* sender of the transaction and its data. While these are generally available
* via msg.sender and msg.data, they should not be accessed in such a direct
* manner, since when dealing with meta-transactions the account sending and
* paying for execution may not be the actual sender (as far as an application
* is concerned).
*
* This contract is only required for intermediate, library-like contracts.
*/
abstract contract Context {
function _msgSender() internal view virtual returns (address) {
return msg.sender;
}
function _msgData() internal view virtual returns (bytes calldata) {
return msg.data;
}
}
[{"inputs":[],"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"enum GasRefunder.CommonParameterKey","name":"parameter","type":"uint8"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"CommonParameterSet","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"addr","type":"address"},{"indexed":true,"internalType":"bool","name":"allowed","type":"bool"}],"name":"ContractAllowedSet","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"address","name":"sender","type":"address"},{"indexed":false,"internalType":"uint256","name":"amount","type":"uint256"}],"name":"Deposited","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"addr","type":"address"}],"name":"DisallowerSet","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"previousOwner","type":"address"},{"indexed":true,"internalType":"address","name":"newOwner","type":"address"}],"name":"OwnershipTransferred","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"refundee","type":"address"},{"indexed":true,"internalType":"address","name":"contractAddress","type":"address"},{"indexed":true,"internalType":"enum GasRefunder.RefundDenyReason","name":"reason","type":"uint8"},{"indexed":false,"internalType":"uint256","name":"gas","type":"uint256"}],"name":"RefundGasCostsDenied","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"refundee","type":"address"},{"indexed":true,"internalType":"address","name":"contractAddress","type":"address"},{"indexed":true,"internalType":"bool","name":"success","type":"bool"},{"indexed":false,"internalType":"uint256","name":"gas","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"gasPrice","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"amountPaid","type":"uint256"}],"name":"RefundedGasCosts","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"addr","type":"address"},{"indexed":true,"internalType":"bool","name":"allowed","type":"bool"}],"name":"RefundeeAllowedSet","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"address","name":"initiator","type":"address"},{"indexed":false,"internalType":"address","name":"destination","type":"address"},{"indexed":false,"internalType":"uint256","name":"amount","type":"uint256"}],"name":"Withdrawn","type":"event"},{"inputs":[{"internalType":"address[]","name":"addresses","type":"address[]"}],"name":"allowContracts","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address[]","name":"addresses","type":"address[]"}],"name":"allowRefundees","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"","type":"address"}],"name":"allowedContracts","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"","type":"address"}],"name":"allowedRefundees","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"commonParams","outputs":[{"internalType":"uint128","name":"maxRefundeeBalance","type":"uint128"},{"internalType":"uint32","name":"extraGasMargin","type":"uint32"},{"internalType":"uint8","name":"calldataCost","type":"uint8"},{"internalType":"uint64","name":"maxGasTip","type":"uint64"},{"internalType":"uint64","name":"maxGasCost","type":"uint64"},{"internalType":"uint32","name":"maxSingleGasUsage","type":"uint32"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address[]","name":"addresses","type":"address[]"}],"name":"disallowContracts","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address[]","name":"addresses","type":"address[]"}],"name":"disallowRefundees","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[],"name":"disallower","outputs":[{"internalType":"address","name":"","type":"address"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address payable","name":"refundee","type":"address"},{"internalType":"uint256","name":"gasUsed","type":"uint256"},{"internalType":"uint256","name":"calldataSize","type":"uint256"}],"name":"onGasSpent","outputs":[{"internalType":"bool","name":"success","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[],"name":"owner","outputs":[{"internalType":"address","name":"","type":"address"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"renounceOwnership","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint8","name":"newValue","type":"uint8"}],"name":"setCalldataCost","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"addr","type":"address"}],"name":"setDisallower","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint32","name":"newValue","type":"uint32"}],"name":"setExtraGasMargin","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint64","name":"newValue","type":"uint64"}],"name":"setMaxGasCost","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint64","name":"newValue","type":"uint64"}],"name":"setMaxGasTip","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint128","name":"newValue","type":"uint128"}],"name":"setMaxRefundeeBalance","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"uint32","name":"newValue","type":"uint32"}],"name":"setMaxSingleGasUsage","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"newOwner","type":"address"}],"name":"transferOwnership","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address payable","name":"destination","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"withdraw","outputs":[],"stateMutability":"nonpayable","type":"function"},{"stateMutability":"payable","type":"receive"}]
608060405234801561001057600080fd5b5061001a336100a5565b6040805160c08101825260008152610fa06020820152600c9181019190915263773594006060820152641bf08eb0006080820152621e848060a090910152600480546001600160e81b03191678773594000c00000fa000000000000000000000000000000000179055600580546001600160601b0319166a1e84800000001bf08eb0001790556100f5565b600080546001600160a01b038381166001600160a01b0319831681178455604051919092169283917f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e09190a35050565b611229806101046000396000f3fe6080604052600436106101235760003560e01c8063ca101295116100a0578063efe12b0111610064578063efe12b011461040d578063f1e845ca1461042d578063f2fde38b1461044d578063f3fef3a31461046d578063f52128eb1461048d57600080fd5b8063ca101295146102e0578063cd499da314610300578063d513894814610320578063e3db8a49146103cd578063e5207453146103ed57600080fd5b80637edddf45116100e75780637edddf451461022357806386b98895146102435780638da5cb5b14610263578063a89d217314610290578063bffe1780146102c057600080fd5b806325416bc9146101675780632ccb03f214610189578063500de431146101a957806351e0e26b146101c9578063715018a61461020e57600080fd5b3661016257604080513381523460208201527f2da466a7b24304f47e87fa2e1e5a81b9831ce54fec19055ce277ca2f39ba42c4910160405180910390a1005b600080fd5b34801561017357600080fd5b50610187610182366004610fbd565b6104ad565b005b34801561019557600080fd5b506101876101a4366004611080565b6104f5565b3480156101b557600080fd5b506101876101c436600461105a565b610571565b3480156101d557600080fd5b506101f96101e4366004610f3f565b60016020526000908152604090205460ff1681565b60405190151581526020015b60405180910390f35b34801561021a57600080fd5b506101876105e3565b34801561022f57600080fd5b5061018761023e36600461105a565b61061e565b34801561024f57600080fd5b5061018761025e366004611080565b610672565b34801561026f57600080fd5b506102786106cd565b6040516001600160a01b039091168152602001610205565b34801561029c57600080fd5b506101f96102ab366004610f3f565b60026020526000908152604090205460ff1681565b3480156102cc57600080fd5b506101876102db3660046110a9565b6106dc565b3480156102ec57600080fd5b506101876102fb366004610fbd565b610746565b34801561030c57600080fd5b5061018761031b366004610fbd565b610781565b34801561032c57600080fd5b5060045460055461037f916001600160801b0381169163ffffffff600160801b830481169260ff600160a01b820416926001600160401b03600160a81b90920482169291811691600160401b9091041686565b604080516001600160801b03909716875263ffffffff958616602088015260ff909416938601939093526001600160401b0391821660608601521660808401521660a082015260c001610205565b3480156103d957600080fd5b506101f96103e8366004610f88565b6107da565b3480156103f957600080fd5b50610187610408366004610fbd565b610ac0565b34801561041957600080fd5b50600354610278906001600160a01b031681565b34801561043957600080fd5b50610187610448366004610f3f565b610b19565b34801561045957600080fd5b50610187610468366004610f3f565b610b92565b34801561047957600080fd5b50610187610488366004610f5c565b610c32565b34801561049957600080fd5b506101876104a8366004611031565b610d44565b336104b66106cd565b6001600160a01b0316146104e55760405162461bcd60e51b81526004016104dc906110cc565b60405180910390fd5b6104f182826001610db7565b5050565b336104fe6106cd565b6001600160a01b0316146105245760405162461bcd60e51b81526004016104dc906110cc565b6005805467ffffffffffffffff19166001600160401b03831617905560045b6040516001600160401b03831681526000805160206111d4833981519152906020015b60405180910390a250565b3361057a6106cd565b6001600160a01b0316146105a05760405162461bcd60e51b81526004016104dc906110cc565b6005805463ffffffff60401b1916600160401b63ffffffff8416021781555b60405163ffffffff831681526000805160206111d483398151915290602001610566565b336105ec6106cd565b6001600160a01b0316146106125760405162461bcd60e51b81526004016104dc906110cc565b61061c6000610e56565b565b336106276106cd565b6001600160a01b03161461064d5760405162461bcd60e51b81526004016104dc906110cc565b6004805463ffffffff60801b1916600160801b63ffffffff84160217905560016105bf565b3361067b6106cd565b6001600160a01b0316146106a15760405162461bcd60e51b81526004016104dc906110cc565b6004805467ffffffffffffffff60a81b1916600160a81b6001600160401b038416021790556003610543565b6000546001600160a01b031690565b336106e56106cd565b6001600160a01b03161461070b5760405162461bcd60e51b81526004016104dc906110cc565b6004805460ff60a01b1916600160a01b60ff841602179055600260405160ff831681526000805160206111d483398151915290602001610566565b3361074f6106cd565b6001600160a01b0316146107755760405162461bcd60e51b81526004016104dc906110cc565b6104f182826001610ea6565b6107896106cd565b6001600160a01b0316336001600160a01b031614806107b257506003546001600160a01b031633145b6107ce5760405162461bcd60e51b81526004016104dc90611101565b6104f182826000610db7565b6000805a905047806108345760035b60405186815233906001600160a01b038916907f2b8ae00e22d9eaf5a92820a22b947c007aee773fa36502ad7a1c9a464ab4932b9060200160405180910390a4600092505050610ab9565b3360009081526001602052604090205460ff166108525760006107e9565b6001600160a01b03861660009081526002602052604090205460ff166108795760016107e9565b60045460009061089990600160a81b90046001600160401b031648611129565b9050803a10156108a657503a5b6005546001600160401b0316158015906108ca57506005546001600160401b031681115b156108dd57506005546001600160401b03165b6004546005546001600160a01b03891631916001600160801b03811691600160401b900463ffffffff169061091c90600160a01b900460ff1689611141565b60045461093690600160801b900463ffffffff1688611129565b6109409190611129565b61094a908a611129565b98505a610957908a611160565b9850801580159061096757508089115b15610970578098505b600061097c8a86611141565b905082158015906109955750826109938286611129565b115b15610a0157828411156109f45760026040518b815233906001600160a01b038e16907f2b8ae00e22d9eaf5a92820a22b947c007aee773fa36502ad7a1c9a464ab4932b9060200160405180910390a46000975050505050505050610ab9565b6109fe8484611160565b90505b85811115610a0c5750845b6040516001600160a01b038c16908290600081818185875af1925050503d8060008114610a55576040519150601f19603f3d011682016040523d82523d6000602084013e610a5a565b606091505b5050604080518c8152602081018890529081018390529098508815159033906001600160a01b038e16907fd0224505f828ccfcbc56ca0590d97442e239a7aa770f712948fd6388356b20de9060600160405180910390a4505050505050505b9392505050565b610ac86106cd565b6001600160a01b0316336001600160a01b03161480610af157506003546001600160a01b031633145b610b0d5760405162461bcd60e51b81526004016104dc90611101565b6104f182826000610ea6565b33610b226106cd565b6001600160a01b031614610b485760405162461bcd60e51b81526004016104dc906110cc565b600380546001600160a01b0319166001600160a01b0383169081179091556040517fc388cec0895ad7ee4635898ec92207ca48d42256d4355f7042efef62c368a97990600090a250565b33610b9b6106cd565b6001600160a01b031614610bc15760405162461bcd60e51b81526004016104dc906110cc565b6001600160a01b038116610c265760405162461bcd60e51b815260206004820152602660248201527f4f776e61626c653a206e6577206f776e657220697320746865207a65726f206160448201526564647265737360d01b60648201526084016104dc565b610c2f81610e56565b50565b33610c3b6106cd565b6001600160a01b031614610c615760405162461bcd60e51b81526004016104dc906110cc565b6000826001600160a01b03168260405160006040518083038185875af1925050503d8060008114610cae576040519150601f19603f3d011682016040523d82523d6000602084013e610cb3565b606091505b5050905080610cf65760405162461bcd60e51b815260206004820152600f60248201526e15d2551211149055d7d19052531151608a1b60448201526064016104dc565b604080513381526001600160a01b03851660208201529081018390527fd1c19fbcd4551a5edfb66d43d2e337c04837afda3482b42bdf569a8fccdae5fb9060600160405180910390a1505050565b33610d4d6106cd565b6001600160a01b031614610d735760405162461bcd60e51b81526004016104dc906110cc565b600480546001600160801b0319166001600160801b03831617905560006040516001600160801b03831681526000805160206111d483398151915290602001610566565b60005b82811015610e50576000848483818110610dd657610dd66111a8565b9050602002016020810190610deb9190610f3f565b6001600160a01b038116600081815260016020526040808220805460ff19168815159081179091559051939450927fb0918cd965657b8d231f8adba328fa810b6d61d800de9c795d40eb3623498c019190a35080610e4881611177565b915050610dba565b50505050565b600080546001600160a01b038381166001600160a01b0319831681178455604051919092169283917f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e09190a35050565b60005b82811015610e50576000848483818110610ec557610ec56111a8565b9050602002016020810190610eda9190610f3f565b6001600160a01b038116600081815260026020526040808220805460ff19168815159081179091559051939450927ff544cca9d5484bfd447775bd759d12d53f1aa7c5f770be82c55070798ff9c63e9190a35080610f3781611177565b915050610ea9565b600060208284031215610f5157600080fd5b8135610ab9816111be565b60008060408385031215610f6f57600080fd5b8235610f7a816111be565b946020939093013593505050565b600080600060608486031215610f9d57600080fd5b8335610fa8816111be565b95602085013595506040909401359392505050565b60008060208385031215610fd057600080fd5b82356001600160401b0380821115610fe757600080fd5b818501915085601f830112610ffb57600080fd5b81358181111561100a57600080fd5b8660208260051b850101111561101f57600080fd5b60209290920196919550909350505050565b60006020828403121561104357600080fd5b81356001600160801b0381168114610ab957600080fd5b60006020828403121561106c57600080fd5b813563ffffffff81168114610ab957600080fd5b60006020828403121561109257600080fd5b81356001600160401b0381168114610ab957600080fd5b6000602082840312156110bb57600080fd5b813560ff81168114610ab957600080fd5b6020808252818101527f4f776e61626c653a2063616c6c6572206973206e6f7420746865206f776e6572604082015260600190565b6020808252600e908201526d1393d517d055551213d49256915160921b604082015260600190565b6000821982111561113c5761113c611192565b500190565b600081600019048311821515161561115b5761115b611192565b500290565b60008282101561117257611172611192565b500390565b600060001982141561118b5761118b611192565b5060010190565b634e487b7160e01b600052601160045260246000fd5b634e487b7160e01b600052603260045260246000fd5b6001600160a01b0381168114610c2f57600080fdfeda79b6b81f905f788560507c685a42d5a8ab209ee26538cbcf3ce3caed601f9ba26469706673582212203ee6596b1aa27e0fbc7cd50eb82aab26dae349bdc40a8426a1a5018e2fca032364736f6c63430008070033
Checkout more smart contracts
- Lido
- Proposer Fee Recipient
- Router
- Verified, Router
- Router
- Verified, Router
- Router

LidoExecutionLayerRewardsVault
The following smart contract is called LidoExecutionLayerRewardsVault. It is used to manage rewards for the Lido protocol. The contract allows Lido to withdraw rewards, recover ERC20 and ERC721 tokens, and receive ETH. The contract uses the SafeERC20 library to ensure safe transfers of ERC20 tokens. The purpose of this contract is to provide a secure and efficient way to manage rewards for the Lido protocol.

Registry
The following smart contract is a Registry contract that manages routes for cross-chain transfers. It allows adding, disabling, and executing routes for middleware and bridge contracts. It also includes a function for rescuing funds and uses OpenZeppelin libraries for access control and ERC20 token handling.

GasRefunder
The following smart contract is a GasRefunder contract that refunds gas costs to specified refundees. It allows the owner to set common parameters such as maximum refundee balance, extra gas margin, calldata cost, maximum gas tip, maximum gas cost, and maximum single gas usage. The contract also allows the owner to set allowed contracts and refundees, and withdraw funds.
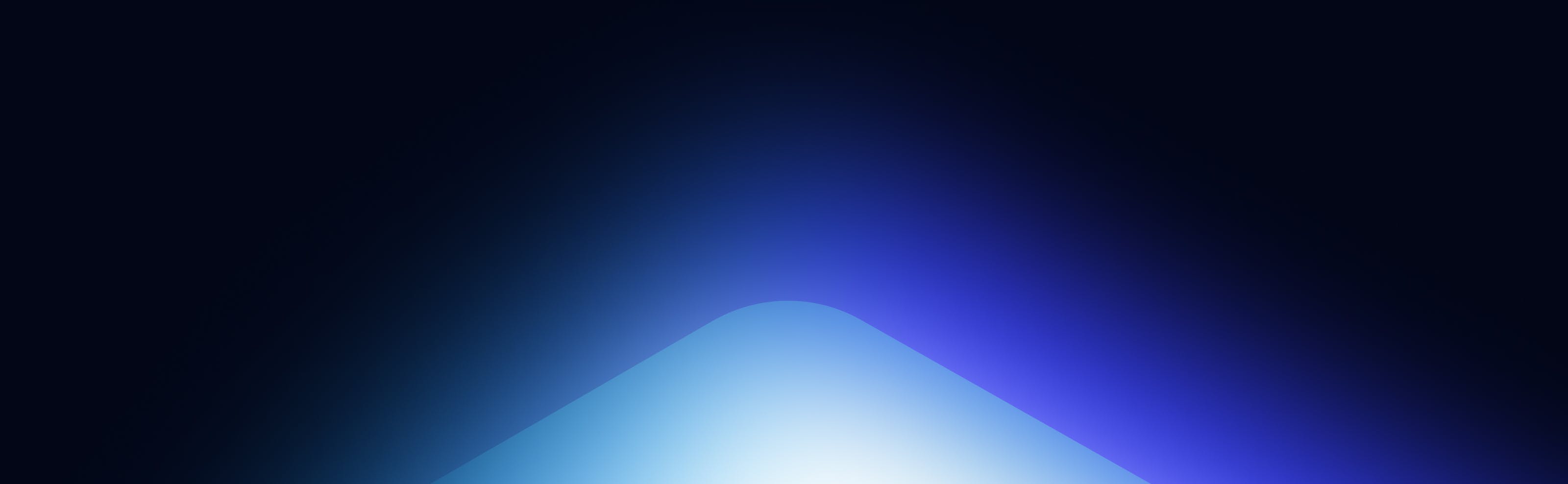