- Verified
- Proxy
This contract is a proxy and the implementation details are not yet known.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.10;
/******************************************************************************\
* A custom implementation of EIP-2535
* EIP-2535: https://eips.ethereum.org/EIPS/eip-2535
/******************************************************************************/
import {ParaProxyLib} from "./lib/ParaProxyLib.sol";
import {IParaProxy} from "../../../interfaces/IParaProxy.sol";
contract ParaProxy is IParaProxy {
constructor(address _contractOwner) payable {
ParaProxyLib.setContractOwner(_contractOwner);
}
function updateImplementation(
ProxyImplementation[] calldata _implementationParams,
address _init,
bytes calldata _calldata
) external override {
ParaProxyLib.enforceIsContractOwner();
ParaProxyLib.updateImplementation(
_implementationParams,
_init,
_calldata
);
}
// Find implementation for function that is called and execute the
// function if a implementation is found and return any value.
fallback() external payable {
ParaProxyLib.ProxyStorage storage ds;
bytes32 position = ParaProxyLib.PROXY_STORAGE_POSITION;
// get proxy storage
assembly {
ds.slot := position
}
// get implementation from function selector
address implementation = ds
.selectorToImplAndPosition[msg.sig]
.implAddress;
require(
implementation != address(0),
"ParaProxy: Function does not exist"
);
// Execute external function from implementation using delegatecall and return any value.
assembly {
// copy function selector and any arguments
calldatacopy(0, 0, calldatasize())
// execute function call using the implementation
let result := delegatecall(
gas(),
implementation,
0,
calldatasize(),
0,
0
)
// get any return value
returndatacopy(0, 0, returndatasize())
// return any return value or error back to the caller
switch result
case 0 {
revert(0, returndatasize())
}
default {
return(0, returndatasize())
}
}
}
receive() external payable {}
}
// SPDX-License-Identifier: AGPL-3.0
pragma solidity ^0.8.10;
/******************************************************************************\
* EIP-2535: https://eips.ethereum.org/EIPS/eip-2535
/******************************************************************************/
interface IParaProxy {
enum ProxyImplementationAction {
Add,
Replace,
Remove
}
// Add=0, Replace=1, Remove=2
struct ProxyImplementation {
address implAddress;
ProxyImplementationAction action;
bytes4[] functionSelectors;
}
/// @notice Add/replace/remove any number of functions and optionally execute
/// a function with delegatecall
/// @param _implementationParams Contains the implementation addresses and function selectors
/// @param _init The address of the contract or implementation to execute _calldata
/// @param _calldata A function call, including function selector and arguments
/// _calldata is executed with delegatecall on _init
function updateImplementation(
ProxyImplementation[] calldata _implementationParams,
address _init,
bytes calldata _calldata
) external;
event ImplementationUpdated(
ProxyImplementation[] _implementationParams,
address _init,
bytes _calldata
);
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.10;
/******************************************************************************\
* EIP-2535 Diamonds: https://eips.ethereum.org/EIPS/eip-2535
/******************************************************************************/
import {IParaProxy} from "../../../../interfaces/IParaProxy.sol";
library ParaProxyLib {
bytes32 constant PROXY_STORAGE_POSITION =
bytes32(
uint256(keccak256("paraspace.proxy.implementation.storage")) - 1
);
struct ImplementationAddressAndPosition {
address implAddress;
uint96 functionSelectorPosition; // position in implementationFunctionSelectors.functionSelectors array
}
struct ImplementationFunctionSelectors {
bytes4[] functionSelectors;
uint256 implementationAddressPosition; // position of implAddress in implementationAddresses array
}
struct ProxyStorage {
// maps function selector to the implementation address and
// the position of the selector in the implementationFunctionSelectors.selectors array
mapping(bytes4 => ImplementationAddressAndPosition) selectorToImplAndPosition;
// maps implementation addresses to function selectors
mapping(address => ImplementationFunctionSelectors) implementationFunctionSelectors;
// implementation addresses
address[] implementationAddresses;
// Used to query if a contract implements an interface.
// Used to implement ERC-165.
mapping(bytes4 => bool) supportedInterfaces;
// owner of the contract
address contractOwner;
}
function diamondStorage() internal pure returns (ProxyStorage storage ds) {
bytes32 position = PROXY_STORAGE_POSITION;
assembly {
ds.slot := position
}
}
event OwnershipTransferred(
address indexed previousOwner,
address indexed newOwner
);
function setContractOwner(address _newOwner) internal {
ProxyStorage storage ds = diamondStorage();
address previousOwner = ds.contractOwner;
ds.contractOwner = _newOwner;
emit OwnershipTransferred(previousOwner, _newOwner);
}
function contractOwner() internal view returns (address contractOwner_) {
contractOwner_ = diamondStorage().contractOwner;
}
function enforceIsContractOwner() internal view {
require(
msg.sender == diamondStorage().contractOwner,
"ParaProxy: Must be contract owner"
);
}
event ImplementationUpdated(
IParaProxy.ProxyImplementation[] _implementationData,
address _init,
bytes _calldata
);
// Internal function version of diamondCut
function updateImplementation(
IParaProxy.ProxyImplementation[] memory _implementationData,
address _init,
bytes memory _calldata
) internal {
for (
uint256 implIndex;
implIndex < _implementationData.length;
implIndex++
) {
IParaProxy.ProxyImplementationAction action = _implementationData[
implIndex
].action;
if (action == IParaProxy.ProxyImplementationAction.Add) {
addFunctions(
_implementationData[implIndex].implAddress,
_implementationData[implIndex].functionSelectors
);
} else if (action == IParaProxy.ProxyImplementationAction.Replace) {
replaceFunctions(
_implementationData[implIndex].implAddress,
_implementationData[implIndex].functionSelectors
);
} else if (action == IParaProxy.ProxyImplementationAction.Remove) {
removeFunctions(
_implementationData[implIndex].implAddress,
_implementationData[implIndex].functionSelectors
);
} else {
revert("ParaProxy: Incorrect ProxyImplementationAction");
}
}
emit ImplementationUpdated(_implementationData, _init, _calldata);
initializeImplementation(_init, _calldata);
}
function addFunctions(
address _implementationAddress,
bytes4[] memory _functionSelectors
) internal {
require(
_functionSelectors.length > 0,
"ParaProxy: No selectors in implementation to cut"
);
ProxyStorage storage ds = diamondStorage();
require(
_implementationAddress != address(0),
"ParaProxy: Add implementation can't be address(0)"
);
uint96 selectorPosition = uint96(
ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors
.length
);
// add new implementation address if it does not exist
if (selectorPosition == 0) {
addFacet(ds, _implementationAddress);
}
for (
uint256 selectorIndex;
selectorIndex < _functionSelectors.length;
selectorIndex++
) {
bytes4 selector = _functionSelectors[selectorIndex];
address oldImplementationAddress = ds
.selectorToImplAndPosition[selector]
.implAddress;
require(
oldImplementationAddress == address(0),
"ParaProxy: Can't add function that already exists"
);
addFunction(ds, selector, selectorPosition, _implementationAddress);
selectorPosition++;
}
}
function replaceFunctions(
address _implementationAddress,
bytes4[] memory _functionSelectors
) internal {
require(
_functionSelectors.length > 0,
"ParaProxy: No selectors in implementation to cut"
);
ProxyStorage storage ds = diamondStorage();
require(
_implementationAddress != address(0),
"ParaProxy: Add implementation can't be address(0)"
);
uint96 selectorPosition = uint96(
ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors
.length
);
// add new implementation address if it does not exist
if (selectorPosition == 0) {
addFacet(ds, _implementationAddress);
}
for (
uint256 selectorIndex;
selectorIndex < _functionSelectors.length;
selectorIndex++
) {
bytes4 selector = _functionSelectors[selectorIndex];
address oldImplementationAddress = ds
.selectorToImplAndPosition[selector]
.implAddress;
require(
oldImplementationAddress != _implementationAddress,
"ParaProxy: Can't replace function with same function"
);
removeFunction(ds, oldImplementationAddress, selector);
addFunction(ds, selector, selectorPosition, _implementationAddress);
selectorPosition++;
}
}
function removeFunctions(
address _implementationAddress,
bytes4[] memory _functionSelectors
) internal {
require(
_functionSelectors.length > 0,
"ParaProxy: No selectors in implementation to cut"
);
ProxyStorage storage ds = diamondStorage();
// if function does not exist then do nothing and return
require(
_implementationAddress == address(0),
"ParaProxy: Remove implementation address must be address(0)"
);
for (
uint256 selectorIndex;
selectorIndex < _functionSelectors.length;
selectorIndex++
) {
bytes4 selector = _functionSelectors[selectorIndex];
address oldImplementationAddress = ds
.selectorToImplAndPosition[selector]
.implAddress;
removeFunction(ds, oldImplementationAddress, selector);
}
}
function addFacet(ProxyStorage storage ds, address _implementationAddress)
internal
{
enforceHasContractCode(
_implementationAddress,
"ParaProxy: New implementation has no code"
);
ds
.implementationFunctionSelectors[_implementationAddress]
.implementationAddressPosition = ds.implementationAddresses.length;
ds.implementationAddresses.push(_implementationAddress);
}
function addFunction(
ProxyStorage storage ds,
bytes4 _selector,
uint96 _selectorPosition,
address _implementationAddress
) internal {
ds
.selectorToImplAndPosition[_selector]
.functionSelectorPosition = _selectorPosition;
ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors
.push(_selector);
ds
.selectorToImplAndPosition[_selector]
.implAddress = _implementationAddress;
}
function removeFunction(
ProxyStorage storage ds,
address _implementationAddress,
bytes4 _selector
) internal {
require(
_implementationAddress != address(0),
"ParaProxy: Can't remove function that doesn't exist"
);
// an immutable function is a function defined directly in a paraProxy
require(
_implementationAddress != address(this),
"ParaProxy: Can't remove immutable function"
);
// replace selector with last selector, then delete last selector
uint256 selectorPosition = ds
.selectorToImplAndPosition[_selector]
.functionSelectorPosition;
uint256 lastSelectorPosition = ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors
.length - 1;
// if not the same then replace _selector with lastSelector
if (selectorPosition != lastSelectorPosition) {
bytes4 lastSelector = ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors[lastSelectorPosition];
ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors[selectorPosition] = lastSelector;
ds
.selectorToImplAndPosition[lastSelector]
.functionSelectorPosition = uint96(selectorPosition);
}
// delete the last selector
ds
.implementationFunctionSelectors[_implementationAddress]
.functionSelectors
.pop();
delete ds.selectorToImplAndPosition[_selector];
// if no more selectors for implementation address then delete the implementation address
if (lastSelectorPosition == 0) {
// replace implementation address with last implementation address and delete last implementation address
uint256 lastImplementationAddressPosition = ds
.implementationAddresses
.length - 1;
uint256 implementationAddressPosition = ds
.implementationFunctionSelectors[_implementationAddress]
.implementationAddressPosition;
if (
implementationAddressPosition !=
lastImplementationAddressPosition
) {
address lastImplementationAddress = ds.implementationAddresses[
lastImplementationAddressPosition
];
ds.implementationAddresses[
implementationAddressPosition
] = lastImplementationAddress;
ds
.implementationFunctionSelectors[lastImplementationAddress]
.implementationAddressPosition = implementationAddressPosition;
}
ds.implementationAddresses.pop();
delete ds
.implementationFunctionSelectors[_implementationAddress]
.implementationAddressPosition;
}
}
function initializeImplementation(address _init, bytes memory _calldata)
internal
{
if (_init == address(0)) {
require(
_calldata.length == 0,
"ParaProxy: _init is address(0) but_calldata is not empty"
);
} else {
require(
_calldata.length > 0,
"ParaProxy: _calldata is empty but _init is not address(0)"
);
if (_init != address(this)) {
enforceHasContractCode(
_init,
"ParaProxy: _init address has no code"
);
}
(bool success, bytes memory error) = _init.delegatecall(_calldata);
if (!success) {
if (error.length > 0) {
// bubble up the error
revert(string(error));
} else {
revert("ParaProxy: _init function reverted");
}
}
}
}
function enforceHasContractCode(
address _contract,
string memory _errorMessage
) internal view {
uint256 contractSize;
assembly {
contractSize := extcodesize(_contract)
}
require(contractSize > 0, _errorMessage);
}
}
[{"inputs":[{"internalType":"address","name":"_contractOwner","type":"address"}],"stateMutability":"payable","type":"constructor"},{"anonymous":false,"inputs":[{"components":[{"internalType":"address","name":"implAddress","type":"address"},{"internalType":"enum IParaProxy.ProxyImplementationAction","name":"action","type":"uint8"},{"internalType":"bytes4[]","name":"functionSelectors","type":"bytes4[]"}],"indexed":false,"internalType":"struct IParaProxy.ProxyImplementation[]","name":"_implementationParams","type":"tuple[]"},{"indexed":false,"internalType":"address","name":"_init","type":"address"},{"indexed":false,"internalType":"bytes","name":"_calldata","type":"bytes"}],"name":"ImplementationUpdated","type":"event"},{"stateMutability":"payable","type":"fallback"},{"inputs":[{"components":[{"internalType":"address","name":"implAddress","type":"address"},{"internalType":"enum IParaProxy.ProxyImplementationAction","name":"action","type":"uint8"},{"internalType":"bytes4[]","name":"functionSelectors","type":"bytes4[]"}],"internalType":"struct IParaProxy.ProxyImplementation[]","name":"_implementationParams","type":"tuple[]"},{"internalType":"address","name":"_init","type":"address"},{"internalType":"bytes","name":"_calldata","type":"bytes"}],"name":"updateImplementation","outputs":[],"stateMutability":"nonpayable","type":"function"},{"stateMutability":"payable","type":"receive"}]
608060405260405162001b5538038062001b558339810160408190526200002691620000db565b6200003c816200004360201b620001af1760201c565b5062000133565b60006200004f620000a5565b6004810180546001600160a01b038581166001600160a01b031983168117909355604051939450169182907f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e090600090a3505050565b600080620000d560017f6337542cd2f84f19034eb9306f0e56d253861ffec87880bde5606f90448356eb6200010d565b92915050565b600060208284031215620000ee57600080fd5b81516001600160a01b03811681146200010657600080fd5b9392505050565b6000828210156200012e57634e487b7160e01b600052601160045260246000fd5b500390565b611a1280620001436000396000f3fe6080604052600436106100225760003560e01c80635a8339911461013457610029565b3661002957005b60008061005760017f6337542cd2f84f19034eb9306f0e56d253861ffec87880bde5606f90448356eb6113e9565b600080357fffffffff00000000000000000000000000000000000000000000000000000000168152602082905260409020549092508291506001600160a01b0316806101105760405162461bcd60e51b815260206004820152602260248201527f5061726150726f78793a2046756e6374696f6e20646f6573206e6f742065786960448201527f737400000000000000000000000000000000000000000000000000000000000060648201526084015b60405180910390fd5b3660008037600080366000845af43d6000803e80801561012f573d6000f35b3d6000fd5b34801561014057600080fd5b5061015461014f366004611465565b610156565b005b61015e61021c565b6101a861016b85876115c9565b8484848080601f0160208091040260200160405190810160405280939291908181526020018383808284376000920191909152506102a792505050565b5050505050565b60006101b96104d1565b6004810180546001600160a01b0385811673ffffffffffffffffffffffffffffffffffffffff1983168117909355604051939450169182907f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e090600090a3505050565b6102246104d1565b600401546001600160a01b031633146102a55760405162461bcd60e51b815260206004820152602160248201527f5061726150726f78793a204d75737420626520636f6e7472616374206f776e6560448201527f72000000000000000000000000000000000000000000000000000000000000006064820152608401610107565b565b60005b83518110156104865760008482815181106102c7576102c7611725565b6020026020010151602001519050600060028111156102e8576102e861173b565b8160028111156102fa576102fa61173b565b14156103495761034485838151811061031557610315611725565b60200260200101516000015186848151811061033357610333611725565b602002602001015160400151610505565b610473565b600181600281111561035d5761035d61173b565b14156103a75761034485838151811061037857610378611725565b60200260200101516000015186848151811061039657610396611725565b60200260200101516040015161080f565b60028160028111156103bb576103bb61173b565b1415610405576103448583815181106103d6576103d6611725565b6020026020010151600001518684815181106103f4576103f4611725565b602002602001015160400151610b2a565b60405162461bcd60e51b815260206004820152602e60248201527f5061726150726f78793a20496e636f72726563742050726f7879496d706c656d60448201527f656e746174696f6e416374696f6e0000000000000000000000000000000000006064820152608401610107565b508061047e81611751565b9150506102aa565b507f7994b9362f6f8b2522d7dfbe2519931ad73d1308b8bcfbc600db6de899c3d5288383836040516104ba939291906117e2565b60405180910390a16104cc8282610cba565b505050565b6000806104ff60017f6337542cd2f84f19034eb9306f0e56d253861ffec87880bde5606f90448356eb6113e9565b92915050565b600081511161057c5760405162461bcd60e51b815260206004820152603060248201527f5061726150726f78793a204e6f2073656c6563746f727320696e20696d706c6560448201527f6d656e746174696f6e20746f20637574000000000000000000000000000000006064820152608401610107565b60006105866104d1565b90506001600160a01b0383166106045760405162461bcd60e51b815260206004820152603160248201527f5061726150726f78793a2041646420696d706c656d656e746174696f6e20636160448201527f6e277420626520616464726573732830290000000000000000000000000000006064820152608401610107565b6001600160a01b03831660009081526001820160205260409020546bffffffffffffffffffffffff811661063c5761063c8285610ede565b60005b83518110156101a857600084828151811061065c5761065c611725565b6020908102919091018101517fffffffff0000000000000000000000000000000000000000000000000000000081166000908152918690526040909120549091506001600160a01b0316801561071a5760405162461bcd60e51b815260206004820152603160248201527f5061726150726f78793a2043616e2774206164642066756e6374696f6e20746860448201527f617420616c7265616479206578697374730000000000000000000000000000006064820152608401610107565b7fffffffff00000000000000000000000000000000000000000000000000000000821660008181526020878152604080832080546001600160a01b03908116740100000000000000000000000000000000000000006bffffffffffffffffffffffff8c16021782558c168085526001808c0185529285208054938401815585528385206008840401805463ffffffff60079095166004026101000a948502191660e08a901c949094029390931790925593909252879052815473ffffffffffffffffffffffffffffffffffffffff1916179055836107f781611917565b9450505050808061080790611751565b91505061063f565b60008151116108865760405162461bcd60e51b815260206004820152603060248201527f5061726150726f78793a204e6f2073656c6563746f727320696e20696d706c6560448201527f6d656e746174696f6e20746f20637574000000000000000000000000000000006064820152608401610107565b60006108906104d1565b90506001600160a01b03831661090e5760405162461bcd60e51b815260206004820152603160248201527f5061726150726f78793a2041646420696d706c656d656e746174696f6e20636160448201527f6e277420626520616464726573732830290000000000000000000000000000006064820152608401610107565b6001600160a01b03831660009081526001820160205260409020546bffffffffffffffffffffffff8116610946576109468285610ede565b60005b83518110156101a857600084828151811061096657610966611725565b6020908102919091018101517fffffffff0000000000000000000000000000000000000000000000000000000081166000908152918690526040909120549091506001600160a01b03908116908716811415610a2a5760405162461bcd60e51b815260206004820152603460248201527f5061726150726f78793a2043616e2774207265706c6163652066756e6374696f60448201527f6e20776974682073616d652066756e6374696f6e0000000000000000000000006064820152608401610107565b610a35858284610f55565b7fffffffff00000000000000000000000000000000000000000000000000000000821660008181526020878152604080832080546001600160a01b03908116740100000000000000000000000000000000000000006bffffffffffffffffffffffff8c16021782558c168085526001808c0185529285208054938401815585528385206008840401805463ffffffff60079095166004026101000a948502191660e08a901c949094029390931790925593909252879052815473ffffffffffffffffffffffffffffffffffffffff191617905583610b1281611917565b94505050508080610b2290611751565b915050610949565b6000815111610ba15760405162461bcd60e51b815260206004820152603060248201527f5061726150726f78793a204e6f2073656c6563746f727320696e20696d706c6560448201527f6d656e746174696f6e20746f20637574000000000000000000000000000000006064820152608401610107565b6000610bab6104d1565b90506001600160a01b03831615610c2a5760405162461bcd60e51b815260206004820152603b60248201527f5061726150726f78793a2052656d6f766520696d706c656d656e746174696f6e60448201527f2061646472657373206d757374206265206164647265737328302900000000006064820152608401610107565b60005b8251811015610cb4576000838281518110610c4a57610c4a611725565b6020908102919091018101517fffffffff0000000000000000000000000000000000000000000000000000000081166000908152918590526040909120549091506001600160a01b0316610c9f848284610f55565b50508080610cac90611751565b915050610c2d565b50505050565b6001600160a01b038216610d4157805115610d3d5760405162461bcd60e51b815260206004820152603860248201527f5061726150726f78793a205f696e69742069732061646472657373283029206260448201527f75745f63616c6c64617461206973206e6f7420656d70747900000000000000006064820152608401610107565b5050565b6000815111610db85760405162461bcd60e51b815260206004820152603960248201527f5061726150726f78793a205f63616c6c6461746120697320656d70747920627560448201527f74205f696e6974206973206e6f742061646472657373283029000000000000006064820152608401610107565b6001600160a01b0382163014610dea57610dea82604051806060016040528060248152602001611990602491396113b2565b600080836001600160a01b031683604051610e059190611943565b600060405180830381855af49150503d8060008114610e40576040519150601f19603f3d011682016040523d82523d6000602084013e610e45565b606091505b509150915081610cb457805115610e70578060405162461bcd60e51b8152600401610107919061195f565b60405162461bcd60e51b815260206004820152602260248201527f5061726150726f78793a205f696e69742066756e6374696f6e2072657665727460448201527f65640000000000000000000000000000000000000000000000000000000000006064820152608401610107565b610f00816040518060600160405280602981526020016119b4602991396113b2565b6002820180546001600160a01b03909216600081815260019485016020908152604082208601859055948401835591825292902001805473ffffffffffffffffffffffffffffffffffffffff19169091179055565b6001600160a01b038216610fd15760405162461bcd60e51b815260206004820152603360248201527f5061726150726f78793a2043616e27742072656d6f76652066756e6374696f6e60448201527f207468617420646f65736e2774206578697374000000000000000000000000006064820152608401610107565b6001600160a01b0382163014156110505760405162461bcd60e51b815260206004820152602a60248201527f5061726150726f78793a2043616e27742072656d6f766520696d6d757461626c60448201527f652066756e6374696f6e000000000000000000000000000000000000000000006064820152608401610107565b7fffffffff000000000000000000000000000000000000000000000000000000008116600090815260208481526040808320546001600160a01b0386168452600180880190935290832054740100000000000000000000000000000000000000009091046bffffffffffffffffffffffff1692916110cd916113e9565b90508082146111ed576001600160a01b0384166000908152600186016020526040812080548390811061110257611102611725565b600091825260208083206008830401546001600160a01b038916845260018a019091526040909220805460079092166004026101000a90920460e01b92508291908590811061115357611153611725565b600091825260208083206008830401805463ffffffff60079094166004026101000a938402191660e09590951c929092029390931790557fffffffff000000000000000000000000000000000000000000000000000000009290921682528690526040902080546001600160a01b0316740100000000000000000000000000000000000000006bffffffffffffffffffffffff8516021790555b6001600160a01b0384166000908152600186016020526040902080548061121657611216611979565b60008281526020808220600860001990940193840401805463ffffffff600460078716026101000a0219169055919092557fffffffff00000000000000000000000000000000000000000000000000000000851682528690526040812055806101a857600285015460009061128d906001906113e9565b6001600160a01b03861660009081526001808901602052604090912001549091508082146113495760008760020183815481106112cc576112cc611725565b6000918252602090912001546002890180546001600160a01b0390921692508291849081106112fd576112fd611725565b6000918252602080832091909101805473ffffffffffffffffffffffffffffffffffffffff19166001600160a01b03948516179055929091168152600189810190925260409020018190555b8660020180548061135c5761135c611979565b600082815260208082208301600019908101805473ffffffffffffffffffffffffffffffffffffffff191690559092019092556001600160a01b0388168252600189810190915260408220015550505050505050565b813b8181610cb45760405162461bcd60e51b8152600401610107919061195f565b634e487b7160e01b600052601160045260246000fd5b6000828210156113fb576113fb6113d3565b500390565b80356001600160a01b038116811461141757600080fd5b919050565b60008083601f84011261142e57600080fd5b50813567ffffffffffffffff81111561144657600080fd5b60208301915083602082850101111561145e57600080fd5b9250929050565b60008060008060006060868803121561147d57600080fd5b853567ffffffffffffffff8082111561149557600080fd5b818801915088601f8301126114a957600080fd5b8135818111156114b857600080fd5b8960208260051b85010111156114cd57600080fd5b602083019750809650506114e360208901611400565b945060408801359150808211156114f957600080fd5b506115068882890161141c565b969995985093965092949392505050565b634e487b7160e01b600052604160045260246000fd5b6040516060810167ffffffffffffffff8111828210171561155057611550611517565b60405290565b604051601f82017fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffe016810167ffffffffffffffff8111828210171561159d5761159d611517565b604052919050565b600067ffffffffffffffff8211156115bf576115bf611517565b5060051b60200190565b60006115dc6115d7846115a5565b611556565b83815260208082019190600586811b8601368111156115fa57600080fd5b865b8181101561171857803567ffffffffffffffff8082111561161d5760008081fd5b818a019150606082360312156116335760008081fd5b61163b61152d565b61164483611400565b815286830135600381106116585760008081fd5b81880152604083810135838111156116705760008081fd5b939093019236601f85011261168757600092508283fd5b833592506116976115d7846115a5565b83815292871b840188019288810190368511156116b45760008081fd5b948901945b848610156117015785357fffffffff00000000000000000000000000000000000000000000000000000000811681146116f25760008081fd5b825294890194908901906116b9565b9183019190915250885250509483019483016115fc565b5092979650505050505050565b634e487b7160e01b600052603260045260246000fd5b634e487b7160e01b600052602160045260246000fd5b6000600019821415611765576117656113d3565b5060010190565b60005b8381101561178757818101518382015260200161176f565b83811115610cb45750506000910152565b600081518084526117b081602086016020860161176c565b601f017fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffe0169290920160200192915050565b60006060808301818452808751808352608092508286019150828160051b8701016020808b0160005b848110156118e7577fffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffff808a850301865281518885016001600160a01b038251168652848201516003811061186e57634e487b7160e01b600052602160045260246000fd5b868601526040918201519186018a905281519081905290840190600090898701905b808310156118d25783517fffffffff00000000000000000000000000000000000000000000000000000000168252928601926001929092019190860190611890565b5097850197955050509082019060010161180b565b50506001600160a01b038a169088015286810360408801526119098189611798565b9a9950505050505050505050565b60006bffffffffffffffffffffffff80831681811415611939576119396113d3565b6001019392505050565b6000825161195581846020870161176c565b9190910192915050565b6020815260006119726020830184611798565b9392505050565b634e487b7160e01b600052603160045260246000fdfe5061726150726f78793a205f696e6974206164647265737320686173206e6f20636f64655061726150726f78793a204e657720696d706c656d656e746174696f6e20686173206e6f20636f6465a26469706673582212209e02e0420062cc1933af5fa6b98e3d7f363c1c4aa9720e23bb57b65edc892f1864736f6c634300080a00330000000000000000000000006cd30e716adbe47dadf7319f6f2fb83d507c857d
Checkout more smart contracts
- Verified
- Fungible Token
- ERC20
- Verified

SHILAINU
The following smart contract is the SHILAINU token contract, which is an ERC20 token with a total supply of 1 trillion. It includes features such as transaction limits, fees, and automatic liquidity provision. The contract also has a blacklist mode and the ability to set fee and transaction exemptions for specific addresses. The purpose of the contract is to provide a decentralized currency for the Shiba Inu community.

Token
The following smart contract is a token contract that implements the ERC20 standard. It includes features such as a fee system, wallet and transaction limits, and liquidity provision. The contract also allows for the destruction of tokens through a fee system. The contract is designed to be used with the Uniswap decentralized exchange.
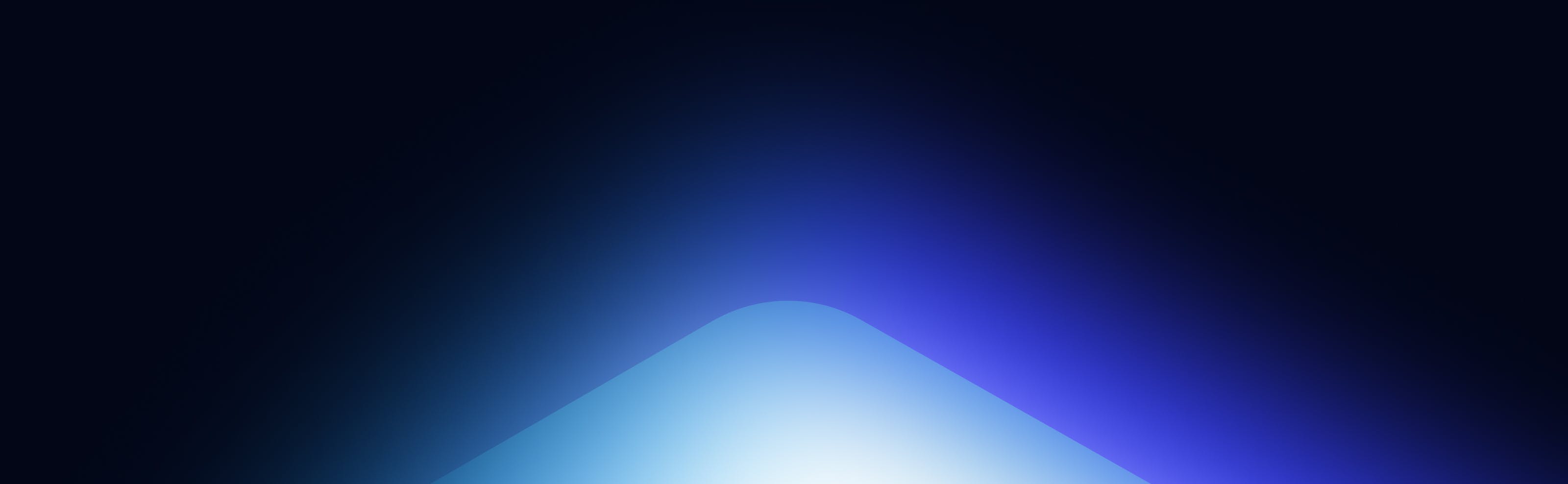