- Verified
The following smart contract is called SwftSwap and it allows users to swap tokens and ETH. It uses OpenZeppelin libraries for access control, reentrancy protection, and safe math operations. The contract has functions for swapping tokens and ETH, withdrawing tokens and ETH, and receiving ETH. The purpose of the contract is to provide a secure and efficient way for users to swap tokens and ETH.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/security/ReentrancyGuard.sol";
import "@openzeppelin/contracts/utils/math/SafeMath.sol";
import "./lib/TransferHelper.sol";
/// @notice swftswap
contract SwftSwap is ReentrancyGuard, Ownable {
using SafeMath for uint256;
string public name;
string public symbol;
/// @notice Swap's log.
/// @param fromToken token's address.
/// @param toToken ������������������������������������������������������������������������������������������������������������'usdt(matic)'
/// @param sender Who swap
/// @param destination ������������������������������������������������������
/// @param fromAmount Input amount.
/// @param minReturnAmount ���������������������������������������������������������������������������������������������������������������������������������������
event Swap(
address fromToken,
string toToken,
address sender,
string destination,
uint256 fromAmount,
uint256 minReturnAmount
);
/// @notice SwapEth's log.
/// @param toToken ������������������������������������������������������������������������������������������������������������'usdt(matic)'
/// @param sender Who swap
/// @param destination ������������������������������������������������������
/// @param fromAmount Input amount.
/// @param minReturnAmount ���������������������������������������������������������������������������������������������������������������������������������������
event SwapEth(
string toToken,
address sender,
string destination,
uint256 fromAmount,
uint256 minReturnAmount
);
event WithdrawETH(uint256 amount);
event Withdtraw(address token, uint256 amount);
constructor() {
name = "SWFT Swap1.1";
symbol = "SSwap";
}
/// @notice Excute transactions. ������������������������������������������������������������������������������������������������������������
/// @param fromToken token's address. ���������������������������������������������������������������
/// @param toToken ���������������������������������������������������������������������������������'usdt(matic)'
/// @param destination ������������������������������������������������������������������������
/// @param fromAmount ���������������������������������������������
/// @param minReturnAmount ���������������������������������������������������������������������������������������������������������������������������������������
function swap(
address fromToken,
string memory toToken,
string memory destination,
uint256 fromAmount,
uint256 minReturnAmount
) external nonReentrant {
require(fromToken != address(0), "FROMTOKEN_CANT_T_BE_0"); // ���������������������������������������������������������������0
require(fromAmount > 0, "FROM_TOKEN_AMOUNT_MUST_BE_MORE_THAN_0");
uint256 _inputAmount; // ������������������������������������������������������������������������������������������
uint256 _fromTokenBalanceOrigin = IERC20(fromToken).balanceOf(address(this));
TransferHelper.safeTransferFrom(fromToken, msg.sender, address(this), fromAmount);
uint256 _fromTokenBalanceNew = IERC20(fromToken).balanceOf(address(this));
_inputAmount = _fromTokenBalanceNew.sub(_fromTokenBalanceOrigin);
require(_inputAmount > 0, "NO_FROM_TOKEN_TRANSFER_TO_THIS_CONTRACT");
emit Swap(fromToken, toToken, msg.sender, destination, fromAmount, minReturnAmount);
}
/// @notice Excute transactions. ������������������������������������������������������������������������������������������������������������
/// @param toToken ���������������������������������������������������������������������������������'usdt(matic)'
/// @param destination ������������������������������������������������������������������������
/// @param minReturnAmount ���������������������������������������������������������������������������������������������������������������������������������������
function swapEth(string memory toToken, string memory destination, uint256 minReturnAmount
) external payable nonReentrant {
uint256 _ethAmount = msg.value; // ���������������������������������������������eth���������������������������
require(_ethAmount > 0, "ETH_AMOUNT_MUST_BE_MORE_THAN_0");
emit SwapEth(toToken, msg.sender, destination, _ethAmount, minReturnAmount);
}
function withdrawETH(address destination, uint256 amount) external onlyOwner {
require(destination != address(0), "DESTINATION_CANNT_BE_0_ADDRESS");
uint256 balance = address(this).balance;
require(balance >= amount, "AMOUNT_CANNT_MORE_THAN_BALANCE");
TransferHelper.safeTransferETH(destination, amount);
emit WithdrawETH(amount);
}
function withdraw(address token, address destination, uint256 amount) external onlyOwner {
require(destination != address(0), "DESTINATION_CANNT_BE_0_ADDRESS");
require(token != address(0), "TOKEN_MUST_NOT_BE_0");
uint256 balance = IERC20(token).balanceOf(address(this));
require(balance >= amount, "AMOUNT_CANNT_MORE_THAN_BALANCE");
TransferHelper.safeTransfer(token, destination, amount);
emit Withdtraw(token, amount);
}
receive() external payable {}
}
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
library TransferHelper {
function safeApprove(address token, address to, uint value) internal {
// bytes4(keccak256(bytes('approve(address,uint256)')));
(bool success, bytes memory data) = token.call(abi.encodeWithSelector(0x095ea7b3, to, value));
require(success && (data.length == 0 || abi.decode(data, (bool))), 'TransferHelper: APPROVE_FAILED');
}
function safeTransfer(address token, address to, uint value) internal {
// bytes4(keccak256(bytes('transfer(address,uint256)')));
(bool success, bytes memory data) = token.call(abi.encodeWithSelector(0xa9059cbb, to, value));
require(success && (data.length == 0 || abi.decode(data, (bool))), 'TransferHelper: TRANSFER_FAILED');
}
function safeTransferFrom(address token, address from, address to, uint value) internal {
// bytes4(keccak256(bytes('transferFrom(address,address,uint256)')));
(bool success, bytes memory data) = token.call(abi.encodeWithSelector(0x23b872dd, from, to, value));
require(success && (data.length == 0 || abi.decode(data, (bool))), 'TransferHelper: TRANSFER_FROM_FAILED');
}
function safeTransferETH(address to, uint value) internal {
(bool success,) = to.call{value:value}(new bytes(0));
require(success, 'TransferHelper: ETH_TRANSFER_FAILED');
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
// CAUTION
// This version of SafeMath should only be used with Solidity 0.8 or later,
// because it relies on the compiler's built in overflow checks.
/**
* @dev Wrappers over Solidity's arithmetic operations.
*
* NOTE: `SafeMath` is no longer needed starting with Solidity 0.8. The compiler
* now has built in overflow checking.
*/
library SafeMath {
/**
* @dev Returns the addition of two unsigned integers, with an overflow flag.
*
* _Available since v3.4._
*/
function tryAdd(uint256 a, uint256 b) internal pure returns (bool, uint256) {
unchecked {
uint256 c = a + b;
if (c < a) return (false, 0);
return (true, c);
}
}
/**
* @dev Returns the substraction of two unsigned integers, with an overflow flag.
*
* _Available since v3.4._
*/
function trySub(uint256 a, uint256 b) internal pure returns (bool, uint256) {
unchecked {
if (b > a) return (false, 0);
return (true, a - b);
}
}
/**
* @dev Returns the multiplication of two unsigned integers, with an overflow flag.
*
* _Available since v3.4._
*/
function tryMul(uint256 a, uint256 b) internal pure returns (bool, uint256) {
unchecked {
// Gas optimization: this is cheaper than requiring 'a' not being zero, but the
// benefit is lost if 'b' is also tested.
// See: https://github.com/OpenZeppelin/openzeppelin-contracts/pull/522
if (a == 0) return (true, 0);
uint256 c = a * b;
if (c / a != b) return (false, 0);
return (true, c);
}
}
/**
* @dev Returns the division of two unsigned integers, with a division by zero flag.
*
* _Available since v3.4._
*/
function tryDiv(uint256 a, uint256 b) internal pure returns (bool, uint256) {
unchecked {
if (b == 0) return (false, 0);
return (true, a / b);
}
}
/**
* @dev Returns the remainder of dividing two unsigned integers, with a division by zero flag.
*
* _Available since v3.4._
*/
function tryMod(uint256 a, uint256 b) internal pure returns (bool, uint256) {
unchecked {
if (b == 0) return (false, 0);
return (true, a % b);
}
}
/**
* @dev Returns the addition of two unsigned integers, reverting on
* overflow.
*
* Counterpart to Solidity's `+` operator.
*
* Requirements:
*
* - Addition cannot overflow.
*/
function add(uint256 a, uint256 b) internal pure returns (uint256) {
return a + b;
}
/**
* @dev Returns the subtraction of two unsigned integers, reverting on
* overflow (when the result is negative).
*
* Counterpart to Solidity's `-` operator.
*
* Requirements:
*
* - Subtraction cannot overflow.
*/
function sub(uint256 a, uint256 b) internal pure returns (uint256) {
return a - b;
}
/**
* @dev Returns the multiplication of two unsigned integers, reverting on
* overflow.
*
* Counterpart to Solidity's `*` operator.
*
* Requirements:
*
* - Multiplication cannot overflow.
*/
function mul(uint256 a, uint256 b) internal pure returns (uint256) {
return a * b;
}
/**
* @dev Returns the integer division of two unsigned integers, reverting on
* division by zero. The result is rounded towards zero.
*
* Counterpart to Solidity's `/` operator.
*
* Requirements:
*
* - The divisor cannot be zero.
*/
function div(uint256 a, uint256 b) internal pure returns (uint256) {
return a / b;
}
/**
* @dev Returns the remainder of dividing two unsigned integers. (unsigned integer modulo),
* reverting when dividing by zero.
*
* Counterpart to Solidity's `%` operator. This function uses a `revert`
* opcode (which leaves remaining gas untouched) while Solidity uses an
* invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
*
* - The divisor cannot be zero.
*/
function mod(uint256 a, uint256 b) internal pure returns (uint256) {
return a % b;
}
/**
* @dev Returns the subtraction of two unsigned integers, reverting with custom message on
* overflow (when the result is negative).
*
* CAUTION: This function is deprecated because it requires allocating memory for the error
* message unnecessarily. For custom revert reasons use {trySub}.
*
* Counterpart to Solidity's `-` operator.
*
* Requirements:
*
* - Subtraction cannot overflow.
*/
function sub(
uint256 a,
uint256 b,
string memory errorMessage
) internal pure returns (uint256) {
unchecked {
require(b <= a, errorMessage);
return a - b;
}
}
/**
* @dev Returns the integer division of two unsigned integers, reverting with custom message on
* division by zero. The result is rounded towards zero.
*
* Counterpart to Solidity's `/` operator. Note: this function uses a
* `revert` opcode (which leaves remaining gas untouched) while Solidity
* uses an invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
*
* - The divisor cannot be zero.
*/
function div(
uint256 a,
uint256 b,
string memory errorMessage
) internal pure returns (uint256) {
unchecked {
require(b > 0, errorMessage);
return a / b;
}
}
/**
* @dev Returns the remainder of dividing two unsigned integers. (unsigned integer modulo),
* reverting with custom message when dividing by zero.
*
* CAUTION: This function is deprecated because it requires allocating memory for the error
* message unnecessarily. For custom revert reasons use {tryMod}.
*
* Counterpart to Solidity's `%` operator. This function uses a `revert`
* opcode (which leaves remaining gas untouched) while Solidity uses an
* invalid opcode to revert (consuming all remaining gas).
*
* Requirements:
*
* - The divisor cannot be zero.
*/
function mod(
uint256 a,
uint256 b,
string memory errorMessage
) internal pure returns (uint256) {
unchecked {
require(b > 0, errorMessage);
return a % b;
}
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
/**
* @dev Provides information about the current execution context, including the
* sender of the transaction and its data. While these are generally available
* via msg.sender and msg.data, they should not be accessed in such a direct
* manner, since when dealing with meta-transactions the account sending and
* paying for execution may not be the actual sender (as far as an application
* is concerned).
*
* This contract is only required for intermediate, library-like contracts.
*/
abstract contract Context {
function _msgSender() internal view virtual returns (address) {
return msg.sender;
}
function _msgData() internal view virtual returns (bytes calldata) {
return msg.data;
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
/**
* @dev Interface of the ERC20 standard as defined in the EIP.
*/
interface IERC20 {
/**
* @dev Returns the amount of tokens in existence.
*/
function totalSupply() external view returns (uint256);
/**
* @dev Returns the amount of tokens owned by `account`.
*/
function balanceOf(address account) external view returns (uint256);
/**
* @dev Moves `amount` tokens from the caller's account to `recipient`.
*
* Returns a boolean value indicating whether the operation succeeded.
*
* Emits a {Transfer} event.
*/
function transfer(address recipient, uint256 amount) external returns (bool);
/**
* @dev Returns the remaining number of tokens that `spender` will be
* allowed to spend on behalf of `owner` through {transferFrom}. This is
* zero by default.
*
* This value changes when {approve} or {transferFrom} are called.
*/
function allowance(address owner, address spender) external view returns (uint256);
/**
* @dev Sets `amount` as the allowance of `spender` over the caller's tokens.
*
* Returns a boolean value indicating whether the operation succeeded.
*
* IMPORTANT: Beware that changing an allowance with this method brings the risk
* that someone may use both the old and the new allowance by unfortunate
* transaction ordering. One possible solution to mitigate this race
* condition is to first reduce the spender's allowance to 0 and set the
* desired value afterwards:
* https://github.com/ethereum/EIPs/issues/20#issuecomment-263524729
*
* Emits an {Approval} event.
*/
function approve(address spender, uint256 amount) external returns (bool);
/**
* @dev Moves `amount` tokens from `sender` to `recipient` using the
* allowance mechanism. `amount` is then deducted from the caller's
* allowance.
*
* Returns a boolean value indicating whether the operation succeeded.
*
* Emits a {Transfer} event.
*/
function transferFrom(
address sender,
address recipient,
uint256 amount
) external returns (bool);
/**
* @dev Emitted when `value` tokens are moved from one account (`from`) to
* another (`to`).
*
* Note that `value` may be zero.
*/
event Transfer(address indexed from, address indexed to, uint256 value);
/**
* @dev Emitted when the allowance of a `spender` for an `owner` is set by
* a call to {approve}. `value` is the new allowance.
*/
event Approval(address indexed owner, address indexed spender, uint256 value);
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
/**
* @dev Contract module that helps prevent reentrant calls to a function.
*
* Inheriting from `ReentrancyGuard` will make the {nonReentrant} modifier
* available, which can be applied to functions to make sure there are no nested
* (reentrant) calls to them.
*
* Note that because there is a single `nonReentrant` guard, functions marked as
* `nonReentrant` may not call one another. This can be worked around by making
* those functions `private`, and then adding `external` `nonReentrant` entry
* points to them.
*
* TIP: If you would like to learn more about reentrancy and alternative ways
* to protect against it, check out our blog post
* https://blog.openzeppelin.com/reentrancy-after-istanbul/[Reentrancy After Istanbul].
*/
abstract contract ReentrancyGuard {
// Booleans are more expensive than uint256 or any type that takes up a full
// word because each write operation emits an extra SLOAD to first read the
// slot's contents, replace the bits taken up by the boolean, and then write
// back. This is the compiler's defense against contract upgrades and
// pointer aliasing, and it cannot be disabled.
// The values being non-zero value makes deployment a bit more expensive,
// but in exchange the refund on every call to nonReentrant will be lower in
// amount. Since refunds are capped to a percentage of the total
// transaction's gas, it is best to keep them low in cases like this one, to
// increase the likelihood of the full refund coming into effect.
uint256 private constant _NOT_ENTERED = 1;
uint256 private constant _ENTERED = 2;
uint256 private _status;
constructor() {
_status = _NOT_ENTERED;
}
/**
* @dev Prevents a contract from calling itself, directly or indirectly.
* Calling a `nonReentrant` function from another `nonReentrant`
* function is not supported. It is possible to prevent this from happening
* by making the `nonReentrant` function external, and make it call a
* `private` function that does the actual work.
*/
modifier nonReentrant() {
// On the first call to nonReentrant, _notEntered will be true
require(_status != _ENTERED, "ReentrancyGuard: reentrant call");
// Any calls to nonReentrant after this point will fail
_status = _ENTERED;
_;
// By storing the original value once again, a refund is triggered (see
// https://eips.ethereum.org/EIPS/eip-2200)
_status = _NOT_ENTERED;
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "../utils/Context.sol";
/**
* @dev Contract module which provides a basic access control mechanism, where
* there is an account (an owner) that can be granted exclusive access to
* specific functions.
*
* By default, the owner account will be the one that deploys the contract. This
* can later be changed with {transferOwnership}.
*
* This module is used through inheritance. It will make available the modifier
* `onlyOwner`, which can be applied to your functions to restrict their use to
* the owner.
*/
abstract contract Ownable is Context {
address private _owner;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
/**
* @dev Initializes the contract setting the deployer as the initial owner.
*/
constructor() {
_setOwner(_msgSender());
}
/**
* @dev Returns the address of the current owner.
*/
function owner() public view virtual returns (address) {
return _owner;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(owner() == _msgSender(), "Ownable: caller is not the owner");
_;
}
/**
* @dev Leaves the contract without owner. It will not be possible to call
* `onlyOwner` functions anymore. Can only be called by the current owner.
*
* NOTE: Renouncing ownership will leave the contract without an owner,
* thereby removing any functionality that is only available to the owner.
*/
function renounceOwnership() public virtual onlyOwner {
_setOwner(address(0));
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`).
* Can only be called by the current owner.
*/
function transferOwnership(address newOwner) public virtual onlyOwner {
require(newOwner != address(0), "Ownable: new owner is the zero address");
_setOwner(newOwner);
}
function _setOwner(address newOwner) private {
address oldOwner = _owner;
_owner = newOwner;
emit OwnershipTransferred(oldOwner, newOwner);
}
}
[{"inputs":[],"stateMutability":"nonpayable","type":"constructor"},{"anonymous":false,"inputs":[{"indexed":true,"internalType":"address","name":"previousOwner","type":"address"},{"indexed":true,"internalType":"address","name":"newOwner","type":"address"}],"name":"OwnershipTransferred","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"address","name":"fromToken","type":"address"},{"indexed":false,"internalType":"string","name":"toToken","type":"string"},{"indexed":false,"internalType":"address","name":"sender","type":"address"},{"indexed":false,"internalType":"string","name":"destination","type":"string"},{"indexed":false,"internalType":"uint256","name":"fromAmount","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"minReturnAmount","type":"uint256"}],"name":"Swap","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"string","name":"toToken","type":"string"},{"indexed":false,"internalType":"address","name":"sender","type":"address"},{"indexed":false,"internalType":"string","name":"destination","type":"string"},{"indexed":false,"internalType":"uint256","name":"fromAmount","type":"uint256"},{"indexed":false,"internalType":"uint256","name":"minReturnAmount","type":"uint256"}],"name":"SwapEth","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"uint256","name":"amount","type":"uint256"}],"name":"WithdrawETH","type":"event"},{"anonymous":false,"inputs":[{"indexed":false,"internalType":"address","name":"token","type":"address"},{"indexed":false,"internalType":"uint256","name":"amount","type":"uint256"}],"name":"Withdtraw","type":"event"},{"inputs":[],"name":"name","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"owner","outputs":[{"internalType":"address","name":"","type":"address"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"renounceOwnership","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"fromToken","type":"address"},{"internalType":"string","name":"toToken","type":"string"},{"internalType":"string","name":"destination","type":"string"},{"internalType":"uint256","name":"fromAmount","type":"uint256"},{"internalType":"uint256","name":"minReturnAmount","type":"uint256"}],"name":"swap","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"string","name":"toToken","type":"string"},{"internalType":"string","name":"destination","type":"string"},{"internalType":"uint256","name":"minReturnAmount","type":"uint256"}],"name":"swapEth","outputs":[],"stateMutability":"payable","type":"function"},{"inputs":[],"name":"symbol","outputs":[{"internalType":"string","name":"","type":"string"}],"stateMutability":"view","type":"function"},{"inputs":[{"internalType":"address","name":"newOwner","type":"address"}],"name":"transferOwnership","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"token","type":"address"},{"internalType":"address","name":"destination","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"withdraw","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"destination","type":"address"},{"internalType":"uint256","name":"amount","type":"uint256"}],"name":"withdrawETH","outputs":[],"stateMutability":"nonpayable","type":"function"},{"stateMutability":"payable","type":"receive"}]
60806040523480156200001157600080fd5b5060016000556200002b6200002562000096565b6200009a565b60408051808201909152600c8082526b535746542053776170312e3160a01b60209092019182526200006091600291620000ec565b5060408051808201909152600580825264053537761760dc1b60209092019182526200008f91600391620000ec565b50620001cf565b3390565b600180546001600160a01b038381166001600160a01b0319831681179093556040519116919082907f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e090600090a35050565b828054620000fa9062000192565b90600052602060002090601f0160209004810192826200011e576000855562000169565b82601f106200013957805160ff191683800117855562000169565b8280016001018555821562000169579182015b82811115620001695782518255916020019190600101906200014c565b50620001779291506200017b565b5090565b5b808211156200017757600081556001016200017c565b600281046001821680620001a757607f821691505b60208210811415620001c957634e487b7160e01b600052602260045260246000fd5b50919050565b61121b80620001df6000396000f3fe60806040526004361061008a5760003560e01c80638da5cb5b116100595780638da5cb5b1461010b57806395d89b411461012d5780639ddf93bb14610142578063d9caed1214610162578063f2fde38b1461018257610091565b806306fdde031461009657806316b3b4c2146100c15780634782f779146100d6578063715018a6146100f657610091565b3661009157005b600080fd5b3480156100a257600080fd5b506100ab6101a2565b6040516100b89190610ddf565b60405180910390f35b6100d46100cf366004610c71565b610230565b005b3480156100e257600080fd5b506100d46100f1366004610c28565b6102c9565b34801561010257600080fd5b506100d4610395565b34801561011757600080fd5b506101206103e0565b6040516100b89190610d3b565b34801561013957600080fd5b506100ab6103ef565b34801561014e57600080fd5b506100d461015d366004610ba5565b6103fc565b34801561016e57600080fd5b506100d461017d366004610b6a565b6105f4565b34801561018e57600080fd5b506100d461019d366004610b50565b61076a565b600280546101af90611194565b80601f01602080910402602001604051908101604052809291908181526020018280546101db90611194565b80156102285780601f106101fd57610100808354040283529160200191610228565b820191906000526020600020905b81548152906001019060200180831161020b57829003601f168201915b505050505081565b6002600054141561025c5760405162461bcd60e51b815260040161025390611101565b60405180910390fd5b6002600055348061027f5760405162461bcd60e51b815260040161025390610f34565b7f4e96fb90a89341a56db7ad2bbf04c715bbf20be6a9a9e764671f718c4697649a84338584866040516102b6959493929190610df2565b60405180910390a1505060016000555050565b6102d16107db565b6001600160a01b03166102e26103e0565b6001600160a01b0316146103085760405162461bcd60e51b815260040161025390610fe9565b6001600160a01b03821661032e5760405162461bcd60e51b815260040161025390610f6b565b478181101561034f5760405162461bcd60e51b815260040161025390610eb7565b61035983836107df565b7f94effa14ea3a1ef396fa2fd829336d1597f1d76b548c26bfa2332869706638af826040516103889190611138565b60405180910390a1505050565b61039d6107db565b6001600160a01b03166103ae6103e0565b6001600160a01b0316146103d45760405162461bcd60e51b815260040161025390610fe9565b6103de6000610871565b565b6001546001600160a01b031690565b600380546101af90611194565b6002600054141561041f5760405162461bcd60e51b815260040161025390611101565b60026000556001600160a01b03851661044a5760405162461bcd60e51b81526004016102539061104b565b6000821161046a5760405162461bcd60e51b815260040161025390610e72565b600080866001600160a01b03166370a08231306040518263ffffffff1660e01b81526004016104999190610d3b565b60206040518083038186803b1580156104b157600080fd5b505afa1580156104c5573d6000803e3d6000fd5b505050506040513d601f19601f820116820180604052508101906104e99190610cdb565b90506104f7873330876108c3565b6040516370a0823160e01b81526000906001600160a01b038916906370a0823190610526903090600401610d3b565b60206040518083038186803b15801561053e57600080fd5b505afa158015610552573d6000803e3d6000fd5b505050506040513d601f19601f820116820180604052508101906105769190610cdb565b905061058281836109b3565b9250600083116105a45760405162461bcd60e51b815260040161025390610fa2565b7f45f377f845e1cc76ae2c08f990e15d58bcb732db46f92a4852b956580c3a162f8888338989896040516105dd96959493929190610d73565b60405180910390a150506001600055505050505050565b6105fc6107db565b6001600160a01b031661060d6103e0565b6001600160a01b0316146106335760405162461bcd60e51b815260040161025390610fe9565b6001600160a01b0382166106595760405162461bcd60e51b815260040161025390610f6b565b6001600160a01b03831661067f5760405162461bcd60e51b81526004016102539061101e565b6040516370a0823160e01b81526000906001600160a01b038516906370a08231906106ae903090600401610d3b565b60206040518083038186803b1580156106c657600080fd5b505afa1580156106da573d6000803e3d6000fd5b505050506040513d601f19601f820116820180604052508101906106fe9190610cdb565b9050818110156107205760405162461bcd60e51b815260040161025390610eb7565b61072b8484846109c6565b7f7bf0873174a9cc6b28e039b52e74903dd59d650205f32748e3c3dd6b9918ea87848360405161075c929190610dc6565b60405180910390a150505050565b6107726107db565b6001600160a01b03166107836103e0565b6001600160a01b0316146107a95760405162461bcd60e51b815260040161025390610fe9565b6001600160a01b0381166107cf5760405162461bcd60e51b815260040161025390610eee565b6107d881610871565b50565b3390565b604080516000808252602082019092526001600160a01b0384169083906040516108099190610d1f565b60006040518083038185875af1925050503d8060008114610846576040519150601f19603f3d011682016040523d82523d6000602084013e61084b565b606091505b505090508061086c5760405162461bcd60e51b81526004016102539061107a565b505050565b600180546001600160a01b038381166001600160a01b0319831681179093556040519116919082907f8be0079c531659141344cd1fd0a4f28419497f9722a3daafe3b4186f6b6457e090600090a35050565b600080856001600160a01b03166323b872dd8686866040516024016108ea93929190610d4f565b6040516020818303038152906040529060e01b6020820180516001600160e01b0383818316178352505050506040516109239190610d1f565b6000604051808303816000865af19150503d8060008114610960576040519150601f19603f3d011682016040523d82523d6000602084013e610965565b606091505b509150915081801561098f57508051158061098f57508080602001905181019061098f9190610c51565b6109ab5760405162461bcd60e51b8152600401610253906110bd565b505050505050565b60006109bf8284611141565b9392505050565b600080846001600160a01b031663a9059cbb85856040516024016109eb929190610dc6565b6040516020818303038152906040529060e01b6020820180516001600160e01b038381831617835250505050604051610a249190610d1f565b6000604051808303816000865af19150503d8060008114610a61576040519150601f19603f3d011682016040523d82523d6000602084013e610a66565b606091505b5091509150818015610a90575080511580610a90575080806020019051810190610a909190610c51565b610aac5760405162461bcd60e51b815260040161025390610e3b565b5050505050565b80356001600160a01b0381168114610aca57600080fd5b919050565b600082601f830112610adf578081fd5b813567ffffffffffffffff80821115610afa57610afa6111cf565b604051601f8301601f191681016020018281118282101715610b1e57610b1e6111cf565b604052828152848301602001861015610b35578384fd5b82602086016020830137918201602001929092529392505050565b600060208284031215610b61578081fd5b6109bf82610ab3565b600080600060608486031215610b7e578182fd5b610b8784610ab3565b9250610b9560208501610ab3565b9150604084013590509250925092565b600080600080600060a08688031215610bbc578081fd5b610bc586610ab3565b9450602086013567ffffffffffffffff80821115610be1578283fd5b610bed89838a01610acf565b95506040880135915080821115610c02578283fd5b50610c0f88828901610acf565b9598949750949560608101359550608001359392505050565b60008060408385031215610c3a578182fd5b610c4383610ab3565b946020939093013593505050565b600060208284031215610c62578081fd5b815180151581146109bf578182fd5b600080600060608486031215610c85578283fd5b833567ffffffffffffffff80821115610c9c578485fd5b610ca887838801610acf565b94506020860135915080821115610cbd578384fd5b50610cca86828701610acf565b925050604084013590509250925092565b600060208284031215610cec578081fd5b5051919050565b60008151808452610d0b816020860160208601611164565b601f01601f19169290920160200192915050565b60008251610d31818460208701611164565b9190910192915050565b6001600160a01b0391909116815260200190565b6001600160a01b039384168152919092166020820152604081019190915260600190565b600060018060a01b03808916835260c06020840152610d9560c0840189610cf3565b81881660408501528381036060850152610daf8188610cf3565b608085019690965250505060a00152949350505050565b6001600160a01b03929092168252602082015260400190565b6000602082526109bf6020830184610cf3565b600060a08252610e0560a0830188610cf3565b6001600160a01b03871660208401528281036040840152610e268187610cf3565b60608401959095525050608001529392505050565b6020808252601f908201527f5472616e7366657248656c7065723a205452414e534645525f4641494c454400604082015260600190565b60208082526025908201527f46524f4d5f544f4b454e5f414d4f554e545f4d5553545f42455f4d4f52455f54604082015264048414e5f360dc1b606082015260800190565b6020808252601e908201527f414d4f554e545f43414e4e545f4d4f52455f5448414e5f42414c414e43450000604082015260600190565b60208082526026908201527f4f776e61626c653a206e6577206f776e657220697320746865207a65726f206160408201526564647265737360d01b606082015260800190565b6020808252601e908201527f4554485f414d4f554e545f4d5553545f42455f4d4f52455f5448414e5f300000604082015260600190565b6020808252601e908201527f44455354494e4154494f4e5f43414e4e545f42455f305f414444524553530000604082015260600190565b60208082526027908201527f4e4f5f46524f4d5f544f4b454e5f5452414e534645525f544f5f544849535f4360408201526613d395149050d560ca1b606082015260800190565b6020808252818101527f4f776e61626c653a2063616c6c6572206973206e6f7420746865206f776e6572604082015260600190565b6020808252601390820152720544f4b454e5f4d5553545f4e4f545f42455f3606c1b604082015260600190565b602080825260159082015274046524f4d544f4b454e5f43414e545f545f42455f3605c1b604082015260600190565b60208082526023908201527f5472616e7366657248656c7065723a204554485f5452414e534645525f46414960408201526213115160ea1b606082015260800190565b60208082526024908201527f5472616e7366657248656c7065723a205452414e534645525f46524f4d5f46416040820152631253115160e21b606082015260800190565b6020808252601f908201527f5265656e7472616e637947756172643a207265656e7472616e742063616c6c00604082015260600190565b90815260200190565b60008282101561115f57634e487b7160e01b81526011600452602481fd5b500390565b60005b8381101561117f578181015183820152602001611167565b8381111561118e576000848401525b50505050565b6002810460018216806111a857607f821691505b602082108114156111c957634e487b7160e01b600052602260045260246000fd5b50919050565b634e487b7160e01b600052604160045260246000fdfea264697066735822122097aef730e38bcafe3a097fed3f6392140278fcd4cf93a5eab8a30bc8c33834fe64736f6c63430008000033
Checkout more smart contracts
- Verified
- Fungible Token
- ERC20
- Verified

SHILAINU
The following smart contract is the SHILAINU token contract, which is an ERC20 token with a total supply of 1 trillion. It includes features such as transaction limits, fees, and automatic liquidity provision. The contract also has a blacklist mode and the ability to set fee and transaction exemptions for specific addresses. The purpose of the contract is to provide a decentralized currency for the Shiba Inu community.

Token
The following smart contract is a token contract that implements the ERC20 standard. It includes features such as a fee system, wallet and transaction limits, and liquidity provision. The contract also allows for the destruction of tokens through a fee system. The contract is designed to be used with the Uniswap decentralized exchange.
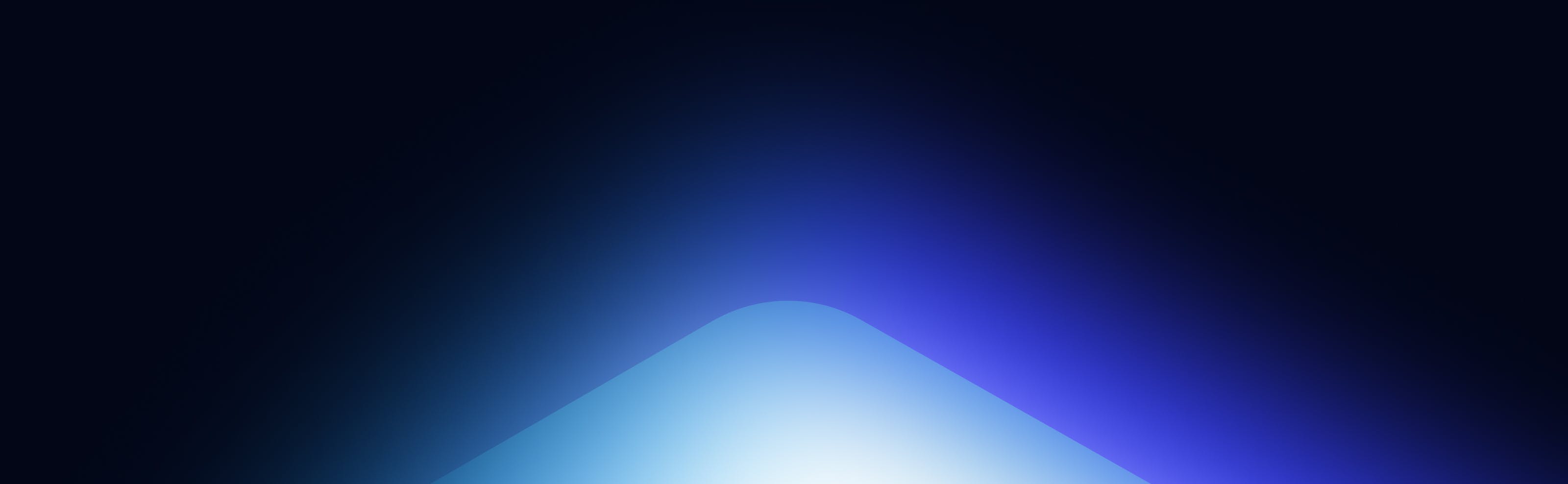