- Verified, Token
- Fungible Token
- ERC-20
The following smart contract is a basic ERC20 token contract called OrdinalBTC. It has a constructor that takes in a uint parameter representing the initial supply of the token. The constructor then calls the _mint function to mint the initial supply of the token and assign it to the contract deployer's address. The name of the token is "Ordinal BTC" and its symbol is "oBTC".
/**
,.=ctE55ttt553tzs.,
,,c5;z==!!:::: .::7:==it3>.,
,xC;z!:::::: ::::::::::::!=c33x,
,czz!::::: ::;;..===:..::: ::::!ct3.
,C;/.:: : ;=c!:::::::::::::::.. !tt3.
/z/.: :;z!:::::J :E3. E:::::::.. !ct3.
,E;F ::;t::::::::J :E3. E::. ::. \ttL
;E7. :c::::F****** **. *==c;.. :: Jttk
.EJ. ;::::::L "\:. ::. Jttl
[:. :::::::::773. JE773zs. I:. ::::. It3L
;:[ L:::::::::::L |t::!::J |:::::::: :Et3
[:L !::::::::::::L |t::;z2F .Et:::.:::. ::[13
E:. !::::::::::::L =Et::::::::! ::|13
E:. (::::::::::::L ....... \:::::::! ::|i3
[:L !:::: ::L |3t::::!3. ]::::::. ::[13
!:( .::::: ::L |t::::::3L |:::::; ::::EE3
E3. :::::::::;z5. Jz;;;z=F. :E:::::.::::II3[
Jt1. :::::::[ ;z5::::;.::::;3t3
\z1.::::::::::l...... .. ;.=ct5::::::/.::::;Et3L
\t3.:::::::::::::::J :E3. Et::::::::;!:::::;5E3L
"cz\.:::::::::::::J E3. E:::::::z! ;Zz37`
\z3. ::;:::::::::::::::;=' ./355F
\z3x. ::=======' ,c253F
"tz3=. ..c5t32^
"=zz3==... ...=t3z13P^
`*=zjzczIIII3zzztE3>*^`
Ordinal BTC
The initial specified satoshis 'Ordinals' on the Ethereum blockchain utilising
the bitcoin blockchain. Explore each Inscription and Ordinal Exactly like you
would there. Many fully synchronised Bitcoin Core nodes will be available for
usage on our upcoming Dapp.
Twitter: https://twitter.com/OrdinalBTC
*/
pragma solidity ^0.8.0;
interface IERC20 {
function totalSupply() external view returns (uint256);
function balanceOf(address account) external view returns (uint256);
function transfer(address recipient, uint256 amount) external returns (bool);
function allowance(address owner, address spender) external view returns (uint256);
function approve(address spender, uint256 amount) external returns (bool);
function transferFrom(address sender, address recipient, uint256 amount) external returns (bool);
event Transfer(address indexed from, address indexed to, uint256 value);
event Approval(address indexed owner, address indexed spender, uint256 value);
}
abstract contract Context {
function _msgSender() internal view virtual returns (address) {
return msg.sender;
}
function _msgData() internal view virtual returns (bytes calldata) {
this;
return msg.data;
}
}
contract ERC20 is Context, IERC20 {
mapping (address => uint256) private _balances;
mapping (address => mapping (address => uint256)) private _allowances;
uint256 private _totalSupply;
string private _name;
string private _symbol;
constructor (string memory name_, string memory symbol_) {
_name = name_;
_symbol = symbol_;
}
function name() public view virtual returns (string memory) {
return _name;
}
function symbol() public view virtual returns (string memory) {
return _symbol;
}
function decimals() public view virtual returns (uint8) {
return 18;
}
function totalSupply() public view virtual override returns (uint256) {
return _totalSupply;
}
function balanceOf(address account) public view virtual override returns (uint256) {
return _balances[account];
}
function transfer(address recipient, uint256 amount) public virtual override returns (bool) {
_transfer(_msgSender(), recipient, amount);
return true;
}
function allowance(address owner, address spender) public view virtual override returns (uint256) {
return _allowances[owner][spender];
}
function approve(address spender, uint256 amount) public virtual override returns (bool) {
_approve(_msgSender(), spender, amount);
return true;
}
function transferFrom(address sender, address recipient, uint256 amount) public virtual override returns (bool) {
_transfer(sender, recipient, amount);
uint256 currentAllowance = _allowances[sender][_msgSender()];
require(currentAllowance >= amount, "ERC20: transfer amount exceeds allowance");
_approve(sender, _msgSender(), currentAllowance - amount);
return true;
}
function increaseAllowance(address spender, uint256 addedValue) public virtual returns (bool) {
_approve(_msgSender(), spender, _allowances[_msgSender()][spender] + addedValue);
return true;
}
function decreaseAllowance(address spender, uint256 subtractedValue) public virtual returns (bool) {
uint256 currentAllowance = _allowances[_msgSender()][spender];
require(currentAllowance >= subtractedValue, "ERC20: decreased allowance below zero");
_approve(_msgSender(), spender, currentAllowance - subtractedValue);
return true;
}
function _transfer(address sender, address recipient, uint256 amount) internal virtual {
require(sender != address(0), "ERC20: transfer from the zero address");
require(recipient != address(0), "ERC20: transfer to the zero address");
_beforeTokenTransfer(sender, recipient, amount);
uint256 senderBalance = _balances[sender];
require(senderBalance >= amount, "ERC20: transfer amount exceeds balance");
_balances[sender] = senderBalance - amount;
_balances[recipient] += amount;
emit Transfer(sender, recipient, amount);
}
function _mint(address account, uint256 amount) internal virtual {
require(account != address(0), "ERC20: mint to the zero address");
_beforeTokenTransfer(address(0), account, amount);
_totalSupply += amount;
_balances[account] += amount;
emit Transfer(address(0), account, amount);
}
function _burn(address account, uint256 amount) internal virtual {
require(account != address(0), "ERC20: burn from the zero address");
_beforeTokenTransfer(account, address(0), amount);
uint256 accountBalance = _balances[account];
require(accountBalance >= amount, "ERC20: burn amount exceeds balance");
_balances[account] = accountBalance - amount;
_totalSupply -= amount;
emit Transfer(account, address(0), amount);
}
function _approve(address owner, address spender, uint256 amount) internal virtual {
require(owner != address(0), "ERC20: approve from the zero address");
require(spender != address(0), "ERC20: approve to the zero address");
_allowances[owner][spender] = amount;
emit Approval(owner, spender, amount);
}
function _beforeTokenTransfer(address from, address to, uint256 amount) internal virtual { }
}
contract OrdinalBTC is ERC20 {
constructor(uint supply) ERC20("Ordinal BTC ", "oBTC") {
_mint(msg.sender, supply);
}
}
[{"inputs":[{"internalType":"uint256","name":"supply","type":"uint256"}],"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"owner","type":"address"},{"indexed":true,"internalType":"address","name":"spender","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Approval","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"from","type":"address"},{"indexed":true,"internalType":"address","name":"to","type":"address"},{"indexed":false,"internalType":"uint256","name":"value","type":"uint256"}],"name":"Transfer","type":"event"},{"inputs":[{"internalType":"address","name":"owner","type":"address"},{"internalType":"address","name":"spender","type":"address"}],"name":"allowance","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"approve","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"account","type":"address"}],"name":"balanceOf","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"decimals","outputs":[{"internalType":"uint8","name":"","type":"uint8"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"subtractedValue","type":"uint256"}],"name":"decreaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"spender","type":"address"},{"internalType":"uint256","name":"addedValue","type":"uint256"}],"name":"increaseAllowance","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[],"name":"name","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"symbol","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"totalSupply","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"recipient","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transfer","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"sender","type":"address"},{"internalType":"address","name":"recipient","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"transferFrom","outputs":[{"internalType":"bool","name":"","type":"bool"}],"stateMutability":"nonpayable","type":"function"}]
60806040523480156200001157600080fd5b506040516200189538038062001895833981810160405281019062000037919062000321565b6040518060400160405280600c81526020017f4f7264696e616c204254432000000000000000000000000000000000000000008152506040518060400160405280600481526020017f6f425443000000000000000000000000000000000000000000000000000000008152508160039080519060200190620000bb9291906200025a565b508060049080519060200190620000d49291906200025a565b505050620000e93382620000f060201b60201c565b5062000505565b600073ffffffffffffffffffffffffffffffffffffffff168273ffffffffffffffffffffffffffffffffffffffff16141562000163576040517f08c379a00000000000000000000000000000000000000000000000000000000081526004016200015a90620003a0565b60405180910390fd5b62000177600083836200025560201b60201c565b80600260008282546200018b9190620003f0565b92505081905550806000808473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020019081526020016000206000828254620001e29190620003f0565b925050819055508173ffffffffffffffffffffffffffffffffffffffff16600073ffffffffffffffffffffffffffffffffffffffff167fddf252ad1be2c89b69c2b068fc378daa952ba7f163c4a11628f55a4df523b3ef83604051620002499190620003c2565b60405180910390a35050565b505050565b828054620002689062000457565b90600052602060002090601f0160209004810192826200028c5760008555620002d8565b82601f10620002a757805160ff1916838001178555620002d8565b82800160010185558215620002d8579182015b82811115620002d7578251825591602001919060010190620002ba565b5b509050620002e79190620002eb565b5090565b5b8082111562000306576000816000905550600101620002ec565b5090565b6000815190506200031b81620004eb565b92915050565b6000602082840312156200033457600080fd5b600062000344848285016200030a565b91505092915050565b60006200035c601f83620003df565b91507f45524332303a206d696e7420746f20746865207a65726f2061646472657373006000830152602082019050919050565b6200039a816200044d565b82525050565b60006020820190508181036000830152620003bb816200034d565b9050919050565b6000602082019050620003d960008301846200038f565b92915050565b600082825260208201905092915050565b6000620003fd826200044d565b91506200040a836200044d565b9250827fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff038211156200044257620004416200048d565b5b828201905092915050565b6000819050919050565b600060028204905060018216806200047057607f821691505b60208210811415620004875762000486620004bc565b5b50919050565b7f4e487b7100000000000000000000000000000000000000000000000000000000600052601160045260246000fd5b7f4e487b7100000000000000000000000000000000000000000000000000000000600052602260045260246000fd5b620004f6816200044d565b81146200050257600080fd5b50565b61138080620005156000396000f3fe608060405234801561001057600080fd5b50600436106100a95760003560e01c80633950935111610071578063395093511461016857806370a082311461019857806395d89b41146101c8578063a457c2d7146101e6578063a9059cbb14610216578063dd62ed3e14610246576100a9565b806306fdde03146100ae578063095ea7b3146100cc57806318160ddd146100fc57806323b872dd1461011a578063313ce5671461014a575b600080fd5b6100b6610276565b6040516100c39190611015565b60405180910390f35b6100e660048036038101906100e19190610c8e565b610308565b6040516100f39190610ffa565b60405180910390f35b610104610326565b6040516101119190611117565b60405180910390f35b610134600480360381019061012f9190610c3f565b610330565b6040516101419190610ffa565b60405180910390f35b610152610431565b60405161015f9190611132565b60405180910390f35b610182600480360381019061017d9190610c8e565b61043a565b60405161018f9190610ffa565b60405180910390f35b6101b260048036038101906101ad9190610bda565b6104e6565b6040516101bf9190611117565b60405180910390f35b6101d061052e565b6040516101dd9190611015565b60405180910390f35b61020060048036038101906101fb9190610c8e565b6105c0565b60405161020d9190610ffa565b60405180910390f35b610230600480360381019061022b9190610c8e565b6106b4565b60405161023d9190610ffa565b60405180910390f35b610260600480360381019061025b9190610c03565b6106d2565b60405161026d9190611117565b60405180910390f35b6060600380546102859061127b565b80601f01602080910402602001604051908101604052809291908181526020018280546102b19061127b565b80156102fe5780601f106102d3576101008083540402835291602001916102fe565b820191906000526020600020905b8154815290600101906020018083116102e157829003601f168201915b5050505050905090565b600061031c610315610759565b8484610761565b6001905092915050565b6000600254905090565b600061033d84848461092c565b6000600160008673ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020019081526020016000206000610388610759565b73ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002054905082811015610408576040517f08c379a00000000000000000000000000000000000000000000000000000000081526004016103ff90611097565b60405180910390fd5b61042585610414610759565b858461042091906111bf565b610761565b60019150509392505050565b60006012905090565b60006104dc610447610759565b848460016000610455610759565b73ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002060008873ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff168152602001908152602001600020546104d79190611169565b610761565b6001905092915050565b60008060008373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff168152602001908152602001600020549050919050565b60606004805461053d9061127b565b80601f01602080910402602001604051908101604052809291908181526020018280546105699061127b565b80156105b65780601f1061058b576101008083540402835291602001916105b6565b820191906000526020600020905b81548152906001019060200180831161059957829003601f168201915b5050505050905090565b600080600160006105cf610759565b73ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002060008573ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020019081526020016000205490508281101561068c576040517f08c379a0000000000000000000000000000000000000000000000000000000008152600401610683906110f7565b60405180910390fd5b6106a9610697610759565b8585846106a491906111bf565b610761565b600191505092915050565b60006106c86106c1610759565b848461092c565b6001905092915050565b6000600160008473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002060008373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002054905092915050565b600033905090565b600073ffffffffffffffffffffffffffffffffffffffff168373ffffffffffffffffffffffffffffffffffffffff1614156107d1576040517f08c379a00000000000000000000000000000000000000000000000000000000081526004016107c8906110d7565b60405180910390fd5b600073ffffffffffffffffffffffffffffffffffffffff168273ffffffffffffffffffffffffffffffffffffffff161415610841576040517f08c379a000000000000000000000000000000000000000000000000000000000815260040161083890611057565b60405180910390fd5b80600160008573ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002060008473ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff168152602001908152602001600020819055508173ffffffffffffffffffffffffffffffffffffffff168373ffffffffffffffffffffffffffffffffffffffff167f8c5be1e5ebec7d5bd14f71427d1e84f3dd0314c0f7b2291e5b200ac8c7c3b9258360405161091f9190611117565b60405180910390a3505050565b600073ffffffffffffffffffffffffffffffffffffffff168373ffffffffffffffffffffffffffffffffffffffff16141561099c576040517f08c379a0000000000000000000000000000000000000000000000000000000008152600401610993906110b7565b60405180910390fd5b600073ffffffffffffffffffffffffffffffffffffffff168273ffffffffffffffffffffffffffffffffffffffff161415610a0c576040517f08c379a0000000000000000000000000000000000000000000000000000000008152600401610a0390611037565b60405180910390fd5b610a17838383610bab565b60008060008573ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002054905081811015610a9d576040517f08c379a0000000000000000000000000000000000000000000000000000000008152600401610a9490611077565b60405180910390fd5b8181610aa991906111bf565b6000808673ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002081905550816000808573ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff1681526020019081526020016000206000828254610b399190611169565b925050819055508273ffffffffffffffffffffffffffffffffffffffff168473ffffffffffffffffffffffffffffffffffffffff167fddf252ad1be2c89b69c2b068fc378daa952ba7f163c4a11628f55a4df523b3ef84604051610b9d9190611117565b60405180910390a350505050565b505050565b600081359050610bbf8161131c565b92915050565b600081359050610bd481611333565b92915050565b600060208284031215610bec57600080fd5b6000610bfa84828501610bb0565b91505092915050565b60008060408385031215610c1657600080fd5b6000610c2485828601610bb0565b9250506020610c3585828601610bb0565b9150509250929050565b600080600060608486031215610c5457600080fd5b6000610c6286828701610bb0565b9350506020610c7386828701610bb0565b9250506040610c8486828701610bc5565b9150509250925092565b60008060408385031215610ca157600080fd5b6000610caf85828601610bb0565b9250506020610cc085828601610bc5565b9150509250929050565b610cd381611205565b82525050565b6000610ce48261114d565b610cee8185611158565b9350610cfe818560208601611248565b610d078161130b565b840191505092915050565b6000610d1f602383611158565b91507f45524332303a207472616e7366657220746f20746865207a65726f206164647260008301527f65737300000000000000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610d85602283611158565b91507f45524332303a20617070726f766520746f20746865207a65726f20616464726560008301527f73730000000000000000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610deb602683611158565b91507f45524332303a207472616e7366657220616d6f756e742065786365656473206260008301527f616c616e636500000000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610e51602883611158565b91507f45524332303a207472616e7366657220616d6f756e742065786365656473206160008301527f6c6c6f77616e63650000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610eb7602583611158565b91507f45524332303a207472616e736665722066726f6d20746865207a65726f20616460008301527f64726573730000000000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610f1d602483611158565b91507f45524332303a20617070726f76652066726f6d20746865207a65726f2061646460008301527f72657373000000000000000000000000000000000000000000000000000000006020830152604082019050919050565b6000610f83602583611158565b91507f45524332303a2064656372656173656420616c6c6f77616e63652062656c6f7760008301527f207a65726f0000000000000000000000000000000000000000000000000000006020830152604082019050919050565b610fe581611231565b82525050565b610ff48161123b565b82525050565b600060208201905061100f6000830184610cca565b92915050565b6000602082019050818103600083015261102f8184610cd9565b905092915050565b6000602082019050818103600083015261105081610d12565b9050919050565b6000602082019050818103600083015261107081610d78565b9050919050565b6000602082019050818103600083015261109081610dde565b9050919050565b600060208201905081810360008301526110b081610e44565b9050919050565b600060208201905081810360008301526110d081610eaa565b9050919050565b600060208201905081810360008301526110f081610f10565b9050919050565b6000602082019050818103600083015261111081610f76565b9050919050565b600060208201905061112c6000830184610fdc565b92915050565b60006020820190506111476000830184610feb565b92915050565b600081519050919050565b600082825260208201905092915050565b600061117482611231565b915061117f83611231565b9250827fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff038211156111b4576111b36112ad565b5b828201905092915050565b60006111ca82611231565b91506111d583611231565b9250828210156111e8576111e76112ad565b5b828203905092915050565b60006111fe82611211565b9050919050565b60008115159050919050565b600073ffffffffffffffffffffffffffffffffffffffff82169050919050565b6000819050919050565b600060ff82169050919050565b60005b8381101561126657808201518184015260208101905061124b565b83811115611275576000848401525b50505050565b6000600282049050600182168061129357607f821691505b602082108114156112a7576112a66112dc565b5b50919050565b7f4e487b7100000000000000000000000000000000000000000000000000000000600052601160045260246000fd5b7f4e487b7100000000000000000000000000000000000000000000000000000000600052602260045260246000fd5b6000601f19601f8301169050919050565b611325816111f3565b811461133057600080fd5b50565b61133c81611231565b811461134757600080fd5b5056fea2646970667358221220a8d7f40ebbd9f1ae794308454bd45f46a7457d176dcd62455900bdfd0758ee2a64736f6c63430008000033000000000000000000000000000000000000000000115eec47f6cf7e35000000
Checkout more smart contracts
- Verified
- Fungible Token
- ERC20
- Verified, Token
- LooksRare
- Fungible Token
- ERC-20
- Verified, Token
- Fungible Token
- ERC-20

SHILAINU
The following smart contract is the SHILAINU token contract, which is an ERC20 token with a total supply of 1 trillion. It includes features such as transaction limits, fees, and automatic liquidity provision. The contract also has a blacklist mode and the ability to set fee and transaction exemptions for specific addresses. The purpose of the contract is to provide a decentralized currency for the Shiba Inu community.

LooksRareAirdrop
The following smart contract is a LooksRareAirdrop contract that allows users to claim airdrop rewards in the form of ERC20 tokens. Users must provide a valid merkle proof and meet certain requirements, including having a signed maker order and approval for the collection. The contract is pausable and has a maximum amount that can be claimed. The owner can set the merkle root, update the end timestamp, and withdraw token rewards.

WETH9
The following smart contract is a basic implementation of the Wrapped Ether (WETH) token on the Ethereum blockchain. It allows users to deposit Ether into the contract and receive WETH tokens in return, which can be transferred to other users or contracts. The contract also includes functions for withdrawing Ether, checking balances, and approving transfers. The WETH token has a fixed supply of 18 decimal places and is represented by the symbol "WETH".
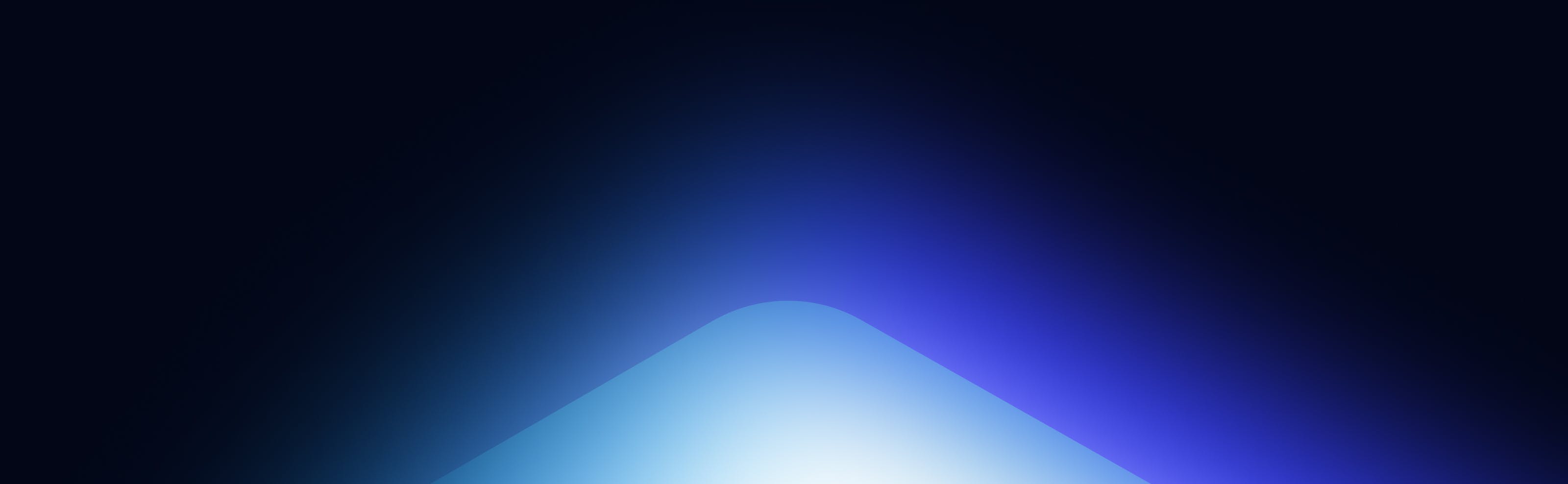